I made a small JavaScript snippet you can run in your browsers dev console (typically press F12):
let row = 2 // Change this to the row of the color
let offset = 4 // Offset of the color from the left
row = row - 1
let pos = 0
for(let i = 1; i <= row; i++) {
if (i >= 7) {
pos += 13-(i-7)
} else {
pos += 7+i-1
}
}
console.log(pos + offset)
For example, Ghost Grey is row 6 offset 5:
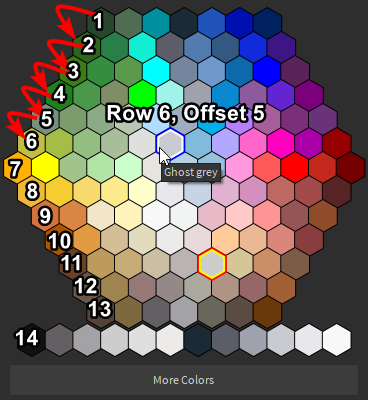
Offset is the number of times you moved right to get to the color. Rows over 14 or under 1 wont work. Make sure to count the offset right or it’ll be wrong
I can convert this to lua if you want a script.
EDIT: I made it a script anyways…
function getPalettePosition(row, offset)
row = row - 1
local pos = 0
for i = 1,row,1 do
if i >= 7 then
pos += 13-(i-7)
else
pos += 7+i-1
end
end
return pos + offset
end
NOTE: The last row (row 14) is literally just Really Black
, there are no other offsets. They’re just showing colors that were already in the palette before, so they don’t have another index.
If you really want to get it by name and not row/offset, you can just loop over all of them, but it’s pretty inefficient, especially if it’s closer to the end, but if you want that anyways, the range is [0, 127]
Edit 2: So I’m really bored, this script gets the index based on name, though I cant say I recommend actually using it
function getIndexByName(name)
for i=0,127,1 do
if tostring(BrickColor.palette(i)) == name then
return i
end
end
return -1
end
What I could recommend is a lookup table instead:
local colorLookup = {
["Earth green"] = 0,
["Slime green"] = 1,
["Bright bluish green"] = 2,
["Black"] = 3,
["Deep blue"] = 4,
["Dark blue"] = 5,
["Navy blue"] = 6,
["Parsley green"] = 7,
["Dark green"] = 8,
["Teal"] = 9,
["Smoky grey"] = 10,
["Steel blue"] = 11,
["Storm blue"] = 12,
["Lapis"] = 13,
["Dark indigo"] = 14,
["Camo"] = 15,
["Sea green"] = 16,
["Shamrock"] = 17,
["Toothpaste"] = 18,
["Sand blue"] = 19,
["Medium blue"] = 20,
["Bright blue"] = 21,
["Really blue"] = 22,
["Mulberry"] = 23,
["Forest green"] = 24,
["Bright green"] = 25,
["Grime"] = 26,
["Lime green"] = 27,
["Pastel blue-green"] = 28,
["Fossil"] = 29,
["Electric blue"] = 30,
["Lavender"] = 31,
["Royal purple"] = 32,
["Eggplant"] = 33,
["Sand green"] = 34,
["Moss"] = 35,
["Artichoke"] = 36,
["Sage green"] = 37,
["Pastel light blue"] = 38,
["Cadet blue"] = 39,
["Cyan"] = 40,
["Alder"] = 41,
["Lilac"] = 42,
["Plum"] = 43,
["Bright violet"] = 44,
["Olive"] = 45,
["Br. yellowish green"] = 46,
["Olivine"] = 47,
["Laurel green"] = 48,
["Quill grey"] = 49,
["Ghost grey"] = 50,
["Pastel Blue"] = 51,
["Pastel violet"] = 52,
["Pink"] = 53,
["Hot pink"] = 54,
["Magenta"] = 55,
["Crimson"] = 56,
["Deep orange"] = 57,
["New Yeller"] = 58,
["Medium green"] = 59,
["Mint"] = 60,
["Pastel green"] = 61,
["Light stone grey"] = 62,
["Light blue"] = 63,
["Baby blue"] = 64,
["Carnation pink"] = 65,
["Persimmon"] = 66,
["Really red"] = 67,
["Bright red"] = 68,
["Maroon"] = 69,
["Gold"] = 70,
["Bright yellow"] = 71,
["Daisy orange"] = 72,
["Cool yellow"] = 73,
["Pastel yellow"] = 74,
["Pearl"] = 75,
["Fog"] = 76,
["Mauve"] = 77,
["Sunrise"] = 78,
["Terra Cotta"] = 79,
["Dusty Rose"] = 80,
["Cocoa"] = 81,
["Neon orange"] = 82,
["Bright orange"] = 83,
["Wheat"] = 84,
["Buttermilk"] = 85,
["Institutional white"] = 86,
["White"] = 87,
["Light reddish violet"] = 88,
["Pastel orange"] = 89,
["Salmon"] = 90,
["Tawny"] = 91,
["Rust"] = 92,
["CGA brown"] = 93,
["Br. yellowish orange"] = 94,
["Cashmere"] = 95,
["Khaki"] = 96,
["Lily white"] = 97,
["Seashell"] = 98,
["Pastel brown"] = 99,
["Light orange"] = 100,
["Medium red"] = 101,
["Burgundy"] = 102,
["Reddish brown"] = 103,
["Cork"] = 104,
["Burlap"] = 105,
["Beige"] = 106,
["Oyster"] = 107,
["Mid gray"] = 108,
["Brick yellow"] = 109,
["Nougat"] = 110,
["Brown"] = 111,
["Pine Cone"] = 112,
["Fawn brown"] = 113,
["Sand red"] = 114,
["Hurricane grey"] = 115,
["Cloudy grey"] = 116,
["Linen"] = 117,
["Copper"] = 118,
["Dark orange"] = 119,
["Dirt brown"] = 120,
["Bronze"] = 121,
["Dark stone grey"] = 122,
["Medium stone grey"] = 123,
["Flint"] = 124,
["Dark taupe"] = 125,
["Burnt Sienna"] = 126,
["Really black"] = 127
}
then it’s just colorLookup[“White”] for example