Essentially, it is always best to try things out, when working with vectors in 2D since they actually are very easy to write into 3D. Finding the corners of a 2D Plane is essentially taking the width and height of the plane and adding and subtracting it from the origin of the plane accordingly. That would probably look something like this:
local Rectangle = {
Position = {X = 20, Y = 20}
Height = 30
Width = 50
}
We will be using a simple object, “Rectangle”, that holds information about where its origin is situated in a 2D cartesian graph and how long its Height and Width are. Now to the interesting part! How about we write a quick and dirty function that takes a Rectangle object as an input and returns its four corners!
local function GetCorners(Rectangle: Rectangle)
local PositionX, PositionY = Rectangle.Position.X, Rectangle.Position.Y
end
Now to actually fetch the 4 corners, we will need to equate how exactly we should do that! Now, as we know, the length of a polygon defines how long a polygon is on the x-axis
and the height defines how high the polygon is on the y-axis
. Assuming the origin of our Rectangle is at the exact centre of itself, we now know that the origin is situated in the exact middle of the x-
and y-axis
.
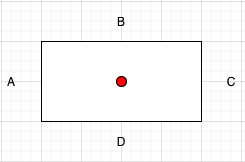
Taking our knowledge about what the width and height of a polygon represent into consideration, and knowing that the origin coordinate is in the exact middle of the width and height, we now know that the origin coordinate is situated exactly at {X = (width / 2), Y = (height / 2)}
. Perfect, now that we know this we are all set! So, what do we want to do if we want to find what x-coordinate
the side A
is on? We would simply have to subtract (going left) the origin’s x-coordinate
by half of the width of the rectangle.
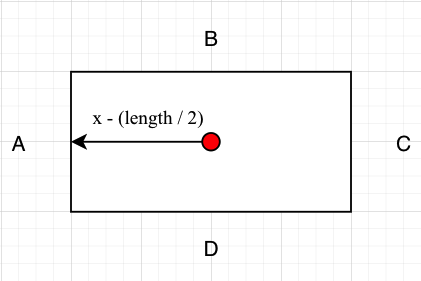
Now, this is not enough to find the corner AB
though, so what would we have to do? We would have to find the corresponding y-coordinate
that B is on. Since we know that the origin y-coordinate
is at height / 2
, we simply have to subtract the origins y-coordinate
by half of the height of the rectangle.
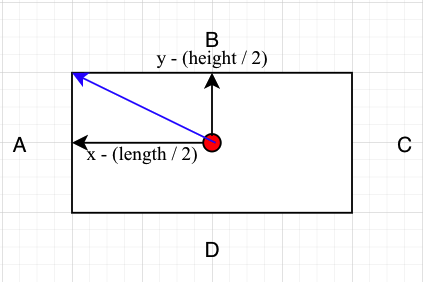
Perfect! Now we have successfully calculated the coordinate at which AB
's corner is situated! Writing this into code shouldn’t be difficult.
local function GetCorners(Rectangle: Rectangle)
local PositionX, PositionY = Rectangle.Position.X, Rectangle.Position.Y
local AB = {X = PositionX - (Rectangle.Width / 2), Y = PositionY - (Rectangle.Height / 2)}
end
Now calculating sides BC
, CD
and AD
only take logic to do, For calculating BC
you would simply have to do the following:
local function GetCorners(Rectangle: Rectangle)
local PositionX, PositionY = Rectangle.Position.X, Rectangle.Position.Y
local AB = {X = PositionX - (Rectangle.Width / 2), Y = PositionY - (Rectangle.Height / 2)}
local BC = {X = PositionX + (Rectangle.Witdh / 2), Y = PositionY - (Rectangle.Height / 2)}
end
At this point, it is pretty self-explanatory and for rewriting this to use 3D Space, you would simply have to add 1 more axis to your calculations. We would have width, height and depth
now which respectively describe its length on the well known x, y, z
axis. I hope this gave you a better understanding at what you are trying to do 
(i know i labeled the arrows as ‘length’, meant to write width there sorry
)