Basically I have a folder and inside of the folder are parts with different names, in each part they have a boolvalue, how can I use GetChildren()
(Im trying to get the bool value in each part) if all of the parts have different names?
I got stuck in here:
local Button = script.Parent.Parent
local PP = script.Parent -- proximityprompt
local Blocks = game.Workspace.Blocks
for index, Campfire in pairs(Blocks:GetChildren()) do -- how do I ask for the children inside of "Blocks" if they all have different names?
if Campfire.Boolvalue.Value == true then
-- code here
end
end
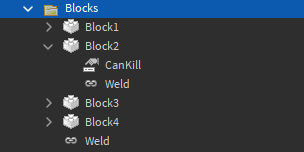
You’re very close. If you’re referring to getting each block with a different name, you’ve already done so.
The problem is on your second line in the key/value pairs:
“Boolvalue” doesn’t exist. It’s called “CanKill.” All you’d have to do is use “CanKill” instead of “Boolvalue”
However, if you meant that each BoolValue would have a different name, all you’d have to do is search via a class search:
if Campfire:FindFirstChildWhichIsA("BoolValue").Value == true then
Keep in mind, this would throw errors if a BoolValue isn’t inside a block, so you could do a check to make sure that it exists.
1 Like
for index, Campfire in pairs(Blocks:GetChildren()) do
local boolvalue = Campfire:FindFirstChildOfClass('BoolValue')
if boolvalue and boolvalue.Value then
-- code here
end
end
Should do what you’re wanting.
for i, v in pairs(workspace.Blocks:GetChildren()) do
if v:IsA("Part") then
for index, value in pairs(v:GetChildren()) do
if value:IsA("BoolValue") then
print(v.Name, value.Value)
end
end
end
end
You’ll need to change the path to the correct folder.