Hello everyone,
I’m currently making a popup system for my game but I have an issue.
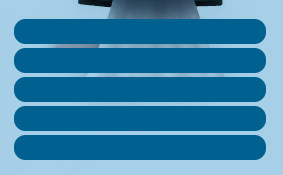
as you can see I’m trying to make it look like this and if the script takes it’s time then it would have no issue. However, when you spam it you get something like this:
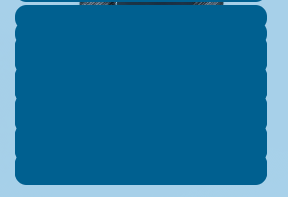
Of course the game won’t spam it this much but the issue will still persist if I called the function twice from 2 different scripts
Here is the script:
local Player = game.Players.LocalPlayer
local TweenService = game:GetService("TweenService")
local Tweeninfo = TweenInfo.new(0.5,Enum.EasingStyle.Linear)
local function UpdateAllGui()
for i,v in ipairs(Player.PlayerGui.PopupUI:GetChildren()) do
local Tween = TweenService:Create(v,Tweeninfo,{Position = v.Position - UDim2.fromScale(0,0.050)})
Tween:Play()
end
end
local GuiFunction = {}
function GuiFunction.InfoPop(Text)
local UIClone = script.Infopopup:Clone()
local MoveTween = TweenService:Create(UIClone,Tweeninfo,{Position = UIClone.Position - UDim2.fromScale(0,0.027)})
local SizeTween = TweenService:Create(UIClone,Tweeninfo, {Size = UIClone.Size + UDim2.fromScale(0.189,0)})
UIClone.Parent = Player.PlayerGui.PopupUI
MoveTween:Play()
MoveTween.Completed:Wait()
UpdateAllGui()
SizeTween:Play()
end
return GuiFunction
Is there a way to make it the same as the first picture regardless of how many times the function is called at once? Thank you.
Hi, what about a debounce to prevent the spamming you’re facing? – Possible with a queue table
won’t that cause other function calls to be canceled? like I call the function from 2 different scripts and if there is a debounce one of the scripts won’t get their own popup
That is why I suggest the queue table.
I’m sorry but I have no idea what you mean by a queue table? can you explain please
ok so I tried implementing the queue table but it seems to bug out the entire function once I spam a bit
not sure if I did it right or not but here is the modified script:
local Player = game.Players.LocalPlayer
local TweenService = game:GetService("TweenService")
local Tweeninfo = TweenInfo.new(0.1,Enum.EasingStyle.Linear)
local GuiQueue = {}
local GuiQueueInProgress = false
local function UpdateAllGui()
for i,v in ipairs(Player.PlayerGui.PopupUI:GetChildren()) do
local Tween = TweenService:Create(v,Tweeninfo,{Position = v.Position - UDim2.fromScale(0,0.050)})
Tween:Play()
end
end
local GuiFunction = {}
function GuiFunction.InfoPop(Text)
local UIClone = script.Infopopup:Clone()
GuiQueue[UIClone] = true
local MoveTween = TweenService:Create(UIClone,Tweeninfo,{Position = UIClone.Position - UDim2.fromScale(0,0.027)})
local SizeTween = TweenService:Create(UIClone,Tweeninfo, {Size = UIClone.Size + UDim2.fromScale(0.189,0)})
if not GuiQueueInProgress then
GuiQueueInProgress = true
for Popup,_ in pairs(GuiQueue) do
UIClone.Parent = Player.PlayerGui.PopupUI
MoveTween:Play()
MoveTween.Completed:Wait()
UpdateAllGui()
SizeTween:Play()
SizeTween.Completed:Wait()
task.wait(1)
UIClone:Destroy()
end
GuiQueueInProgress = false
end
end
return GuiFunction
Remember to remove the Popup from the queue again, by setting it to nil
The Parent property of Infopopup is locked, current parent: NULL, new parent PopupUI
if not GuiQueueInProgress then
GuiQueueInProgress = true
for Popup,_ in pairs(GuiQueue) do
UIClone.Parent = Player.PlayerGui.PopupUI
MoveTween:Play()
MoveTween.Completed:Wait()
UpdateAllGui()
SizeTween:Play()
SizeTween.Completed:Wait()
task.wait(1)
GuiQueue[Popup] = nil
UIClone:Destroy()
end
GuiQueueInProgress = false
end
it still throws the same error
I would love the gui to be positioned correctly rather then preventing the spam if that’s possible
I cannot tell you what’s wrong then. But the setup should be: Frame with UIListLayout, containing all incoming popups.
And for the popup frame itself, have a parentframe, and then a childframe. Make sure to tween the childframe and not the parentframe, since the parentframe is the one keeping the correct position.
Just as a quick note, I don’t believe you can tween positions of frames affected by UIListLayout
as @PuffoThePufferfish said:
it’s not possible to tween frames inside the UIListLayout, that’s why I’m looking for another way to sort the popups
That is why you have the childframe to tween, and not the parentframe as mentioned above. 
Edit:
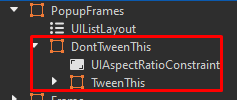
Look at my image above, it shows you the setup. Also in the post with the link I sent you, I have attached an image of what it looks like.
I will try this now and see the results thanks for your help
Make sure that LayoutOrder = tick(), since the newest comes on top then. If reverse just use a minus infront of tick()
so after a few trails and error I managed to pull it off thanks to you but I have another question, How could I limit the amount of popups in the screen and delete the old ones?