I can confirm that the value is in fact created, so the issue is most likely related to your code stopping at the module script method. Any errors displayed in the output? In case you’d like this value to be inserted into player’s character, you have to parent it there instead. Could it be that you are looking for the value on the wrong spot?
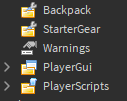
EDIT
Does the value spawn in this case?
Code
-- local Module = require(script.Parent:WaitForChild("ServerAdmin"):WaitForChild("ModuleScript"))
game.Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(char)
local Warning = Instance.new("IntValue")
Warning.Name = "Warnings"
-- Warning.Value = Module.LoadWarnings(player)
Warning.Parent = player
end)
end)
game.Players.PlayerRemoving:Connect(function(player)
Module.SaveWarnings(player)
end)
EDIT 2
@SOTR654 you were right, it was a path problem. Just in case you were wondering, both, this and ServerAdmin script were stored inside ServerScriptService, to which you can’t refer with
-- Incorrect
local ServerScriptService = script.Parent
-- Correct
local ServerScriptService = game:GetService("ServerScriptService")
-- or
local ServerScriptService = game:FindService("ServerScriptService")
The difference between :GetService() and :FindService() is that the former doesn’t create required service in case it doesn’t find it in game.
As for require(), it’s lua global that doesn’t allow any time out arguments, however, the following for scenarios apply.
- require() without an argument, with an invalid argument.
local Module = require()
-- OUTPUT
-->> Attempted to call require with invalid argument(s).
- require() with yielding :WaitForChild().
local Module = require(script:WaitForChild("ModuleScript")) -- no module
-- OUTPUT
-->> Infinite yield possible on 'ServerScriptService.Script:WaitForChild("")
- require() wrapped in / secured with pcall().
pcall(function()
local module = require() -- invalid argument
end)
print("Hello beautiful world!") -- prints
- require() wrapped in / secured with pcall(), but combined with :WaitForChild() and no time out causes infinite yield.
It is true that require() used with :WaitForChild(), time out, and wrapped in pcall() works, but usually doesn’t change anything for better, as the script often need module scripts if they require them.