-
Trying to save a string value
-
I am having trouble saving the string value.
local dataStore = game:GetService("DataStoreService"):GetDataStore("myDataStore")
game.Players.PlayerAdded:Connect(function(plr)
local playerUserId = "Player_"..plr.UserId
local data
local success, errormessage = pcall(function()
data = dataStore:GetAsync(tostring(playerUserId))
end)
if success then
if data ~= nil then
plr.Character.FirstName.Value = data[1]
plr.Character.LastName.Value = data[2]
end
end
end)
game.Players.PlayerRemoving:Connect(function(plr)
local playerUserId = "Player_"..plr.UserId
local data = {plr.Character.FirstName.Value, plr.Character.LastName.Value}
local success, errormessage = pcall(function()
dataStore:SetAsync(tostring(playerUserId), data)
end)
if success then
print("Data successfully saved.")
else
print("Error when saving data.")
warn(errormessage)
end
end)
1 Like
What’s the issue? Does the data not load? Are there errors?
Keeps telling me attempt to index nil with ‘FirstName’
Does it occur within the PlayerAdded
event or the PlayerRemoving
event?
If it occurs in the PlayerAdded
event, then that means that by the time the script gets the portion where it’s suppose to apply the DataStore values, the ValueBases
have not yet been added to the player’s character.
If it occurs in the PlayerRemoving
event, then that means that the ValueBases
have been destroyed before the script could save the data.
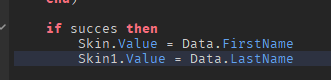
It happens here, on playeradded on Data.FirstName
Judging by your screenshot, I assume you forgot to check if Data
is not nil
before proceeding
Would I just add after if success then if Data ~= nil then
1 Like
So it says data isn’t nil but when I print the data.LastName it returns blank.
That’s because (I assume) you didn’t make a default value to be placed into the StringValues when Data
is nil
.
Example:
if success then
if data ~= nil then
--...
-- inserts the datastore's values
else
--...
-- inserts default values from the script
end
end
I put a default value and now that is all it is saving. When I leave it saves the default value instead of the new value.
Make sure you’re testing it in-game, studio’s DataStoreService can behave differently. And if you are testing in-game, I unfortunately can’t help you
can you try adding WaitForChild()