How can I make a joystick GUI that controls a part.
I don’t know what to do or where to start.
This is what it look like:
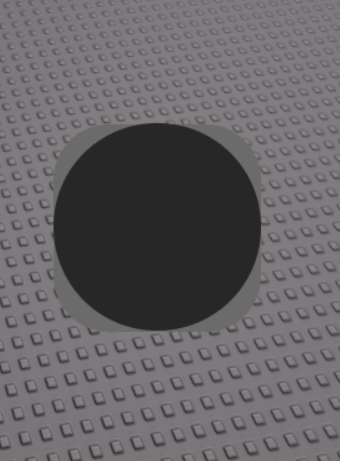
I looked for tutorials and resources on this but all I found was hot to make a custom joystick for mobile players
I would like to know how to drag it around only in a specific area.
and know what direction its being dragged towards.
Basically, you get when the user started the input and then when the user ended the input. Using math, you can determine which direction they intend to move your joystick.
-- In order to use the InputBegan event, the UserInputService service must be used
local UserInputService = game:GetService("UserInputService")
-- A sample function providing multiple usage cases for various types of user input
UserInputService.InputBegan:Connect(function(input, gameProcessed)
if input.UserInputType == Enum.UserInputType.Keyboard then
print("A key is being pushed down! Key:", input.KeyCode)
elseif input.UserInputType == Enum.UserInputType.MouseButton1 then
print("The left mouse button has been pressed down at", input.Position)
elseif input.UserInputType == Enum.UserInputType.MouseButton2 then
print("The right mouse button has been pressed down at", input.Position)
elseif input.UserInputType == Enum.UserInputType.Touch then
print("A touchscreen input has started at", input.Position)
elseif input.UserInputType == Enum.UserInputType.Gamepad1 then
print("A button is being pressed on a gamepad! Button:", input.KeyCode)
end
if gameProcessed then
print("The game engine internally observed this input!")
else
print("The game engine did not internally observe this input!")
end
end)
-- In order to use the InputChanged event, the UserInputService service must be used
local UserInputService = game:GetService("UserInputService")
-- A sample function providing multiple usage cases for various types of user input
UserInputService.InputEnded:Connect(function(input, gameProcessed)
if input.UserInputType == Enum.UserInputType.Keyboard then
print("A key has been released! Key:", input.KeyCode)
elseif input.UserInputType == Enum.UserInputType.MouseButton1 then
print("The left mouse button has been released at", input.Position)
elseif input.UserInputType == Enum.UserInputType.MouseButton2 then
print("The right mouse button has been released at", input.Position)
elseif input.UserInputType == Enum.UserInputType.Touch then
print("A touchscreen input has been released at", input.Position)
elseif input.UserInputType == Enum.UserInputType.Gamepad1 then
print("A button has been released on a gamepad! Button:", input.KeyCode)
end
if gameProcessed then
print("The game engine internally observed this input!")
else
print("The game engine did not internally observe this input!")
end
end)
Reference:
4 Likes