So I have been recently working on a flying boss, and since then I wanted to add a attack such as a homing missile that could be damaged by the player in order to destroy it. However, when starting to make the basics such as making it home in. I have not found a good way to do it and I have done some searching and it seems that RocketPropulsion
is depracted after checking on the documentation.
So I decided to use LineForce
, so far I have just been trying to figure out how to get my Rocket to fly at the NPC as a test. So far the missile has flung around and has done the complete opposite of reaching the NPC. Any ideas?
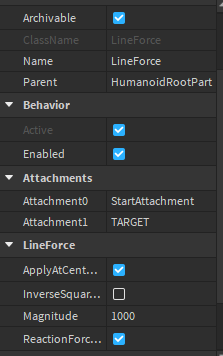
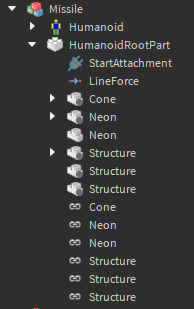
2 Likes
I recommend using AlignPosition and AlignOrientation, and set the missile as massless and uncollideable. If you have any questions, you can ask me
Wow! It works perfectly! How would I make it slower, giving the player more time to react. And also a way to face it towards the player so its a more aiming type of missile.
Glad it was useful. To make it slower, set the responsiveness property to a lower value or you can have an invisible part that can be followed by the missile to the player.
To make it face towards the player, you can simply do like this:
AlignOrientation.CFrame = CFrame.new(ORIGIN_POSITION, POSITION_TO_STARE)
I’ve now done the responsiveness, I will let you know how the testing goes!
Ok. Also, here’s some examples from my code in one of my games:
Example#1
local attachment0 = Instance.new("Attachment", hrp)
local alignOrientation = Instance.new("AlignOrientation")
alignOrientation.Enabled = false
alignOrientation.Attachment0 = attachment0
alignOrientation.Responsiveness = 14
alignOrientation.Mode = Enum.OrientationAlignmentMode.OneAttachment
alignOrientation.MaxTorque = math.huge
Example#2
local alignPosition = Instance.new("AlignPosition")
alignPosition.Enabled = false
alignPosition.Attachment0 = attachment0
alignPosition.Responsiveness = 20
alignPosition.Mode = Enum.PositionAlignmentMode.OneAttachment
alignPosition.MaxForce = math.huge
alignPosition.Parent = hrp
My main idea is to use 2 attachments in the game, and have 1 be in the players primarypart known as the targetattachment. Currently my main idea is to clone the missile and then set the things there.
local function FireRocket(target, rocket_health)
local Missile = BossAssets:WaitForChild("InfernalMissile"):Clone()
Missile.Parent = Effects
Missile:MoveTo(Infernal.PrimaryPart.Position)
Missile.Humanoid.MaxHealth = rocket_health
Missile.Humanoid.Health = rocket_health
local MissilePrimary = Missile.HumanoidRootPart
MissilePrimary.AlignPosition.Attachment1 = target.HumanoidRootPart.TargetAttachment
end
For the health, you can simply use Instane:SetAttribute(Property, Value) or use a IntValue.
And if you want to have control over all the missiles in the game, you can use the CollectionService, like this:
CollectionService:AddTag(MISSILE_INSTANCE, "missile")
heres more about it: CollectionService | Roblox Creator Documentation
and with this, you can have a seperate script to delete missiles that don’t have health
The missile is quite buggy for me since it is very fast and doesn’t want to get damaged, I tried using a humanoid but that didn’t seem to work.
CORRECTION: They do work, they just seem to be under the player most of the time, any ideas for this?
For the buggy missile, try Rocket.PrimaryPart:SetNetworkOwner(nil). (Make sure you run this line after you put it in the workspace, or else it will throw an error.)
And I am no expert on Humanoid, and a fairy long code may be needed to set a linear speed, which I am no sure. I will tell you once I have ideas
Wait a minute, what if you set it to high responsiveness, and modify MaxVelocity of the AlignPosition. I got it from AlignPosition | Roblox Creator Documentation
Edit: also try setting off/on RigidityEnabled
I have to go to sleep now, but I will catch up on this!
k. Have a good sleep, bye bye
[characterlimit]
I’ve sort of fixed the rockets, all I need to do is make them go a bit above the player so they are not below.
Hello back…
I can not visualize what you mean by rocket going below, maybe send me a video
But I am guessing this:
Presuming target.HumanoidRootPart.TargetAttachment is not used by other scripts or by roblox
You could make it go a little above by setting its CFrame
target.HumanoidRootPart.TargetAttachment.CFrame = target.HumanoidRootPart.TargetAttachment.CFrame + Vector3.new(0, 10, 0)
-- x, y, z
This will make the Attachment go up relative to HumanoidRootPart
That could work, After testing, I think creating a new part is the best method as setting the target will completely ruin the players physics in general. Any idea on the targeting system since a new part constantly tracking the player would be difficult.
maybe try this:
local runService = game:GetService("RunService")
local part = Instance.new("Part", workspace)
part.CanCollide = false
part.Anchored = true
local connection
connection = runService.Stepped:Connect(function(time, step)
part.CFrame = target.HumanoidRootPart.CFrame
end)
Once the rocket is done, make sure to disconnect by connection:Disconnect()
and deleting the part.
Problem, it spits out multiple rockets. However I have realised that the Player automatically has a Attachment inside their HRP called “RootJoint” so there is no need for the target attachment.
ok, I see. Not sure how I can help you, because I don’t know what your code looks like 
Heres the function, if you need more then let me know:
local function ExplodeRocket(Rocket, RocketPrimary)
local Explosion = Instance.new("Explosion", RocketPrimary)
Explosion.BlastRadius = 0
Rocket.Humanoid.Health = 0
task.wait(1)
Rocket:Destroy()
local Sound = Instance.new("Sound", Effects)
Sound.SoundId = explosiveSounds[math.random(1, #explosiveSounds)]
Sound:Play()
Debris:AddItem(Sound, Sound.TimeLength)
end
local function FireRocket(target, rocket_health)
local Missile = BossAssets:WaitForChild("InfernalMissile"):Clone()
local MissilePrimary = Missile.HumanoidRootPart
local MissileTarget = Instance.new("Part", workspace.bin)
MissileTarget.Name = "MissileTarget"
MissileTarget.Size = Vector3.new(1,1,1)
MissileTarget.CanCollide = false
MissileTarget.Transparency = 1
MissileTarget.Anchored = true
local AttachmentTarget = Instance.new("Attachment", MissileTarget)
AttachmentTarget.Name = "TargetAttach"
AttachmentTarget.Orientation = Vector3.new(-90, -180, 0)
MissilePrimary.AlignPosition.Attachment1 = AttachmentTarget
MissilePrimary.AlignPosition.Attachment1 = AttachmentTarget
repeat task.wait(0.1)
MissileTarget.Position = target.HumanoidRootPart.Position
until Missile.Humanoid.Died
Missile.Parent = Effects
Missile.HumanoidRootPart:SetNetworkOwner(nil)
Missile:MoveTo(Infernal.PrimaryPart.Position + Vector3.new(0, 10, 0))
Debris:AddItem(Missile, 250)
Missile.Humanoid.MaxHealth = rocket_health
Missile.Humanoid.Health = rocket_health
Missile.Humanoid.Died:Connect(function()
MissileTarget:Destroy()
ExplodeRocket(Missile, MissilePrimary)
end)
MissilePrimary.Touched:Connect(function(Hit)
if not InvulnerabilityFrame then
if Hit ~= nil then
if Players:GetPlayerFromCharacter(Hit.Parent) then
InvulnerabilityFrame = true
ExplodeRocket(Missile, MissilePrimary)
local Player = Players:GetPlayerFromCharacter(Hit.Parent)
local TargetHumanoid = Hit.Parent:WaitForChild("Humanoid")
TargetHumanoid:TakeDamage(MissileDamage)
FX_Remotes.Camera.SmallBump:FireAllClients()
task.wait(1)
InvulnerabilityFrame = false
end
end
end
end)
end