I’m attempting to check how far something is on the X axis to make a sort of border, I have a image attached below to visualise what I’m trying to exactly do.
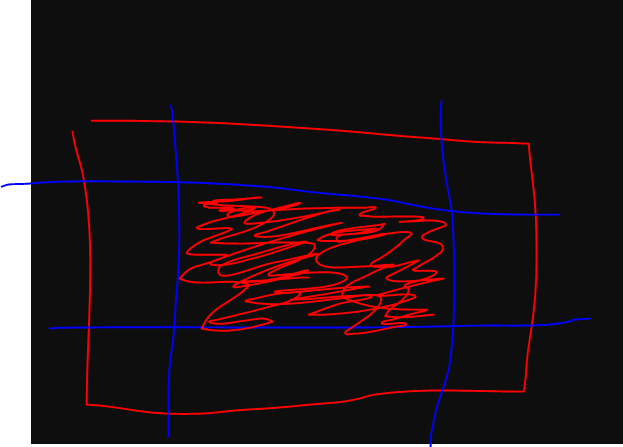
The shaded in red area is the area I want to check. Below is my attempt of it, though it hasn’t really been working at all.
local Player = game.Players.LocalPlayer
local character = Player.Character
local HumanoidRootPart = character:WaitForChild("HumanoidRootPart")
local Workspace = game:GetService("Workspace")
local RunService = game:GetService("RunService")
local static = workspace.CircleHelp.Model.PartToCheck
local renderStepped = RunService.RenderStepped
local connection
local MaxDistance = 50
connection = renderStepped:Connect(function()
wait(3)
local Vector, IsVisible = Workspace.CurrentCamera:WorldToScreenPoint(static.Position)
if IsVisible and Vector.X <= MaxDistance and Vector.X >= 20 then -- closest
print("close")
elseif IsVisible and Vector.X <= MaxDistance and Vector.X <= 20 then -- furthest
print("far")
else
print("not at all")
end
end)
I don’t know about the X, but I made a short function to get the distance in from a given camera to the specified part.
function Get_Distance(Cam:Camera,Part:BasePart)
return (Cam.CFrame.Position-Part.Position).Magnitude
end
Distance = Get_Distance(workspace.CurrentCamera,YOUR_PART_HERE)
-- Replace "YOUR_PART_HERE" with the part you're trying to check
Hope this helps!
Getting the distance on the X axis is actually a lot simpler than it might seem at first. Since a Vector3 is just a collection of lengths on the X, Y and Z axis you can calculate the distance on the X axis like this:
local Distance = TargetVector3.X - OriginVector3.X
The target represents the point in space you want to calculate the distance to and the origin is the point you are calculating the distance from. The resulting distance ends up being the distance from the origin to the target. If you only want the magnitude or rather the absolute distance, you can pass the result to the math.abs()
function. That way your distance will never be negative, even if the target is behind the origin.
To get your desired behaviour all you have to do is replace the origin with your cameras position and the target with the position you want to get the distance to the camera for.
Responded to the wrong post.
Would this work for checking how far something is on the camera? Not sure how to apply this to that.
I believe the problem you’re having lies with how you define your border. WorldToScreenPoint()
returns the position on the screen. This positions range varies with device resolution. A 1080p screen will for example have a range of 0 to 1920 for the X axis. Try creating a GUI at the position you want to check and then check if the given position is contained within the UI bounds. That way you don’t have to worry about scaling as Roblox can handle that for you. You can use the UI elements AbsolutePosition
and AbsoluteSize
properties for the calculations.