I’ll rephrase it and put some code examples/pictures to hopefully make it easier for you (and possibly others) to understand.
Imagine you have 5 players in your game and you only want one of them to be selected for something rather if it’s VIP, Leader, or whatever. One of the most simplistic ways of choosing someone out of that list is to use math.random from the player list, here’s an example.
local ListOfPlayers = game.Players:GetPlayers()
local ChosenPlayerPos = math.random(1, #ListOfPlayers)
local ChosenPlayer = ListOfPlayers[ChosenPlayerPos]
In the first line I gather the list of players that I know are participating to be chosen. Using :GetPlayers() returns a list of players that are connected into your game which looks like this:
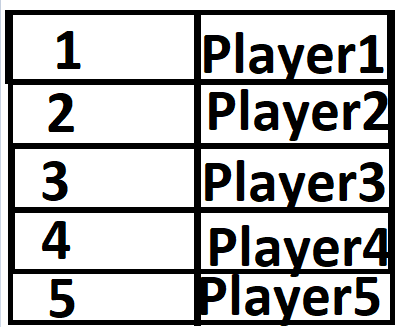
Now in the second part I picked a position which I simply used math.random. When you use math.random using interger numbers for the first and second part (like math.random(1, 3)) it will return a random generated number between the first number and last number. By doing math.random(1, #ListOfPlayers) I am basically doing math.random(1, 5) which I am getting a random number between 1 and 5.
Now that I got my chosen random number I basically go back into the table and grab the player using my random number and retrieve the player that has been chosen. So if my random chosen number was number 3 this would return me Player 3.
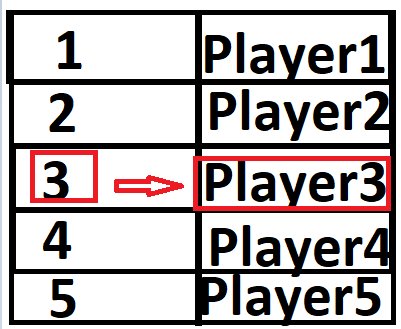
This is a simple script that’s fine to use to pick a player with them all being completely equal percentage wise and all of them having no advantage over the other. In this scenario all players would have a 20% chance of being chosen because everyone would have a 1 / 5 chance of being chosen. If there was 10 players all players would have a 10% chance of being chosen because you would only have a 1 / 10 chance of being chosen.
Now if you want to give players an opportunity to purchase a dev product to increase their chance of being chosen for something, it’s necessary to use something not as simple as math.random. I suggest using a point value system. There are other ways to achieve this but I like this system and I hope you do too lol.
Imagine in the same scenario you have 5 players in your game that want to be chosen and now let’s say each player has 5 points. In this scenario you’re going to need to setup and do two tables for this. So here’s a scripting example
local TotalPlayerPoints = 0
local DefaultPlayerCount = 5
local ListOfPlayerPoints = {}
local ListOfPlayers = game.Players:GetPlayers()
for PlayerNum = 1, #ListOfPlayers do
local Player = ListOfPlayers[PlayerNum]
local CurrentPlayerPoints = DefaultPlayerCount
table.insert(ListOfPlayerPoints, PlayerNum, CurrentPlayerPoints)
TotalPlayerPoints = TotalPlayerPoints+CurrentPlayerPoints
end
Right now we’re setting up a list of participants into a point list which will look something like this.
If for example your dev product increased the chance points by +5, you would add CurrentPlayerPoints = DefaultPlayerCount+Dev(+5)
to the script.
Now to get into the player choosing part you can use math.random but you’ll have to iterate your new participants of player points. We have the Total Points so now we just pick a random number and then we loop through all of the points to make sure one of the players are are in that range. So for scripting example
local WinnerPlayerPoint = TotalPlayerPoints*math.random() --25 total if 5 players
local MinPointRoll = 0
local ChosenPlayerPos = 0 --Not chosen yet
for PlayerPointNum = 1, #ListOfPlayerPoints do
local MaxPointPoll = MinPointRoll+ListOfPlayerPoints [PlayerPointNum]
if WinnerPlayerPoint >= MinPointRoll and WinnerPlayerPoint <= MaxPointPoll then
ChosenPlayerPos = PlayerPointNum
--We have a winner!
break
else
--We don't have a winner, keep drawing
MinPointRoll = MinPointRoll+MaxPointPoll
end
end
And basically what’s going on in your script is that it’s just going through the points to make sure the chosen number is in the range of the points of your participants with player number 4 being the winner.
Hope this helped!