I’m making a vehicle flip script at the moment and I was wondering how you would make a model keep it’s X, and Y Rot but make the Z Rot 90
My current code:
local player = script.Parent.Parent.Parent.Parent.Name
local workplr = game.Workspace:FindFirstChild(player)
script.Parent.MouseButton1Click:Connect(function()
local curpivot = workplr.Vehicle:GetPivot()
workplr.Vehicle:PivotTo(curpivot * CFrame.new(0,0,0))
end)
you could use math.randon(1,100) but to make a car it is better to use velocity in the wheel
here is the spinning script i use
while true do
wait()
script.Parent.CFrame = script.Parent.CFrame * CFrame.fromEulerAnglesXYZ(0,0,math.pi/100)
end
it is going to speen the wheel but not too
fast try to change numbers and see what happens
Use a BodyGyro instead so it looks a little more pleasing. Start with MaxTorque 0, 0, 0 and when it’s flipped over, change the MaxTorque to 4e5, 0, 4e5.
What would the CFrame be, like the properties of it?
If you don’t set the properties of BodyGyro, then it defaults to CFrame.new(0, 0, 0). You would actually not need to set any of the CFrame, just use the MaxTorque. MaxTorque 4e5, 0, 4e5 works because from my experience, CFrame.Angles(Controls up / down, sideways, rolling). You can set it how you want, however. Also note that the BodyGyro should be on a part with default orientation (0, doesn’t matter, 0).
So create a part that’s 0,x,0?
and actually just type 4e5?
Add a BodyGyro into the main part of your car that does not have any x or z Orientation. Then, set its MaxTorque to 0, 0, 0.
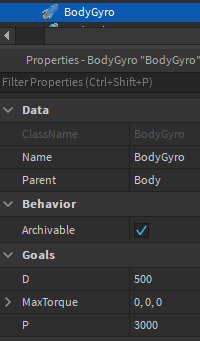
For your script, use a debounce and set MaxTorque to 4e5, 0, 4e5 when flipping over like this:
local player = script.Parent.Parent.Parent.Parent
local character = workspace:FindFirstChild(player.Name)
local gyro = script.Parent["BODYGYRO DIRECTORY"]
local TORQUE_UP = Vector3.new(4e5, 0, 4e5)
local TORQUE_ZERO = Vector3.new()
local debounce = false
script.Parent.MouseButton1Click:Connect(function()
if debounce then return end
debounce = true
gyro.MaxTorque = TORQUE_UP
task.wait(2)
gyro.MaxTorque = TORQUE_ZERO
task.wait(4) -- Buffer so people don't spam flipping over
debounce = false
end)
Yea, you could use a body gyro or your could just change the rotational values of your CFrame.
For the cframe method, you could get the rotational angles of your cframe from cframe:ToOrientation(), keep the x and y rotational values the same, but have the z rotational value some radian/ degrees.
local object = workplr.Vehicle
local Xcf, Ycf = object.CFrame:ToOrientation()
object.CFrame = CFrame.new(object.CFrame.Position) * CFrame.Angles(Xcf, Ycf, math.rad(90))
1 Like
Wouldn’t CFrame.fromOrientation
be better since you’re using :ToOrientation()
Also that assumes that the car is rotated at exactly 90 degrees from the ground. What if it was completely flipped over?
The best method is to reset everything but the y value. See here:
local object = workplr.Vehicle
local rX, rY, rZ = object.CFrame:ToOrientation()
object.CFrame *= CFrame.fromOrientation(rX, 0, rZ):Inverse()
1 Like
whats the mainpart of my car? I’m not very smart so sorry for asking
It’s whatever you choose. Usually, it’s the part in the middle that every other part connects to. There are some models that already have it set by other people in the model’s PrimaryPart.
I don’t have that, It’s a old style roblox vehicle for a Neighborhood of Robloxia V.4 recreation that i’m helping one of the scripters out with
Then pick whichever part you feel is the “core”, “middle”, or “main” part of the car.
For example, let’s say I have a butterfly. There are 2 wings and a body. The main part would be the body because it connects the rest of the butterfly together and is the middle.
Another question that never has a value thats not 0,x,0?
I was talking about Orientation in the Part properties. You don’t need to check it, though. I can alter the script so it takes that into consideration.
question number 99999
it turns out they are all welded does this mean i could put it on any?
Look at the part0 of those welds. If it repeats, then that could be the main part that everything is welded to.