Alright, I think I have a solution. Assuming that you want a cooldown for each player I think this will work best. I tried to keep exploiters in mind and to not trust the client, so I will only send requests to the client with this one.
First, this is how I set up the system if you want to call it; in the explorer.
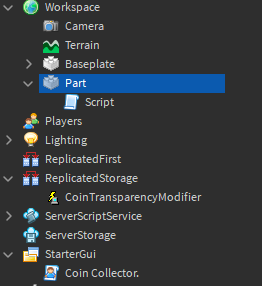
Now to the scripting part. If you are unfamiliar with some of the things in here, there are many YT videos that will explain it well. The API reference manual is a good place to look as well.
https://developer.roblox.com/en-us/api-reference
First, we will get started with the Client Script this is located inside the StarterGui and will make the coin invisible to you but not other players.
In the future, if you need help with scripting; you can use these " ``` " to make a code block.
Ex.
--code here
But onto the script.
local ReplicatedStorage = game:GetService("ReplicatedStorage");
local CoinTransparencyEvent = ReplicatedStorage:WaitForChild("CoinTransparencyModifier");
CoinTransparencyEvent.OnClientEvent:Connect(function(Object, CollisionSetting, CoinTransparencySetting)
Object.Transparency = CoinTransparencySetting;
Object.CanCollide = CollisionSetting;
end)
This script is short as it does not need to be used for much.
Now to the code that will be inside your coin.
local MemoryBank = {}; -- this is a table. you can store vales in here with it.
local CoinValue = 1; -- the amount that the coin is worth.
local WaitTime = 3; -- Seconds. The cooldown time for the coin.
local Coin = script.Parent; -- The coin
local ReplicatedStorage = game:GetService("ReplicatedStorage"); -- this gets the replicated storage
local Players = game:GetService("Players"); -- this gets the players
local CoinTransparencyEvent = ReplicatedStorage:WaitForChild("CoinTransparencyModifier"); --remote event. Passes infomation to client
local function OnTouch(Hit)
print("Hit");
if Hit.Parent:FindFirstChildWhichIsA("Humanoid") then
local Player = Players:GetPlayerFromCharacter(Hit.Parent);
local CoinsValue = Player:WaitForChild("leaderstats"):WaitForChild("Coins");
if not table.find(MemoryBank,Player) then
warn("Added coins");
CoinsValue.Value += CoinValue;
table.insert(MemoryBank,Player);
CoinTransparencyEvent:FireClient(Player,Coin, false, 1);
wait(WaitTime);
pcall(function()
local Num = table.find(MemoryBank, Player);
table.remove(MemoryBank,Num);
CoinTransparencyEvent:FireClient(Player,Coin, true, 0);
end)
end
end
end
Coin.Touched:Connect(OnTouch)
This is more complicated than your original code as it adds players to a table
to see if the player has touched the coin.
I should explain the RemoteEvent
now.
CoinTransparencyEvent:FireClient(Player,Coin, false, 1);
The player is the client we are wending the information over to. The coin is the coin we are changing the values of. false
is use setting the CanCollide. 1
is setting the transparency.
I added this so you can change the properties of the coin on the client to your liking.
All you need to do now is add a remote event to ReplicatedStorage
, name it CoinTransparencyModifier
, and you should be done.
Hope this helps!