Hi! So I’m trying to make players invisible even if they reset/a new player joins. This is not working for some reason. After respawning, the player is visible again.
Code: (I don’t think the full code is necessary)
game.Players.PlayerAdded:Connect(function(plr)
plr.CharacterAdded:Connect(function(char)
if isInvisible then
changeTransparencyTo1()
end
end)
end)
for i,v in next, game.Players:GetChildren() do
v.CharacterAdded:Connect(function(char)
if isInvisible then
changeTransparencyTo1()
end
end)
end
-------------------------------------------------------------
textButton.MouseButton1Click:Connect(function()
if isInvisible == false then
isInvisible = true
--Make them invisible
changeTransparencyTo1()
elseif isInvisible == true then
isInvisible = false
--Make the visible again
changeTransparencyTo0()
end
end)
The issue looks to be that the code is only ran when PlayerAdded
fires which is only fired when a player connects to the game. A solution would be to have the client fire an event every time their character loads, have the server listen for the event and run changeTransparencyTo1()
.
1 Like
So the local script of the player who died should fire a Remote Event and the local script of the player who wants to make him invisible should have a onClientEvent that makes him invisible?
is the isInvisible boolvalue on your client side somewhere in the playergui?
If the ScreenGUI is set to “reset on spawn” it will reset the boolvalue back to where it was at default.
1 Like
No it’s just a variable in my script.
Where is this script located and what kind of script is it
1 Like
Under the TextButton and it’s a local script.
local isInvisible = false
And is the ScreenGui set to reset on respawn? it will turn the boolvalue back to default when you die if this value is set to true
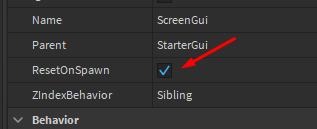
1 Like
No it’s not but that doesn’t really matter, I think. It would just make everyone visible again when the client dies but the problem is that when someone else dies he is not becoming invisible again.
^ Sounds confusing lol
The first part of your script with the PlayerAdded event is located serverside right?
if this is client side - you should not connect this function to all players ever joining the game, you should only connect this function to the client himself since you want to change ONLY the client in this case.
You can get the player as:
local player = game.Players.LocalPlayer
player.CharacterAdded:Connect(function(char)
end)
if this is serverside, I assume you search for the right isInvisible value on the client. Be aware that if you do this, you should do it with a remote event because if the player locally changes the boolvalue, it will not replicate to the server so the server will still think it is turned off.
Yes it is.
local textButton = script.Parent
local isInvisible = false
local isInvisText = "Show hidden Players"
local isNotInvisText = "Hide Players"
local function changeTransparencyTo1()
...
end
local function changeTransparencyTo0()
...
end
game.Players.PlayerAdded:Connect(function(plr)
plr.CharacterAdded:Connect(function(char)
if isInvisible then
changeTransparencyTo1()
end
end)
end)
for i,v in next, game.Players:GetChildren() do
v.CharacterAdded:Connect(function(char)
if isInvisible then
changeTransparencyTo1()
end
end)
end
textButton.MouseButton1Click:Connect(function()
if isInvisible == false then
isInvisible = true
--Make them invisible
changeTransparencyTo1()
...
elseif isInvisible == true then
isInvisible = false
--Make the visible again
changeTransparencyTo0()
...
end)
But I don’t want to change the client, I want to change the other players FOR the client so he can’t see them. This is a script that’s laggy but it works, maybe this gives you an idea of what I want to do:
--//Variables\\--
local textButton = script.Parent
local isInvisible = false
local runService = game:GetService("RunService")
local isInvisText = "SHOW HIDDEN PLAYERS" --Changeable
local isNotInvisText = "HIDE OTHERS" --Changeable
--//Functions\\--
local function changeTransparencyTo1()
...
end
local function changeTransparencyTo0()
...
end
--//Events\\--
textButton.MouseButton1Click:Connect(function()
if isInvisible == false then
isInvisible = true
--Make them invisible
pcall(function()
runService:UnbindFromRenderStep("UnInvis")
end) --Unbind the last Bind from the RenderStep, pcall to catch the error if it's not binded yet
runService:BindToRenderStep("Invis", Enum.RenderPriority.Camera.Value - 10, changeTransparencyTo1)
..
elseif isInvisible == true then
isInvisible = false
--Make them visible again
pcall(function()
runService:UnbindFromRenderStep("Invis")
end) --Unbind the last Bind from the RenderStep, pcall to catch the error if it's not binded yet
runService:BindToRenderStep("UnInvis", Enum.RenderPriority.Camera.Value - 10, changeTransparencyTo0) --Binds to the RenderStep
...
end
end)
I made this before. The problem with this is it uses RenderStepped and if there are like 20 players, it gets pretty laggy.
It works fine though.
Maybe add some wait statements to yield?
or do
local character = player.Character or player.CharacterAdded:Wait()
1 Like
@GEILER123456
did you add yielding or local character = player.Character or player.CharacterAdded:Wait()
to fix this issue?
Yep, it works fine now. I’ve simply added a wait(0.1)
1 Like
if isInvisible then
wait(0.1)
changeTransparencyTo1()