-
What do you want to achieve?
I need to be able to tween a part a certain distance when a global variable is true (and make it go back when false). I got this part done but if I try tweening it (it’s a model) it stops moving and breaks because the other tween moves it past the destination.
-
What is the issue?
It stops moving (the tween inside) because it’s gone past and/or (I don’t know) the position it needed to go. I’ll send a script and images as soon as I can
-
*What solutions have you tried so far?
I’ve tried something’s but they didn’t work, also I couldn’t find anything on dev forum
To sum how it works is when it receives a true value of a certain global variable it moves the distance from 1 part to 2 part (in the same group). That doesn’t mean that it moves on the exact location of 1 and 2 but 2 pos - 1 pos + the moving part pos
Please ask any questions and I will try to get some images, scripts, and videos when I have time
from what i understand is you’re setting the tween on the entire model but different parts move at higher speeds?
you could weld the model or apply the tween on the primary part
The model gets tweened and some of the part(s)/models(s) inside also get tweened
This video should help:
Script (I have a different one for the moving model but this is the best to go off)
local TweenService = game:GetService("TweenService")
local Type1 = script.Parent.Parent.Type1
local Type2 = script.Parent.Parent.Type2
local Type4 = script.Parent.Parent.Type4
local part = script.Parent
local onForward = true
local onBackward = false
local distanceFromType1 = part.Position - Type1.Position
local speed = 1
-- Finding Distances for Types
local function getDistanceToTarget(targetPosition)
local currentX, currentY, currentZ = part.Position.X, part.Position.Y, part.Position.Z
local targetX, targetY, targetZ = targetPosition.X, targetPosition.Y, targetPosition.Z
return math.sqrt(
(currentX - targetX) ^ 2 + (currentY - targetY) ^ 2 + (currentZ - targetZ) ^ 2
)
end
-- Tweening part
while true do
if _G[Type4.BrickColor.Name] == true and onForward == true then
local distanceToType2 = getDistanceToTarget(Type2.Position)
local goal = {Position = Vector3.new(part.Position.X + Type2.Position.X - part.Position.X + distanceFromType1.X, part.Position.Y + Type2.Position.Y - part.Position.Y + distanceFromType1.Y, part.Position.Z + Type2.Position.Z - part.Position.Z + distanceFromType1.Z)}
-- Adjust time based on distance (optional)
local tweenInfo = TweenInfo.new(speed, -- Adjust time based on distance
Enum.EasingStyle.Linear,
Enum.EasingDirection.InOut,
0, -- RepeatCount (0 = no repeat)
false, -- Reverses (tween does not reverse)
0 -- DelayTime
)
local tween = TweenService:Create(part, tweenInfo, goal)
tween:Play()
onBackward = true
onForward = false
elseif _G[Type4.BrickColor.Name] == false and onBackward == true then
local distanceToType1 = getDistanceToTarget(Type1.Position)
local goal = {Position = Vector3.new(part.Position.X + Type1.Position.X - part.Position.X + distanceFromType1.X, part.Position.Y + Type1.Position.Y - part.Position.Y + distanceFromType1.Y, part.Position.Z + Type1.Position.Z - part.Position.Z + distanceFromType1.Z)}
-- Adjust time based on distance (optional)
local tweenInfo = TweenInfo.new(speed, -- Adjust time based on distance
Enum.EasingStyle.Linear,
Enum.EasingDirection.InOut,
0, -- RepeatCount (0 = no repeat)
false, -- Reverses (tween does not reverse)
0 -- DelayTime
)
local tween = TweenService:Create(part, tweenInfo, goal)
tween:Play()
onForward = true
onBackward = false
end
wait(.1)
end
Also I think I want to be able to pause it (instead of stopping it so it keeps the speed)
the issue is that you’re not waiting for tween completion as well u could do something like this
local TweenService = game:GetService("TweenService")
local Type1 = script.Parent.Parent.Type1
local Type2 = script.Parent.Parent.Type2
local Type4 = script.Parent.Parent.Type4
local part = script.Parent
local onForward = true
local onBackward = false
local distanceFromType1 = part.Position - Type1.Position
local speed = 1
local isPaused = false
local currentTween = nil
local function getDistanceToTarget(targetPosition)
local currentX, currentY, currentZ = part.Position.X, part.Position.Y, part.Position.Z
local targetX, targetY, targetZ = targetPosition.X, targetPosition.Y, targetPosition.Z
return math.sqrt((currentX - targetX) ^ 2 + (currentY - targetY) ^ 2 + (currentZ - targetZ) ^ 2)
end
local function tweenPart(targetPosition)
local goal = {Position = targetPosition + distanceFromType1}
local tweenInfo = TweenInfo.new(speed, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
local tween = TweenService:Create(part, tweenInfo, goal)
return tween
end
local function pauseTween()
if currentTween and currentTween.PlaybackState == Enum.PlaybackState.Playing then
isPaused = true
currentTween:Pause()
end
end
local function resumeTween()
if currentTween and isPaused then
isPaused = false
currentTween:Play()
end
end
while true do
if not isPaused then
if _G[Type4.BrickColor.Name] == true and onForward then
if currentTween then currentTween:Cancel() end
currentTween = tweenPart(Type2.Position)
currentTween:Play()
currentTween.Completed:Wait()
onBackward = true
onForward = false
elseif _G[Type4.BrickColor.Name] == false and onBackward then
if currentTween then currentTween:Cancel() end
currentTween = tweenPart(Type1.Position)
currentTween:Play()
currentTween.Completed:Wait()
onForward = true
onBackward = false
end
end
task.wait(0.1)
end
no, it’s supposed be able to go back anytime, not only once it’s been completed. So if it half way and the global value is now false it will go back to the starting position
My problem is that the red part (can be any color) doesn’t move the right amount when both the colors is activated because it tweens forward, but since the other one is moving past the set distances, it doesn’t work
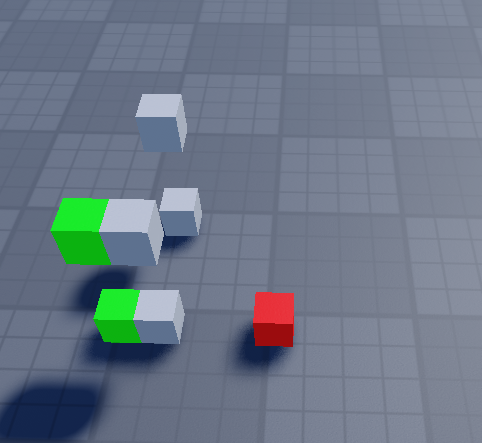
i made tween part takes both start and targetr positions as well with loop checks could you try it and let me know if there’s any issue
local TweenService = game:GetService("TweenService")
local Type1 = script.Parent.Parent.Type1
local Type2 = script.Parent.Parent.Type2
local Type4 = script.Parent.Parent.Type4
local part = script.Parent
local onForward = true
local onBackward = false
local distanceFromType1 = part.Position - Type1.Position
local speed = 1
local isPaused = false
local currentTween = nil
local function getDistanceToTarget(targetPosition)
local currentX, currentY, currentZ = part.Position.X, part.Position.Y, part.Position.Z
local targetX, targetY, targetZ = targetPosition.X, targetPosition.Y, targetPosition.Z
return math.sqrt((currentX - targetX) ^ 2 + (currentY - targetY) ^ 2 + (currentZ - targetZ) ^ 2)
end
local function tweenPart(startPosition, targetPosition)
if currentTween then
currentTween:Cancel()
end
local distance = (targetPosition - startPosition).Magnitude
local duration = distance / speed
local goal = {Position = targetPosition}
local tweenInfo = TweenInfo.new(duration, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
currentTween = TweenService:Create(part, tweenInfo, goal)
return currentTween
end
local function pauseTween()
if currentTween and currentTween.PlaybackState == Enum.PlaybackState.Playing then
isPaused = true
currentTween:Pause()
end
end
local function resumeTween()
if currentTween and isPaused then
isPaused = false
currentTween:Play()
end
end
while true do
if not isPaused then
local globalValue = _G[Type4.BrickColor.Name]
local currentPosition = part.Position
local targetPosition
if globalValue and onBackward then
targetPosition = Type2.Position + distanceFromType1
onForward = true
onBackward = false
elseif not globalValue and onForward then
targetPosition = Type1.Position + distanceFromType1
onForward = false
onBackward = true
end
if targetPosition and currentPosition ~= targetPosition then
currentTween = tweenPart(currentPosition, targetPosition)
currentTween:Play()
end
end
task.wait(0.1)
end
For some reason the red part doesn’t move (I welded it to the type4, which is green). Anyway I can fix this?
(The higher one moves the type4 which is welded to all of the other part in the lower one and the lower one moves (supposed to) the red part to distance (Calculations in script))
Maybe I could move all of the parts instead of only moving 1 and having everything welded to it
Hey, I need to tween all of the decedents so it’s not working. (I need to move all of them but if the other part that moves is welded to something else it wont tween)