So I followed every step in the SQLite3 Database tutorial and now I’m stuck at how to operate it.
Here is what I have so far.
const databaseFile = ‘./.data/rbx-sqlite3-db003.db’; // If you need to reset EVERYTHING, you can change this.
const tables = [ // These are created once if alwaysCreateTables is false, however if it is set to true these all will be made each time (will not reset data, but will create any new table entries)
"table1", // All tables have two rows, key and value
"table2",
"table3"
];
const alwaysCreateTables = true;
const ApiToken = "secret"; // Highly recommended: https://www.grc.com/passwords.htm
const tableKeyLength = 150;
const tableValueLength = 3500;
const getAsyncAllowStar = true; // Allow "*" to be sent to the server to return all data from a table.
/*
SETTINGS ABOVE
*/
var $stmt;
var fs = require('fs');
const sqlite3 = require('better-sqlite3');
var dbExists = fs.existsSync(databaseFile);
const Database = new sqlite3(databaseFile);
const json = JSON.stringify;
var express = require('express');
var bodyParser = require('body-parser');
var app = express();
app.use(bodyParser.json());
if (!dbExists || alwaysCreateTables) {
/* fill database with tables */
tables.forEach(function(v, i) {
Database.prepare("CREATE TABLE IF NOT EXISTS "+v+" (key VARCHAR("+tableKeyLength+") PRIMARY KEY, value VARCHAR("+tableValueLength+") NOT NULL)").run();
});
}
function isValidTable(tab) {
return (tables.indexOf(tab) !== -1); // prepared statements don't allow you to specify a table, so we have to whitelist tables specified above.
}
app.delete("/deleteAsync", function(req, res) {
if (req.headers["apitoken"] == ApiToken) {
if (req.body.Key != null && req.body.Table != null) {
if (isValidTable(req.body.Table)) {
$stmt = Database.prepare("DELETE FROM `"+ req.body.Table +"` WHERE `key`=?");
var data = $stmt.run(req.body.Key);
res.status(200);
res.send(json({
Success: true,
KeyDeleted: data.changes > 0
}));
} else {
res.status(404);
res.send(json({
Success: false,
Message: "Table doesn't exist"
}));
}
} else {
res.status(400);
res.send(json({
Success: false,
Message: "Invalid request"
}));
}
} else {
res.status(403);
res.send(json({
Success: false,
Message: "You are unauthorized to make requests to this host."
}));
}
});
app.post("/postAsync", function(req, res) {
if (req.headers["apitoken"] == ApiToken) {
if (req.body.Key != null && req.body.Value != null && req.body.Table != null) {
if (isValidTable(req.body.Table)) {
$stmt = Database.prepare("REPLACE INTO `"+ req.body.Table +"` (key, value) VALUES (?, ?)"); // Create value if not exist, change value if exist.
var changes = $stmt.run(req.body.Key, req.body.Value).changes;
res.status(200);
res.send(json({
Success: true,
Changes: changes
}));
} else {
res.status(404);
res.send(json({
Success: false,
Message: "Table doesn't exist"
}));
}
} else {
res.status(400);
res.send(json({
Success: false,
Message: "Invalid request"
}));
}
} else {
res.status(403);
res.send(json({
Success: false,
Message: "You are unauthorized to make requests to this host."
}));
}
});
app.post("/getAsync", function(req, res) {
if (req.headers["apitoken"] == ApiToken) {
if (req.body.Key != null && req.body.Table != null) {
if (isValidTable(req.body.Table)) {
if (req.body.Key == "*" && getAsyncAllowStar) {
$stmt = Database.prepare("SELECT * FROM `" + req.body.Table +"`");
var data = $stmt.all();
res.status(200);
res.send(json({
Success: true,
ValueExists: data.length > 0,
Value: data
}));
} else {
$stmt = Database.prepare("SELECT * FROM `" + req.body.Table + "` WHERE `key`=?");
var data = $stmt.get(req.body.Key);
res.status(200);
res.send(json({
Success: true,
ValueExists: (!data == null),
Value: data
}));
}
} else {
res.status(404);
res.send(json({
Success: false,
Message: "Table doesn't exist"
}));
}
} else {
res.status(400);
res.send(json({
Success: false,
Message: "Invalid request"
}));
}
} else {
res.status(403);
res.send(json({
Success: false,
Message: "You are unauthorized to make requests to this host."
}));
}
});
app.all("/", function(req, res) {
res.send("Well, hello there Wanderer!");
});
var list = app.listen(process.env.PORT, function() {
console.log('Server Online, Port ' + list.address().port);
});
And:
{
"name": "rbx-mysql-server",
"version": "1.0.0",
"description": "Roblox MySQL Server",
"main": "server.js",
"scripts": {
"start": "node server.js"
},
"dependencies": {
"express": "^4.16.3",
"sqlite3": "^4.0.0",
"better-sqlite3": "^5.2.1"
},
"engines": {
"node": "8.x"
}
}
And:
local sql = {
ApiUrl = "https://primeverse.glitch.me", -- Do not use a trailing slash.
Token = "secret",
}
local http = game:GetService'HttpService';
local dummyFunction = function()end;
function sql:PostAsync(Table, Key, Value, Callback)
if (Table == nil) then
return error("Table is unspecified", 0);
elseif (Key == nil) then
return error("Key is unspecified", 0);
elseif (Value == nil) then
return error("Value is unspecified", 0);
elseif (Callback == nil) then
Callback = dummyFunction;
end
spawn(function()
local data = http:RequestAsync({
Url = sql.ApiUrl .. "/postAsync",
Method = "POST",
Headers = {
ApiToken = sql.Token,
["Content-Type"] = "application/json"
},
Body = http:JSONEncode{
Key = Key,
Value = Value,
Table = Table
}
})
local validJson, Resp = pcall(function()
return http:JSONDecode(data.Body);
end)
if (validJson) then
if (data.StatusCode == 200) then
Callback(true, Resp);
else
Callback(false, "Request did not succeed. [" .. data.StatusCode .. "]", Resp);
end
else
Callback(false, "Server returned invalid response", data.Body)
end
end)
end
function sql:DeleteAsync(Table, Key, Callback)
if (Table == nil) then
return error("Table is unspecified", 0);
elseif (Key == nil) then
return error("Key is unspecified", 0);
elseif (Callback == nil) then
Callback = dummyFunction;
end
spawn(function()
local data = http:RequestAsync({
Url = sql.ApiUrl .. "/deleteAsync",
Method = "DELETE",
Headers = {
ApiToken = sql.Token,
["Content-Type"] = "application/json"
},
Body = http:JSONEncode{
Key = Key,
Table = Table
}
})
local validJson, Resp = pcall(function()
return http:JSONDecode(data.Body);
end)
if (validJson) then
if (data.StatusCode == 200) then
Callback(true, Resp);
else
Callback(false, "Request did not succeed. [" .. data.StatusCode .. "]", Resp);
end
else
Callback(false, "Server returned invalid response", data.Body)
end
end)
end
function sql:GetAsync(Table, Key, Callback)
if (Table == nil) then
return error("Table is unspecified", 0);
elseif (Key == nil) then
return error("Key is unspecified", 0);
elseif (Callback == nil) then
return error("Callback is unspecified", 0);
end
spawn(function()
local data = http:RequestAsync({
Url = sql.ApiUrl .. "/getAsync",
Method = "POST",
Headers = {
ApiToken = sql.Token,
["Content-Type"] = "application/json"
},
Body = http:JSONEncode{
Key = Key,
Table = Table
}
})
local validJson, Resp = pcall(function()
return http:JSONDecode(data.Body);
end)
if (validJson) then
if (data.StatusCode == 200) then
Callback(true, Resp);
else
Callback(false, "Request did not succeed. [" .. data.StatusCode .. "]", Resp);
end
else
Callback(false, "Server returned invalid response", data.Body);
end
end)
end
return sql;
And:
sql = require(script.DatabaseService)
game.Workspace.Model.Event.Event:Connect(function()
wait(10)
if sql:GetAsync("ifnuke")==true then
print("hi")
end
local a = true
p(a)
a = false
p(a)
end)
function p(a)
sql:PostAsync("ifnuke", a)
end
but I keep getting this error: 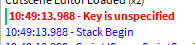