Saving chunks should be easy enough, just go through the world N studs at a time, stopping at (0+k1N, 0+k2N, 0+k3N) for k1, k2, k3 being integers and saving them into the array at location [k1][k2][k3]. (Might sound a bit complicated but this part really isn’t, I’m just kinda sleepy and can’t think of a better way to explain it lol)
As for getting the chunks which intersect with the thing that you cut up, heres a diagram:
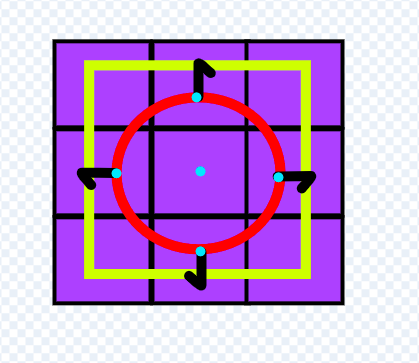
Each purple square (or cube, since its supposed to be 3D) is a chunk.
The red circle marks where you’ve cut,
With the 5 (techinically 6, but since its a topdown view, the middle one represents 2) blue dots representing the points you’re supposed to find.
The black arrows are the rounding you have to do to the points you found (depending on which side of the center point its at, you have to either round it up or down so that it would become kN for k being a integer)
After doing the rounding, you would end up with the yellow square (cube), which is a box made up of lines that pass through the most outer layer of the group of chunks you want. Using the value of N and the location of the vertices of the cube, you can then find all the other chunks.
(So for example, our yellow square {ill use square here since its simpler} has vertices 0,0 0,5 5,5 5,0 and N = 1. We can then get that all the coordinates between 0,0 and 0,5 are the ones we want as long as they follow the rule that it is 0,0+kN for any integer k where kN<=5. We then also know that all of the columns between 0,0 and 5,0 are ones we want, where we can get all chunks in each row by doing the previous operation we did with 0,0 and 0,5, and then by incrementing the X value by 1 until it also reaches the ending point of 5. We thus get that the chunks we want are the following 2D array:
[[0, 0], [0, 1] … [0, 5]
[1, 0], [1, 1] … [1, 5]
…
[5, 0]. [5, 1] … [5, 5]]
We can then get all the chunk data by getting the reference we want from the original array.
As we only used the k value and not the kN value in the orignal array, we have to divide each X and Y (and Z, since your code should be 3D) value by N. So for example, to get the stored information for the chunk at [0, 0], we should reference Chunks[0/N][0/N], which gets us Chunks[0][0])
I believe I did a pretty bad job at explaining this, but hopefully its just well enough that you could understand. If not, it might be worth the time to search up some sort of tutorial about storing chunks of the world into arrays.
Theres also a hacky way of finding the intersection:
Place an invisible, cancollide false part on each chunk, and make sure that it is the exact size of that chunk (maybe slightly smaller in case of errors). Then, when you remove terrain, create a sphere the exact size of the removed terrain, and call getPartsInPart on that sphere to get all of the parts which intersect the sphere. This would give you all the parts which correspond to the chunks that your destruction intersects, and you can then simply use the X, Y, and Z value of the parts to get the saved information from the list.
Do note that this method may be laggy if you have larger maps.