So what I want to do is replace the basic tool idle stance to my character holding the gun. Basically from this:
(Part is just a placeholder I haven’t finished my gun just yet)
To something more like this.
So how would I go by achieving this? So far I’ve thought of playing my animation and then pausing it right before it ended, but there has to be a better way. Right?
local UIS = game:GetService("UserInputService")
local player = game.Players.LocalPlayer
repeat wait(1) until player.Character
local character = player.Character
local humanoid = character:WaitForChild("Humanoid")
local sprintSpeed = 30
local walkSpeed = 16
local crouchSpeed = 10
local equipped = false
local animation = Instance.new("Animation")
animation.Name = "Idle"
animation.Parent = script.Parent
animation.AnimationId = "rbxassetid://8269791536"
local animtrack = humanoid:LoadAnimation(animation)
local runAnim = Instance.new("Animation")
runAnim.Name = "Sprint"
runAnim.Parent = script.Parent
runAnim.AnimationId = "rbxassetid://6932225442"
local runAnimTrack = humanoid:LoadAnimation(runAnim)
local function beginSprint(input, gameProcessed)
if not gameProcessed then
if input.UserInputType == Enum.UserInputType.Keyboard then
local keycode = input.KeyCode
if keycode == Enum.KeyCode.LeftShift and equipped then
runAnimTrack:Play()
end
end
end
end
local function endSprint(input, gameProcessed)
if not gameProcessed then
if input.UserInputType == Enum.UserInputType.Keyboard then
local keycode = input.KeyCode
if keycode == Enum.KeyCode.LeftShift then
runAnimTrack:Stop()
end
end
end
end
script.Parent.Equipped:Connect(function()
if character.Humanoid.WalkSpeed == sprintSpeed then
runAnimTrack:Play()
else
animtrack:Play()
end
equipped = true
end)
script.Parent.Unequipped:Connect(function()
animtrack:Stop()
runAnimTrack:Stop()
equipped = false
end)
UIS.InputBegan:Connect(beginSprint)
UIS.InputEnded:Connect(endSprint)
This will give you a custom idle as well as a custom sprint animation
try
local Player = game.Players.LocalPlayer
local Character = Player.Character or Player.CharacterAdded:Wait()
local Animate = Character:WaitForChild("Animate")
Animate:FindFirstChild("toolnone"):GetChildren()[1].AnimationId = "rbxassetid//0000000000"
I just get da children cuz i forgot the animation name
So do I need to play the animation at all?
no the animate script will play the animation just change the animation id
I got this
I copy and pasted your script directly into this local script
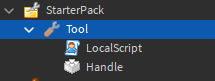
local Player = game.Players.LocalPlayer
local Character = Player.Character or Player.CharacterAdded:Wait()
local Animate = Character:WaitForChild("Animate")
Animate:FindFirstChild("toolnone"):GetChildren()[1].AnimationId = "rbxassetid//8443081390"
Ah I get it now I just replace the ToolNoneAnim ID with the ID of my anim.
Ah, I keep on getting this error From the Animate Script
**Invalid animation id ‘<error: unknown AssetId protocol>’: **
My script:
(I understand it is not the best I just slapped it together quickly for proof of concept)
wait(1)
local player = game.Players.LocalPlayer
local char = player.Character
local toolNoneAnim = char:WaitForChild("Animate").toolnone.ToolNoneAnim
toolNoneAnim.AnimationId = "8443081390"
Did you meant to use:
"rbxassetid://8443081390"
It shouldn’t change anything, I was just setting the AnimationId
Never mind, you were right. Thank you.
Now the animation plays, but the arms still go back down. How do I stop this?
I fixed it, the animation will only stay put if it is one keyframe.
But now the legs won’t move when I walk, but I think it has to do with animation priority.
Nope legs still do not move when walking. But I think this is for another dev forum post. Thanks puofz and Crunch for your help.
Why not just play a custom animation when the tool is equipped, instead of replacing the animation? Works for me.
wait()
local tool = script.Parent
local plr = game.Players.LocalPlayer
local char = plr.Character
local anim = Instance.new("Animation",tool)
anim.AnimationId = "rbxassetid://8443081390"
local animLoaded = char.Humanoid:LoadAnimation(anim)
animLoaded.Priority = Enum.AnimationPriority.Action --This is to prevent the animation from possibly breaking due to other animations
tool.Equipped:Connect(function()
animLoaded:Play()
end)
tool.Unequipped:Connect(function()
animLoaded:Stop()
end)
e tool animaation should be action
The problem being is that the animation just defaults back to the default tool stance.
This may be because you added keyframes for the legs, causing them to stay still.
Remove them and try the animation again.
You can just add the script to the other tools.
Or you can just replace the default toolnone animation, if you want to apply the animation to every tool equipped.