Sorry for late reply.
This script took me like 1 hour to complete.
To make it blackout and blur, Add a instance called ‘Blur’ and ‘Atmosphere’ to Lighting, copy the Atmosphere like this:
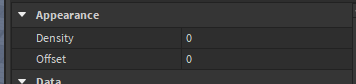
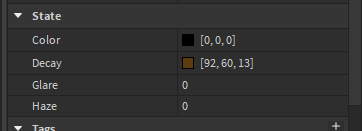
You can change density and offset but it’s optional for your current settings.
Then, add a LocalScript to StarterPlayer > StaterCharacterScripts, then copy and paste into the LocalScript. (Optional) You can rename the LocalScript to ‘Sprint’ or anything
local InputService = game:GetService("UserInputService")
local Lighting = game:GetService("Lighting")
local TweenService = game:GetService("TweenService")
local Players = game:GetService("Players")
local Player = game.Players.LocalPlayer
local Stamina = 100
local Running = false
--Settings
local DefaultAtmosphereDensity = 0.337
local DefaultAtmosphereOffset = 0.25
local DefaultAtmosphereHaze = 0
local DefaultAtmosphereColor = Color3.new(0, 0, 0)
local GainStaminaPower = 1
local DrainStaminaPower = 2
local Cooldown = 0.15
local MaxBlurSize = 12
-----------------------------------------------------
local function killPlayer()
local Atmosphere = Lighting.Atmosphere
local Character = Player.Character
if Character then
local Humanoid = Character:FindFirstChild("Humanoid")
if Humanoid then
Humanoid.Health = 0
wait(Players.RespawnTime)
Atmosphere.Color = DefaultAtmosphereColor
Atmosphere.Density = DefaultAtmosphereDensity
Atmosphere.Haze = DefaultAtmosphereHaze
Atmosphere.Offset = DefaultAtmosphereOffset
end
end
end
local function blackout()
local Atmosphere = Lighting.Atmosphere
local BlackoutTweenInfo = TweenInfo.new(
10,
Enum.EasingStyle.Sine,
Enum.EasingDirection.Out,
0,
false,
0
)
local ColorPropties = {
Density = 1,
Haze = 1,
Offset = 0,
Color = Color3.new(0, 0, 0)
}
local ReverseColorPropties = {
Color = DefaultAtmosphereColor,
Density = DefaultAtmosphereDensity,
Haze = DefaultAtmosphereHaze,
Offset = DefaultAtmosphereOffset
}
local MakeBlackout = TweenService:Create(
Atmosphere,
BlackoutTweenInfo,
ColorPropties
)
local ReverseBlackOut = TweenService:Create(
Atmosphere,
BlackoutTweenInfo,
ReverseColorPropties
)
MakeBlackout.Completed:Connect(killPlayer)
MakeBlackout:Play()
ReverseBlackOut:Cancel()
repeat
task.wait()
until Running == false
MakeBlackout:Cancel()
ReverseBlackOut:Play()
end
local function blurPlayer()
local Blur = Lighting.Blur
spawn(blackout)
repeat
Blur.Size = Blur.Size + 2.5
task.wait()
until Blur.Size >= MaxBlurSize or Running == false
repeat
Blur.Size = Blur.Size - 2.5
task.wait()
until Blur.Size <= 0
end
local function refillStamina()
repeat
Stamina = Stamina + GainStaminaPower
task.wait(Cooldown)
print(Stamina)
until Running == true or Stamina >= 100
end
local function drainStamina()
repeat
Stamina = Stamina - DrainStaminaPower
task.wait(Cooldown)
print(Stamina)
until Running == false or Stamina <= 0
if Stamina <= 0 then
spawn(blurPlayer)
local Character = Player.Character
if Character then
local Humanoid = Character:FindFirstChild("Humanoid")
if Humanoid then
Humanoid.WalkSpeed = 16
end
end
end
end
InputService.InputBegan:Connect(function(input)
if input.KeyCode == Enum.KeyCode.LeftShift then
local Character = Player.Character
if Character then
local Humanoid = Character:FindFirstChild("Humanoid")
if Humanoid then
Running = true
Humanoid.WalkSpeed = 30
spawn(drainStamina)
end
end
end
end)
InputService.InputEnded:Connect(function(input)
if input.KeyCode == Enum.KeyCode.LeftShift then
local Character = Player.Character
if Character then
local Humanoid = Character:FindFirstChild("Humanoid")
if Humanoid then
Running = false
Humanoid.WalkSpeed = 16
spawn(refillStamina)
end
end
end
end)
Make sure in the settings, change the DefaultAtmosphere to your default ones. For Example, if you choose ‘Density’ to 0.365 in Atmosphere, change the ‘DefaultAtmosphereDensity = 0.365’ in the LocalScript.
Please note that this may be inaccurate like the blackout in Apeirophobia.
The player will blackout when they have no stamina when they keep running and they will die, let me know if there’s any problems.
Also do you want it to make a breathing sound inside of a script?