Finally finished the script
, and here it is:
local frame = script.Parent
local addOffset = 0.001
local floors = {
{
Color = Color3.fromRGB(255,0,0),
Size = 30
},
{
Color = Color3.fromRGB(85, 255, 0),
Size = 25
},
{
Color = Color3.fromRGB(0, 85, 255),
Size = 10
},
{
Color = Color3.fromRGB(255, 170, 255),
Size = 40
}
}
local function calculatePercentage(number,full)
return (number/full)
end
local floorPercentages = {}
local totalFloorSize = 0
for i,v in ipairs(floors) do
totalFloorSize += v.Size
end
--yeah i know it might be inefficient to run through the table twice
for i,v in ipairs(floors) do
floorPercentages[i] = {Color = v.Color, Size = calculatePercentage(v.Size, totalFloorSize)}
end
local gradientStepOne = {
{Color = Color3.new(0,0,0),Time=0}
}
local previousSize = 0
for i,v in ipairs(floorPercentages) do
gradientStepOne[#gradientStepOne+1] = {Color = floorPercentages[i].Color, Time = previousSize+addOffset}
gradientStepOne[#gradientStepOne+1] = {Color = v.Color, Time = previousSize+v.Size}
previousSize += v.Size
end
local function TableToColorSequence(t)
local construct = {}
for i, info in ipairs(t) do
construct[i] = ColorSequenceKeypoint.new(info.Time, info.Color)
print(i, info.Time, info.Color)
end
return ColorSequence.new(construct)
end
local finishedGradient = TableToColorSequence(gradientStepOne)
script.Parent.UIGradient.Color = finishedGradient
This code could probably be more efficient, but that isn’t the point. So, when you modify this script to add/remove levels, all you need to change is the floors
table.
Explanation
Explanation:
I’ll go through each part of the script one by one and explain how it works.
First, the two variables at the top define the frame that the UIGradient is applying to and the offset between levels to make the change more abrupt. The layout I made in the explorer looks like this:
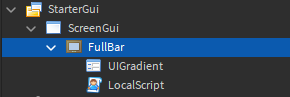
“FullBar” is the frame being referenced to in the script.
Secondly, is the floors
table. It simply uses a similar format to the one you made, with Color and Size being defined within each smaller dictionary. This is what you change to modify the stages/levels and such.
Next, the calculatePercentage()
function. This function simply just takes the size of one level, divides it by the size of all of the levels combined, and creates a size value useable by the UIGradient
.
Afterwards, the floorPercentages
table is defined, and shortly after is filled with values from the floors
table, but in the percentage form I mentioned before.
Then, the gradientStepOne
table is defined. This table is the final table being used before it is converted to a ColorSequence
. It starts defined with
{Color = Color3.new(0,0,0),Time=0}
which is a part to prevent blending of the first stage’s color with the black. Then the table is filled out with values that are doubled up to prevent blending, as shown in this for
loop:
local previousSize = 0
for i,v in ipairs(floorPercentages) do
gradientStepOne[#gradientStepOne+1] = {Color = floorPercentages[i].Color, Time = previousSize+addOffset}
gradientStepOne[#gradientStepOne+1] = {Color = v.Color, Time = previousSize+v.Size}
previousSize += v.Size
end
(ignore the gray text, I’m pretty sure it’s forum formatting for comments in some other coding language)
After the table is filled, it is converted to a color sequence by this function, actually using the code from this forum topic:
local function TableToColorSequence(t)
local construct = {}
for i, info in ipairs(t) do
construct[i] = ColorSequenceKeypoint.new(info.Time, info.Color)
print(i, info.Time, info.Color)
end
return ColorSequence.new(construct)
end
At this point, all you have to do is set the Color
value of the UIGradient
, as done here:
local finishedGradient = TableToColorSequence(gradientStepOne)
It ends up looking like this:
Have a nice day/night 