Hiwi!
Okie, I made your script, and I want to help you to connect it. Just follow these steps.
BTW, I checked your Teleport script, and its good, but its triggering at least 4 times… And thats not good. (Brief explanation)
The characters are made of many parts, and the Touch event, gets the Touch Event PER part inside the character. Everytime a part is found your function is cheking the part’s parent to find a Humanoid… And it will find a Humanoid maybe +16 times… Triggering “something” +16 times… Which you and nobody wants to that happen…
So I changed it completely. I dont know the “best” approach. I just use this approach cause I like it. I only compare if the Hit part.Name its a HumanoidRootPart. We have only 1 HumanoidRootPart inside the characters, so its convinient. Triggers only once.
1- The only one inconvinient, is that you gotta make a “tall” invisible boundary box to check the Touch event, heres some pics: (make it enough tall to hit the full body, and you can make it totally invisible, and keep a small part or something you want as a “Teleport model”)
2- You need to create a RemoteEvent and place it inside ReplicatedStorage, name that RemoteEvent “LightEvent”
And you need to place at least the SunRays inside lighting.
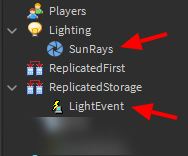
3- Place this Script inside the BoundaryBox:
-- Services
-- Parent ReplicatedStorage
local RepS = game:GetService("ReplicatedStorage")
local Players = game:GetService("Players")
-- RemoteEvents
local LE = RepS:WaitForChild("LightEvent")
-- Coordinates to TP
local place = CFrame.new(-719.318, 525.448, 340.759)
-- On touch a boundary invisible box. Should be tall enough to be touched by the HumanoidRootPart
script.Parent.Touched:connect(function(hit)
if hit.Name == "HumanoidRootPart" then -- Make sure the function trigger ONLY ONCE, only when the HumanoidRootPart is found
local hitPlayer = Players:GetPlayerFromCharacter(hit.Parent) -- Get the Player instance from HRP touched
hitPlayer.Character.HumanoidRootPart.CFrame = place -- Moving the HumanoidRootPart
LE:FireClient(hitPlayer) -- Fire Client Event for only the player who touched the box
end
end)
4- Place this LocalScript inside StarterPlayerScripts
-- Services
-- Parent ReplicatedStorage
local RepS = game:GetService("ReplicatedStorage")
local LE = RepS:WaitForChild("LightEvent")
-- RemoteEvents
local LE = RepS:WaitForChild("LightEvent")
-- Lighting Main Parent
local sun = game.Lighting
-- Parenting the Atmosphere
local sunRays = sun.SunRays
-- Initial SunRays Values for the player when joins
-- Change them to anything you want
sunRays.Intensity = 1
sunRays.Spread = 0.6
-- Function, it will change the Intensity and Spread of SunRays
local function mainLight()
sunRays.Intensity = 0 -- Change this values to anything u want
sunRays.Spread = 0 -- Change this values to anything u want
end
-- Listener, when Server calls the LightEvent it will fire the function above
LE.OnClientEvent:Connect(mainLight)
5- Change values inside the LocalScript to the ones you want for SunRays.
Theres a part in the LocalScript called – Initial SunRays values. Those are the initial values the player gets when join the server. And change the sunRays.Intensity and Spread values inside the function mainLight()
to the ones you want after the player teleports.
Just change the number to the values you want. from 0 to 1. I just used total 0 after the player Teleports. Customize it as you wants.
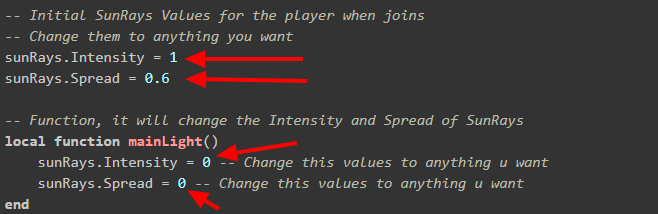
If you did everything right, thats it! If you need to connect more parameters to change, feel free to ask, I can show you how u can change any. Good luck, cya! :3