I’m making a bridge builder game, and i need bridge joints to break if they are under too much load, like Poly Bridge
Bridge = workspace.Bridge
Hinge = Bridge.HingeConstraint
MaxStress = 100
Bridge.Stress.Changed:Connect(function()
if Bridge.Stress.Value > MaxStress then
Hinge.Enabled = false
end
end)
Things that need to affect the hinges are;
- Heavy objects
- Gravity
- Mass
- Stress
- etc
How do i go about creating this?
Iv’e tried using SpringConstraints to do this, as you can get the length of the spring, but the bridges have way to much flex and are extremely glitchy. And hinges make the bridge look much more natural.
1 Like
To detect the total amount of force being applied downwards on Hinge, you can add up the forces of the various items that push downwards. In terms of parts, you can multiply their mass by their velocity downwards.
For example:
Part1 = HingeConstraint.Attachment0.Parent
Part2 = HingeConstraint.Attachment1.Parent
while true do
Stress = 0
TouchingPartsMass = 0
TouchingPartsVelocity = 0
Gravity = workspace.Gravity
--collect the parts that are currently touching the HingeConstraint parts
TouchingParts = Part1:GetTouchingParts()
for _, item in pairs(Part2:GetTouchingParts()) do
table.insert(TouchingParts, item)
end
for number, item in pairs(TouchingParts) do
if item:IsA("Part") or item:IsA("MeshPart") then
TouchingPartsMass = TouchingPartsMass + item:GetMass() + Part1:GetMass() + Part2:GetMass() -- collect those parts mass plus the mass of the HingeConstraint objects
TouchingPartsVelocity = TouchingPartsVelocity + item.Velocity.Y --collect those parts velocity downwards
if TouchingPartsVelocity > 0 then
TouchingPartsVelocity = 0
end
end
end
Stress = Gravity * -1 + TouchingPartsMass * (-1 + TouchingPartsVelocity) --add these values together to get the stress
print(Stress)
if Stress > MaxStress then
HingeConstraint.Enabled = false
end
wait()
end
This is just a concept, and you would have to tinker with how much each force affects the total Stress.
It is also not going to be completely accurate, since there are a few other factors involved, but it can give you an idea.
Hope the above helps!
1 Like
are these suppose to be negative values?
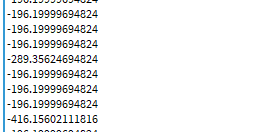
I was thinking in terms of force downward onto the hinge, so yes. Just invert Stress to get it in it’s positive form.
1 Like
So, iv’e done some experimenting with your system, and wow am I impressed. This is by far the best custom roblox physics engine iv’e ever seen! I mean this is just an example, and its good.
And like you said it does need a bit of tweaking, but this will work. Thanks!
And keep up the good work my man!
1 Like
This is by far the best one! but…
stress doesn’t change when there are linked bridges. Stress will only change when there’s weight on it.
Also supports don’t decrease stress.
How should i go about that?
I would add on to the preexisting script to look for other truss’s that are connected, until they hit an anchor point. The more you have, the more stress is added because of weight. For supports you can do the same, but decrease the Stress for all that are connecting
1 Like