Hi, I want to fire a BindableEvent if any BoolValues values change inside this folder (in ServerStorage):
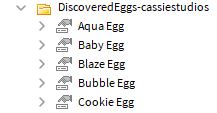
This is the script in ServerScriptService for now:
local sStorage = game:GetService("ServerStorage")
local repStorage = game:GetService("ReplicatedStorage")
local discoveredEggs = sStorage:WaitForChild("DiscoveredEggs")
local bindableEvent = repStorage:FindFirstChild("Bindable").Events.ValueChanged
discoveredEggs["Aqua Egg"]:GetPropertyChangedSignal("Value"):Connect(function()
bindableEvent:Fire()
end)
discoveredEggs["Baby Egg"]:GetPropertyChangedSignal("Value"):Connect(function()
bindableEvent:Fire()
end)
discoveredEggs["Blaze Egg"]:GetPropertyChangedSignal("Value"):Connect(function()
bindableEvent:Fire()
end)
--etc.
Is there a simpler or more effective way to do this?
Thanks to anyone who helps!
Well first of all instead of using GetPropertyChangedSignal
values have a built in .Changed method.
Second off you can loop through the folder that has the BoolValues, and for everyone just set up that changed listener
@RealCreepi ‘s script does what I just explained here
1 Like
Use the power of for loops!
local eggs = discoveredEggs:GetChildren()
for _, child in pairs(eggs) do
child.Changed:Connect(function()
bindableEvent:fire()
end)
end
1 Like
Thank you! I did think about doing this but I’m not sure how often I should run the loop because I don’t want to cause lag.
The loop only runs once for it to connect a function to the Changed
event. That’s it.
1 Like
Hmm… ok thanks. Does that mean it will detect when it changes after it’s finished running the loop? Sorry I’m pretty new to scripting.
Okay, let me explain the code:
-- we get all the eggs inside the folder
local eggs = discoveredEggs:GetChildren()
-- we iterate over every egg in the folder
for _, egg in pairs(eggs) do
-- we tell Roblox to call this function when the value of our egg is changed
egg.Changed:Connect(function()
-- your code
bindableEvent:fire()
end)
end
Changes to the value of the eggs will always be detected after we tell Roblox which function to call (egg.Changed:Connect(...)
)
1 Like
Awesome, thanks for explaining that.
If this is true, then why is this script not working? Let’s just say the Bindable Event prints ‘Bindable Event’ when fired, it’s not printing when the value changes. (The Bindable Event is inside a PlayerAdded function, I’m not sure if that has anything to do with it.)
Try debugging: what happens when you print(egg)
in the for loop?
1 Like
When I join, it prints all the egg BoolValues names & prints ‘Bindable Event’. When the value changes, it prints nothing. 
Is this code in a ServerScript or LocalScript and are you changing the value from a ServerScript or LocalScript?
1 Like
They are all ServerScripts. (3 0 chars)
That’s weird. I am as clueless as you are now too why it’s not firing when the value changes.
Good Luck
Thanks! I might start a new topic to see if anyone else can help.