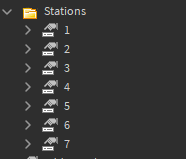
(The station names are stored in the values - eg. “1.Value” or “2.Value” - the station names aren’t numbers meaning I can’t just sort the table with the stations in it.)
The reason I can’t use the values in any order is because on the return journey the stations list needs to be read in reverse order (from 7 to 1) meaning I would need a way of sorting them by reverse alphabetical order too. I know you can sort tables but is there something similar I could use to sort this? Thanks!
local stationName = routeFolder.Stations:GetChildren()
for i = 1, #stationName do
--in here is a script that basically generates a timetable from the children of the Stations folder
end
2 Likes
If you are consistent with your naming of the Value instances (having them as numbers and not skipping a number like 1, 2, 6, 7, 8, 12) then you can easily just do the following to auto sort it without “table.sort.”
local tempArray = {}
for _, station in pairs(stationName) do
table.insert(tempArray, station.Name, station)
-- Reverse order
-- table.insert(tempArray, (#stationName + 1 - station.Name), station)
end
That should place each station into a temporary table(array), at the position of the instance name(1, 2, 3 etc), with the value of that index as the actual instance. You now have a sorted table with access to the instance to manipulate how you see fit. You can do the reverse order in a multitude of ways. I just provided one in the above example.
If you don’t keep the instance names consecutive, you will need to do something like above by creating a proxy table (which is just what was done above also) and then sorting that proxy table with table.sort.
1 Like
Sort the table with a custom sorting function.
For example, you might get routeFolder.Stations:GetChildren()
, which has an undefined order, and arrange it by child.Name
or child.Value
.
local stations = routeFolder.Stations:GetChildren()
-- stations has an undefined order
table.sort(stations, function(a, b) -- table.sort works in-place, it modifies the original table
return a.Name < b.Name
end)
-- stations is now {StringValue 1, StringValue 2, StringValue 3 etc.} (or in reverse - not sure)
If you want to go in reverse, you don’t have to sort the table again with a different sorting function.
This is how you loop through a table normally:
for i = 1, #stations do -- from 1 to 7, increasing by 1 each time
--in here is a script that basically generates a timetable from the children of the Stations folder
end
This is how you do it in reverse:
for i = #stations, 1, -1 do -- from 7 to 1, increasing by -1 (decreasing by 1) each time
-- ...
end
edit:
If your stations are consistently named from 1 to 7, then you may skip the sorting and just get them directly from the folder.
local stationChildren = routeFolder.Stations:GetChildren() -- undefined order, but gives us the amount of stations
local stationsFolder = routeFolder.Stations
for i = 1, #stationChildren do
local station = stationsFolder[i] -- i goes from 1 to 7 anyway
--in here is a script that basically generates a timetable from the children of the Stations folder
end
5 Likes