Good evening, i used to script a lot and quit becuase i didnt have a computer at the time.
I got one but long story short i forgot how to make scaleable ui
Please give me the answer or at least something easy to understand
(I remember changing the size to a certain thing and it would scale with the users screen automatically!)
How to do this?
When making my UIs, I typically size and position them via Offset
and rarely use Scale. Due to this, different devices will render that UI either bigger or smaller. So to combat that, I insert a UIScale
object into the ScreenGui and have this code wrapped in a RunService.Heartbeat loop:
local UIScaler = {}
local IsMobile = game:GetService("UserInputService").TouchEnabled
function UIScaler.Update()
if IsMobile then
_G.mainui.UIScale.Scale = _G.localcamera.ViewportSize.X / 1400
else
_G.mainui.UIScale.Scale = _G.localcamera.ViewportSize.X / 1500
end
end
return UIScaler
Copying and pasting this exact code wouldn’t work for you due to how I have my game set up, so just take the concept from the code above.
[Note]: I typically only have one single ‘ScreenGui’ object present in my game, like so:
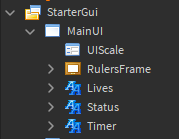
Yes but as i recall, if i just change the size of the gui to a certain thing it makes it scalable automatically without scripts
This would use UDim2, and most likely Vector2. You would also need to use the UserImputService service, which would just be a nightmare. So can you? Yes, but if it isn’t necessary, then don’t do it. (You can just make a script to drag the UI… How to make DRAGGABLE GUIS | Roblox Tutorial - YouTube)
I remember i was just able to type the size a certain way though.
I believe he wants a UI that will scale down as the user’s screen gets smaller lol. Normally you could just use the Scale
part of a UDim2.new(ScaleX, OffsetX, ScaleY, OffsetY), but I’ve always run into issues with sizing the UIObject via Scales.
Ohh! In that case, just make the UI small enough to fit on one screen, and big enough to fit on another.
Ok but i remember i could just change the size to a certain format in properties and it would do it automatically.
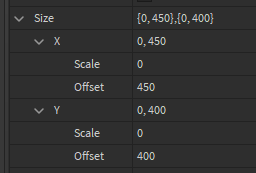
Instead of sizing your UIs with Offset (like I did in this image above), you could make the offset’s 0 and use Scale. Scale will automatically scale the UI to fit the screen by a certain percentage. Scale normally ranges from 0 to 1.
For example: a UI with a size of UDim2.new(0.5, 0, 1, 0) will cover half the screen in the X direction (represented by the 0.5 [50% scale]) and will cover the entire screen in the Y direction (represented by the 1 [100% scale]).
Its not working. The text doesnt change size depending on the screen size
… Then enable the TextScaled property.
Size & position everything using the “Scale” components only of UDim2 values, avoid using the “Offset” components for both the x axis and y axis.