Hello.
So I’m making a city game, and there’d be a leader that controls the city. He has the ability to raise taxes, but once the leader dies, he can’t change the taxes anymore. I made a script that does it, but for the first leader (the first person to join the server), when he dies, the tax button doesn’t go away, and it says in the player’s output:
Infinite yield possible on 'TownGui:WaitForChild("TaxGui")'
however it goes away for the other people, and it doesn’t even say that. I don’t even get what’s wrong.
Thanks for helping, here’s script:
local localplayer = game.Players.LocalPlayer
local playergui = localplayer:WaitForChild("PlayerGui"):WaitForChild("TownGui"):WaitForChild("TaxGui")
while true do
if localplayer.Team == game.Teams.Leader then
workspace.FoodPanel.SurfaceGui.Frame.Increase.Visible = true
playergui:WaitForChild("Increase").Visible = true
playergui:WaitForChild("Decrease").Visible = true
else
workspace.FoodPanel.SurfaceGui.Frame.Increase.Visible = false
playergui:WaitForChild("Increase").Visible = false
playergui:WaitForChild("Decrease").Visible = false
end
wait(0.01)
end
6 Likes
“Infinite yield possible” means Roblox Lua :WaitForChild() is waiting for something to appear that doesn’t exist yet. It keeps checking and checking and checking, and after a while it says that in console to warn you that it could possibly be waiting forever for something to appear that will never exist. In this case, it is apparently your TaxGui. Have a look in your explorer window to see if your TaxGui is where it should be, which is as a child to your TownGui. Are you at any point for some reason deleting your TaxGui? Or only creating it at a specific point in the game? If so, then your script could be waiting for that to happen when it never will, hence infinitely yielding.
Also, you only need to use :WaitForChild() once, as :WaitForChild() will wait for all of the children of the child it is waiting for to be created before it runs the next line. For example - local playergui = localplayer:WaitForChild("PlayerGui").TownGui.TaxGui
would work just fine.
5 Likes
Yes, the Gui is instantly created when the game joins, but it says in the output:
TownGui is not a valid member of PlayerGui "Players.epic_4gaming.PlayerGui"
Even though it’s already there in PlayerGui, it only happens to the first player to join the server, other players don’t expierence it.
EDIT: I also did what you asked and replaced the playergui variable with yours, it just broke the entire script, and the error appeared for all players.
Do you have the Gui in StarterGui? Could you please upload a screenshot of your ui in the explorer window?
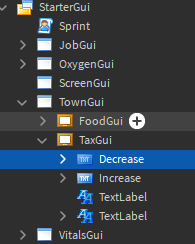
I tested the gui using the test menu on Studio with 4 players.
It seems like a strange bug? It works fine for me
I put the script in starterplayerscripts in StarterPlayer.
I also test the script using the test menu on studio,
The taxgui also only pops up for the person in the leader team in the game, here’s that script if you need it
local Players = game:GetService("Players")
local Teams = game:GetService("Teams")
local currentLeader = nil
local ServerStorage = game:GetService('ServerStorage')
local LeaderValue = ServerStorage:WaitForChild("CurrentLeader")
local function SelectRandomLeader()
return Players:GetPlayers()[Random.new():NextInteger(1, #Players:GetPlayers())]
end
Players.PlayerAdded:Connect(function(PlayerThatJoined)
PlayerThatJoined.CharacterAdded:Connect(function(CharacterThatJoined)
PlayerThatJoined.Team = Teams.Choosing
if currentLeader == nil then
currentLeader = PlayerThatJoined.Name
LeaderValue.Value = PlayerThatJoined.UserId
PlayerThatJoined:LoadCharacter()
PlayerThatJoined.Team = Teams.Leader
wait(0.1)
PlayerThatJoined.Character:SetPrimaryPartCFrame(workspace.CivilianSpawn.CFrame)
print(("%s is the current leader"):format(currentLeader))
end
repeat
wait()
until game.Players:FindFirstChild(currentLeader) == nil or game.Players:FindFirstChild(currentLeader).Character == nil or game.Players:FindFirstChild(currentLeader).Character.Humanoid.Health == 0
if true then
local NewLeader = SelectRandomLeader()
NewLeader.Team = Teams.Leader
game.Players[currentLeader].Team = Teams.Choosing
local character = NewLeader.Character or NewLeader.CharacterAdded:Wait()
character.HumanoidRootPart.Position = workspace.CivilianSpawn.Position + Vector3.new(0,5,0)
print(("%s has died. %s is the new leader."):format(currentLeader, NewLeader.Name))
LeaderValue.Value = NewLeader.UserId
currentLeader = NewLeader.Name
end
end)
end)
Players.PlayerRemoving:Connect(function(PlayerThatLeft)
if PlayerThatLeft.Team == Teams.Leader then
local NewLeader = SelectRandomLeader()
NewLeader.Team = Teams.Leader
game.Players[currentLeader].Team = Teams.Choosing
local character = NewLeader.Character or NewLeader.CharacterAdded:Wait()
character.HumanoidRootPart.Position = workspace.CivilianSpawn.Position + Vector3.new(0,5,0)
print(("%s has left. %s is the new leader."):format(currentLeader, NewLeader.Name))
LeaderValue.Value = NewLeader.UserId
currentLeader = NewLeader.Name
end
end)
I tried with 2 players and changed it to suit StarterPlayerScripts and it seems to work for me? (Ignore the failed to load plugin icon error.) Your server side code fortunately shouldn’t make a difference as it isn’t directly interacting with the ui. Try this!
local playergui = localplayer.PlayerGui:WaitForChild("TownGui").TaxGui
TaxGui is not a valid member of ScreenGui "TownGui"
Nopee. Did what you asked. There’s also have to be a leader team so the other team can’t see the button, only the leader can.
The script is throwing an error before it even checks what team the player is on, so the bug is unrelated to this. Could you maybe re-create what you see from my screenshots on just a new blank Roblox place, and test it there? I believe this is a Roblox bug, as it works completely fine on my end.
Have you included the entire localscript in the script you have sent me? If you haven’t then please could you send the entire thing, and tell me which line the script is throwing an error on?
Yes, I sent the entire script, and it’s throwing the line at the playergui variable.
I also just tested it on a different place, same issue. When I checked the first player to join the server in explorer on the client, PlayerGui isn’t there, when I check via the server, it’s there, maybe it’s that? How do I fix it?
Sometimes when :WaitForChild
shows an error I do.
while game:FindFirstChild("ShouldExistSoon") == nil do
wait(1)
end
Don’t do this. The warning means something.
So that means the code is correct and working, just for some reason Roblox isn’t creating PlayerGui for your second players client when testing, which causes either an infinite yield or ‘not a valid member’ error because what you are looking for isn’t being created by the Roblox client.
How are you testing your game with 2 players? You should be testing with 2 players via - test → select 2 players from dropdown menu → start

I did, testing with Roblox Studio, but same issue.
Nope, I did what you said, and it said
"TaxGui is not a valid member of ScreenGui “TownGui”
If this is a Roblox Bug, I can’t really post in Bug reports, so I wont be able to work on the project till Roblox updates.
Sometimes the game takes too long to load so it prints the error.
1 Like
Did you try suggestion #1, testing with a friend? I also forgot to suggest you could move the script to a different location, e.g. inside StarterGui, or alternatively do what @EvidenceCross suggested, however I don’t recommend this as it is a code smell.
You could also try checking you don’t have any other scripts that could be interfering with Roblox when it is setting up
2 Likes