So I am not asking for someone to make a script. How ever I’m curious on the basics, how do you think someone would go about making a script that would fetch a UGC limited they have on sale? So like it would see the UGC Limited’s they are selling, then be able to prompt the buyer for THAT specific one. Any ideas on how I should try and go about this like what services I should try and work with? (Or if it even sounds possible)
So for starters, Of course we can do this!
Edit: I used a Proximity Prompt to give the notification out the the User, and explain my set up. You may not want to do this, rather use a Gui or something. But it should be somewhat similar!
Now I am gonna break this up, so that we can get an understanding, as this has many parts:
-
Get the limiteds that the user is selling
-
Load any info you would want (like if you wanted to make a gui, with the name or the image of the product), as well as making sure it is still being sold
-
Making the Proximity prompt and selling the product - Remember, I am using a Proximity prompt for my system as it is easy to use as an example! You do not need to do this, if you wanted a click detector or Ui set up!
Now I am going to work a bit backwards, as I have Ideas for step 2 & 3, and worry about step 1 later.
Assuming we have the Asset Id already, we can do step 2 & 3 together. Kind of like this:
Test Coding
local Marketplace = game:GetService("MarketplaceService")
local AssetId = 13318621520 --A test asset ID of a limited item
local player = game.Players:FindFirstChild("OfficialPogCat") --An example, of myself. But you would need to change this up to have many players. And Usually you would want to to find the player from the proxy prompt
wait(10)
local GetInfoFromAssetId = Marketplace:GetProductInfo(AssetId)
if GetInfoFromAssetId and GetInfoFromAssetId.IsForSale == true and GetInfoFromAssetId.CanBeSoldInThisGame == true and GetInfoFromAssetId.Remaining > 0 then
Marketplace:PromptPurchase(player, AssetId)
print(GetInfoFromAssetId.Name)
end
This is what I started Out to test this. Now what this script does, is it waits 10 seconds for the player (Me) to load, and then checks if it is Limited, and can be sold in game. Now, we can easily change this into a Proximity prompt if we want, like:
An example Code For a Proximity prompt type system
local Marketplace = game:GetService("MarketplaceService")
local folder = game.Workspace.AllProxyPrompts -- I have a folder that Has all the prompts in. This may be different in your game!
for i, EachProxy in ipairs(folder:GetChildren()) do
if EachProxy:IsA("BasePart") and EachProxy:FindFirstChild("AssetId") and EachProxy:FindFirstChildWhichIsA("ProximityPrompt") then
local AssetId = EachProxy:FindFirstChild("AssetId").Value
local GetInfoFromAssetId = Marketplace:GetProductInfo(AssetId)
if GetInfoFromAssetId and GetInfoFromAssetId.IsForSale == true and GetInfoFromAssetId.CanBeSoldInThisGame == true and GetInfoFromAssetId.Remaining > 0 then
EachProxy:FindFirstChildWhichIsA("ProximityPrompt").ActionText = "Buy "..GetInfoFromAssetId.Name
EachProxy:FindFirstChildWhichIsA("ProximityPrompt").ObjectText = "Cost: "..GetInfoFromAssetId.PriceInRobux
EachProxy:FindFirstChildWhichIsA("ProximityPrompt").Triggered:Connect(function(player)
Marketplace:PromptPurchase(player, EachProxy:FindFirstChild("AssetId").Value)
end)
else
print("Error: Limited cannot be sold! Asset Name: "..GetInfoFromAssetId.Name)
end
end
end
If you want to know more about the set up for the Proximity prompt, here is the set up. Note that if you use my script or something similar, a lot will need to changed depending on the layout and set up of your game:
Setting up the Proximity prompt
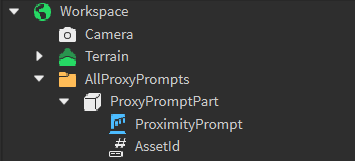
Now that we have step 2 & 3 done, time for the hard part. At least for me. There are many ways to do step 1. So I am gonna talk about 2 main ways you can get the limiteds.
-
You could Have a place where players put the asset id of their limited they want to sell. For me, this is what I would do, and the easiest way. Once they add the Id, you just check if the
Creator.CreatorTargetId
matches theUserId
, and making sure the item is a limited before posting it. Then I would save this ID, in a datastore, just for easy access. -
If we are taking about an automatic system, things get a little too complex for me to explain, but I will try my best. You will need to host a proxy, and do some coding out of roblox to fetch the data, to get the information from roblox limiteds from a users inventory. Note: If a users inventory is private, it just simply won’t work. I would recommend if you are going this route, to use:https://inventory.roblox.com/docs#!/Inventory/get_v2_users_userId_inventory_assetTypeId to get the infromation, and filter the non limiteds out.
Hope this helps you out! And ask questions as needed.
Sweet thanks! I will definitely try this out! What do you think would be best to start my research into making a bot/outside source to make this fully automatic?
Example: So it would fetch the users profile for there inventory Limited’s, check if they are on sale by them, then when someone clicks a button or uses the proximity prompt, it prompts the one they are selling.
Have any ideas on somewhere I should look into 1st?
For starters if your looking for fully automatic systems:
-
I myself don’t use proxy’s as much as I would like too, and even I myself am trying to look for ways to fetch items, like group owned tshirts, and things. However for starting your search, the dev forum is an amazing place for resources. I don’t know how advanced you are in scripting, but I myself find the dev forum helpful, as long as you can understand how other people scripts work and making it your own.
-
I talked about this a bit, but I just want to make sure you understand, as well as developing ways around it. If a player inventory is private, it simply will not work . People’s inventory must be visible to everyone, if you want an inventory api to work. You can use a datastore and store all the asset ids, for easy access, if a player joins with a public inventory, but then hides it.
Here are some good dev forum posts that I found with a little bit bit of searching, about using inventory api:
Dev forum posts
The following are how to set up a proxy:
https://devforum.roblox.com/t/making-a-proxy-for-roblox-using-nodejs/1392622
Some sources about what your trying to do:
[OUTDATED] - But might still be useful, Roblox Inventory API Tutorial
[OUTDATED] Again Ik - But still might help, getting players inventory
When I say “outdated” they sadly are. Any post you find that using roproxy.com, in the url will not work, as the developers have stop supporting it.
These are just a few though, but if you do a search, you can find so many! Here might be a a couple key word searches: “How to make my own proxy roblox”, “How get get items from a players inventory”, “How to access roblox.com through https service”.
- Although setting this up might be a bit complex, I can atleast write it down on paper with what we want to do.
-
We will want to use https://inventory.roblox.com/docs#!/Inventory/get_v2_users_userId_inventory_assetTypeId to fetch every item from a persons inventory.
-
Then filter through each item, With
MarketPlaceService:GetProductInfo(AssetID)
to check ifCreator.CreatorTargetId
matches theUserId
of the player. If it does, we will check if it is a limited item. -
If it makes it through all of that, then we will post it, for other players to see!