Heyo so I’ve been trying to make a teleport system using messaging service for a match making system and I feel like I’m on the right track, but I’m struggling with actually getting the players for teleporting. Here’s the code I currently have.
local Queue = {}
Main.MS:SubscribeAsync("Teleport",function(Message)
warn("Teleporting Players")
local Code = Message.Code
Main.TS:TeleportToPrivateServer(6962511190,Code,Queue)
end)
Main.MS:SubscribeAsync("JoinQueue",function(Message)
local PlayerUserId = Message.Data
for _,Player in pairs(Main.Players:GetPlayers()) do
if Player.UserId == PlayerUserId then
warn(Player.Name.." ("..Player.UserId..") Is joining the queue")
table.insert(Queue,Player)
end
end
warn("Printing Queue")
for _,Player in pairs(Queue) do
warn(Player.Name)
end
--if #Queue >= 2 then
-- local Code = Main.TS:ReserveServer(6962511190)
-- Main.MS:PublishAsync("Teleport",Code)
--end
end)
Main.Remotes.MatchMaking.OnServerEvent:Connect(function(Player)
if not table.find(Queue,Player.UserId) then
Main.MS:PublishAsync("JoinQueue",Player.UserId)
else
Main.MS:PublishAsync("LeaveQueue",Player.UserId)
end
end)
In the Teleport
topic’s callback, your aim is to teleport all players in the queue to the place id 6962511190
.
When the JoinQueue
topic is published, the player’s userid is added to the array Queue
.
TeleportService’s TeleportAsync
provides granular control logic over teleportation and is supposed to be the one-stop solution to unify all of the other teleporting functions.
In the ‘Teleport’ topic’s callback:
Initialize an empty array. This will contain candidates of players that need to be teleported.
local candidates = {}
Since you’re teleporting to a reserved server, you need to create special teleport options. This can be done with the following:
local options = Instance.new("TeleportOptions")
options.ShouldReserveServer = true
By setting options.ShouldReserveServer
to true, we’re telling the teleportation system to automatically reserve a server for us and teleport the player(s) to that server. This removes the need for
Main.TS:ReserveServer(6962511190)
and removes the need for you to pass the access code through MessagingService.
Loop through every player in the game and determine if their userid exists in the queue using table.find
. If the player exists, add them (not their UserId, the actual player instance) to the array of candidates. When the loop is finished, it is appropriate to call TeleportService’s TeleportAsync
if any candidates are found.
if #candidates > 0 then
TeleportService:TeleportAsync(6962511190, candidates, options)
end
Well I don’t wanna teleport all of them at the same time to the same server I’m making a fighting game which is gonna be 1vs1 so I need to send 2 players at a time to a server.
Select 2 players from the queue at a time and run the same logic. I forgot to add to also remove their UserId from the queue once they’re teleported.
Ah alright. By the way just a bit confused would I do all of this within the Main.MS:SubscribeAsync("JoinQueue",function(Message)
? Or once I add the userid to the queue would I use the Teleport one? I think I’m just confused because I don’t know what “callback” means.
Edit: Wait would this work if the players are in different servers? I probably didn’t do this right, but here’s I coded it
Main.MS:SubscribeAsync("Teleport",function(Message)
local Candidates = {}
local Options = Instance.new("TeleportOptions")
Options.ShouldReserveServer = true
warn("Printing Queue")
for _,UserId in pairs(Queue) do
print(UserId)
end
warn("Printing Players")
for _,Player in pairs(Main.Players:GetPlayers()) do
print(Player.Name)
if table.find(Queue,Player.UserId) then
table.insert(Candidates,Player)
end
end
warn("Printing Candidates")
for _,Player in pairs(Candidates) do
print(Player.Name)
end
if #Candidates > 0 then
--Main.TS:TeleportAsync(6962511190,Candidates,Options)
end
end)
Output
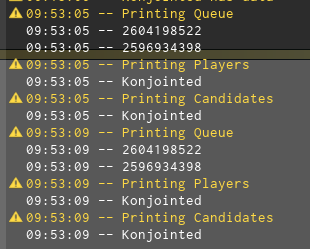