Trying to make a door that opens after 3 seconds using OOP.
The door doesn’t work due to this error:
15:36:00.130 toggleMovement is not a valid member of Part "Workspace.CodeDoor.Door.Hinge" - Server - CodeDoor:9
I have 2 scripts, a server script and a module script. The module script is parented to the server script in serverscriptservice.
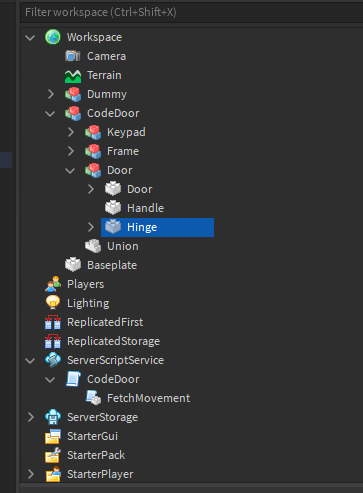
Here are my scripts:
Server script
local collectionService = game:GetService("CollectionService")
local tag = collectionService:GetTagged("CodeDoor")
local fetchMovement = require(script.FetchMovement)
for _, door in pairs(tag) do
local newDoor = fetchMovement:new(door.Door.Hinge)
wait(3)
newDoor:toggleMovement()
end
Module script
local tweenService = game:GetService("TweenService")
local movement = {}
movement.__index = movement
movement.open = false
movement.openTime = 2
function movement:new(object)
local newMovement = setmetatable({}, self)
newMovement.__index = newMovement
newMovement.Model = object
return object
end
function movement:moveObj(properties)
local tween = tweenService:Create(self.Model.Hinge, TweenInfo.new(self.openTime, Enum.EasingStyle.Sine, Enum.EasingDirection.In, properties))
return tween
end
function movement:toggleMovement()
self.open = not self.open
local properties = {}
properties[true] = {CFrame = self.Model.Hinge.CFrame * CFrame.Angles(0, math.rad(90), 0)}
properties[false] = {CFrame = self.Model.Hinge.CFrame * CFrame.Angles(0, 0, 0)}
local tween = self:moveObj(properties[self.open])
tween:Play()
end
return movement
I think it’s something wrong here, try this
function movement.new(object)
local newMovement = {}
setmetatable(newMovement, movement)
newMovement.Model = object
return newMovement
end
In your original code, you were returning the model again, so you were using a function that doesn’t exist for models, and with that change, you will need to do a slight change in your server script
local collectionService = game:GetService("CollectionService")
local tag = collectionService:GetTagged("CodeDoor")
local fetchMovement = require(script.FetchMovement)
for _, door in pairs(tag) do
local newDoor = fetchMovement.new(door.Door)
wait(3)
newDoor:toggleMovement()
end
Judging from how you were using the code, seems your original method would still error because you give it the hinge as the model, rather than the doro model
Also I would check out the toggleMovement
method afterwards as your opening and closing is a bit off
I was about to reply that I solved it by doing that before you even replied lol. Thanks anyway.
Also, shouldn’t you set the __index?
I presume you’re doing OOP? It’s not really needed since you can set the metatable to the movement
template you have and return that. Should still work eitherways
And why is my opening and closing a bit off?
I’m not sure if I’m mistaking it, cause in your toggling, I don’t think it’ll close the door properly since it’ll keep it at the position it was in originally when firing it again. Not sure if
properties[false] = {CFrame = self.Model.Hinge.CFrame * CFrame.Angles(0, math.rad(-90), 0)}
Would be what is needed, but if your original code for it works it should be fine
Oh no, it sets it to the origin again. It worked fine. Also I have run into a really odd issue.
local collectionService = game:GetService("CollectionService")
local tag = collectionService:GetTagged("CodeDoor")
local fetchMovement = require(script.FetchMovement)
for _, door in pairs(tag) do
local newDoor = fetchMovement:new(door)
wait(10)
newDoor:toggleMovement()
end
At the wait(10)
part, for some reason it doesn’t wait 10 seconds. It waits, like, 5 I think.
I’m not sure if that’s an issue with the modulescript or something else, are you certain it’s waiting less than told? Looks to me it should work
Yes, it is working less than told.
That’s odd, maybe try doing the changes I did to both the ModuleScript and the server script and see if that does soemtihng?
2 Likes