To understand how vectors work in general, you’re best bet is to use resources not necessarily for Roblox, so that you can understand the premise behind it - I recommend:
Next, you can apply this new knowledge to Roblox. Using the wiki page,
you can experiment with different things.
A summary of what you need to know about Vectors
A Vector3 is a essentially a point in Roblox space, consisting of 3 coordinates, x, y and z.
Edit:
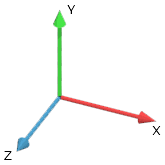
These points can be assigned to various objects within Roblox, mostly objects known as “BaseParts” - giving them a direct position in the “world”.
The size of a part is defined using Vector3 as well.
In Roblox, Vectors are defined using studs. If you made a part with a Vector3 size of (1,1,1), it would be 1 stud tall, 1 stud wide and 1 stud deep. (a cube).
Likewise, if this part was placed at a Vector3 of (5,0,0), it would be placed 5 studs to the right of the origin (the origin being 0,0,0)
Example code to demonstrate:
local part = Instance.new("Part") -- creating the part object
part.Parent = workspace -- parenting the part to the game's workspace, in the 'world'
part.Size = Vector3.new(5,5,5) -- a cube of 5 by 5 by 5 studs.
part.Position = Vector3.new(0,10,0) -- placed 10 studs above the origin in the air.
What is Vector3.new you ask?
When creating a new Vector3, you use Vector3.new() to create a blank Vector3 of x = 0, y = 0, z = 0.
Vector3.new() takes 3 optional arguments (x,y,z) - x, y and z can be basically any float
A problem with Vector3 however, is it doesn’t allow rotation.
To implement rotation, you will need to use CFrame:
Similarly, to create a CFrame, you use CFrame.new(x,y,z)
part.CFrame = CFrame.new(0,10,0) -- the first 3 arguments are a vector3
-- but how would I rotate it? --
part.CFrame = CFrame.new(0,10,0, Vector3.new(5,10,0)) -- to rotate it to look towards a point 5 studs to its right.
-- to rotate it by degrees --
part.CFrame = CFrame.new(0,10,0) * CFrame.Angles(0,90,0)
-- Alternatively, you can rotate *only* using Vector3, using the property "Orientation" --
part.Position = Vector3.new(0,10,0)
part.Orientation = Vector3.new(0,45,0) -- rotated 45 degrees clockwise.
Unfortunately, I can’t cover everything you need to know for Vector3 and CFrame, so various tutorials and especially the Wiki are your best bet. Otherwise, just practise and experiment, eventually it will become clear. Hope this helped!
All of this code is completely untested and just an example coded directly here so it isn’t guaranteed to work out of the box.