Hello everyone, I am trying to make it so when a players mouse hovers over a button (object) a UI appears next to the mouse (please bare in mind my code is basic for now). When I tested this code, the UI did not show up, any idea what I’ve done wrong? All help is much appreciated!
--Buttons
local B1 = script.Parent:GetDescendants("Button_1")
local B2 = script.Parent:GetDescendants("Button_2")
local B3 = script.Parent:GetDescendants("Button_3")
local B4 = script.Parent:GetDescendants("Button_4")
local B5 = script.Parent:GetDescendants("Button_5")
local B6 = script.Parent:GetDescendants("Button_6")
--Services & Plr
local UIS = game:GetService("UserInputService")
local Player = game.Players.LocalPlayer
local Mouse = Player:GetMouse()
UIS.InputBegan:Connect(function(input)
if Mouse.Target then
if Mouse.Target == B6 then
game.StarterGui.MouseOver.Enabled = true
end
end
end)
3 Likes
GetDescendants doesn’t take a parameter. B1 through B6 subsequently all hold the same value, which is an array of the script’s parent’s descendants.
I believe what you meant to do was
script.Parent:FindFirstChild("Button_6", true)
2 Likes
Hi, thanks for the help! I have tried this, but it still doesn’t seem to do anything atm!
1 Like
--Buttons
local B1 = script.Parent.Controls:FindFirstChild("Button_1", true)
local B2 = script.Parent.Controls:FindFirstChild("Button_2", true)
local B3 = script.Parent.Controls:FindFirstChild("Button_3", true)
local B4 = script.Parent.Controls:FindFirstChild("Button_4", true)
local B5 = script.Parent.Controls:FindFirstChild("Button_5", true)
local B6 = script.Parent.Controls:FindFirstChild("Button_6", true)
--Services & Plr
local UIS = game:GetService("UserInputService")
local Player = game.Players.LocalPlayer
local Mouse = Player:GetMouse()
UIS.InputBegan:Connect(function(input)
if Mouse.Target then
if Mouse.Target == B6 then
game.StarterGui.MouseOver.Enabled = true
end
end
end)
Thats what I did, which I assume is what you meant.
1 Like
Ooh I think I know why now.
Mouse.Target is the part in the world space, not in the UI space. You’ll need to change what call you are making. I’ll quickly find out which one you need.
Also, on line 17, you should use Player.PlayerGui instead of game.StarterGui
2 Likes
Actually - I assume Button_1 through Button_6 are actual buttons (TextButtons / ImageButtons). If so, you can use the .MouseEnter and the .MouseLeave events of them in order to detect them
1 Like
Its for these little buttons that I made, the image on them using surface guis and they all use click detectors
1 Like
Also I read that MouseEnter can be buggy, hence why I opted for different
Oh, mouse.target is applicable then.
Try just changing
game.StarterGui.MouseOver.Enabled = true
to
Player.PlayerGui.MouseOver.Enabled = true
1 Like
Still hasn’t worked! I currently have the code as:
--Buttons
local B1 = script.Parent.Controls:FindFirstChild("Button_1", true)
local B2 = script.Parent.Controls:FindFirstChild("Button_2", true)
local B3 = script.Parent.Controls:FindFirstChild("Button_3", true)
local B4 = script.Parent.Controls:FindFirstChild("Button_4", true)
local B5 = script.Parent.Controls:FindFirstChild("Button_5", true)
local B6 = script.Parent.Controls:FindFirstChild("Button_6", true)
--Services & Plr
local UIS = game:GetService("UserInputService")
local Player = game.Players.LocalPlayer
local Mouse = Player:GetMouse()
UIS.InputBegan:Connect(function(input)
if Mouse.Target then
if Mouse.Target == B6 then
Player.PlayerGui.MouseOver.Enabled = true
end
end
end)
Can you please add print statements through the UIS statement, so we can see what is firing?
1 Like
Sure! I wont be a moment! Ty for helping btw
--Buttons
local B1 = script.Parent.Controls:FindFirstChild("Button_1", true)
local B2 = script.Parent.Controls:FindFirstChild("Button_2", true)
local B3 = script.Parent.Controls:FindFirstChild("Button_3", true)
local B4 = script.Parent.Controls:FindFirstChild("Button_4", true)
local B5 = script.Parent.Controls:FindFirstChild("Button_5", true)
local B6 = script.Parent.Controls:FindFirstChild("Button_6", true)
--Services & Plr
local UIS = game:GetService("UserInputService")
local Player = game.Players.LocalPlayer
local Mouse = Player:GetMouse()
UIS.InputBegan:Connect(function(input)
print("connected")
if Mouse.Target then
print("mouse target")
if Mouse.Target == B6 then
print("B6")
Player.PlayerGui.MouseOver.Enabled = true
end
end
end)
Added these but still nothing in output
Where is the script located?
1 Like
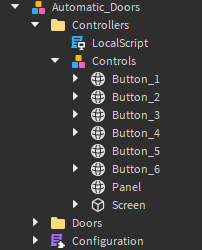
Its the local script in this model, which is in the workspace.
Im still very much learning scripting, so everything is a lesson for me to use in the future!
Try putting it into StarterGui
1 Like
Okay, it now appears to be running and gets to the “Connected” print statement, but still doesn’t show the UI
Sorry, its also printing the mouse target one too
If you have it print out Mouse.Target, then hover over the buttons, what is the output