In studio, I can select a model and add it and all of its children to a particular collision group.
When scripting, I have to add each of the parts to the group one at a time using a recursive function.
Is there a way to set a model to a group without going through each part in script?
This isn’t very hard now that I know how to do it, I admit, but it isn’t obvious.
I spent a few hours trying to figure out why my script was working in studio but not in game while I was setting the UpperTorso, LowerTorso, and HumanoidRootPart to the collision groups individually.
I really wanted to make a feature request for this, but I am unable, so perhaps there is a way to do it and I am unaware?
1 Like
No, there is no function to set the collision group of a model. Models don’t have collision groups as they’re just a collection of parts. If there was a model collision group setter it’d be equivalent to this:
for i, v in ipairs(model:GetDescendants())
if v:IsA("BasePart") then
v.CollisionGroupId = id
end
end
3 Likes
Is there any practical reason to use this for players instead?
local PhysicsService = game:GetService("PhysicsService")
local Players = game:GetService("Players")
local playerCollisionGroupName = "Players"
PhysicsService:CreateCollisionGroup(playerCollisionGroupName)
PhysicsService:CollisionGroupSetCollidable(playerCollisionGroupName, playerCollisionGroupName, false)
local function setCollisionGroupRecursive(object)
if object:IsA("BasePart") then
PhysicsService:SetPartCollisionGroup(object, playerCollisionGroupName)
end
for _, child in ipairs(object:GetChildren()) do
setCollisionGroupRecursive(child)
end
end
local function onCharacterAdded(character)
setCollisionGroupRecursive(character)
end
local function onPlayerAdded(player)
player.CharacterAdded:Connect(onCharacterAdded)
end
Players.PlayerAdded:Connect(onPlayerAdded)
This is what I got from Player-Player Collisions but seems redundant.
There actually should be a built in method using the collision group editor.
Simply select the model, open the editor, then click the plus symbol to add the parts within it to a collision group.
1 Like
Well they kinda do the same thing except the method there uses recursion and works for top level base parts. Using GetDescendants
gets every child and every child’s child and so on at every depth. GetDescendants
itself is a recursive function of getting every child then looping over that child’s children which is exactly what that method does. You can even make your own GetDescendants using the exact same method:
function GetDescendants(object, t)
local t = t or {}
for _, child in ipairs(object:GetChildren()) do
table.insert(t, child)
GetDescendants(child, t)
end
return t
end
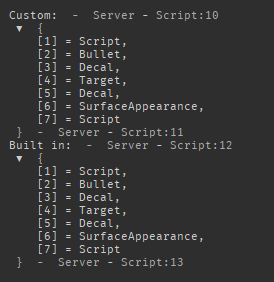
1 Like
You are correct, that is very easy to do and I wish that it was just as easy to do with scripting, but we are required to use SetPartCollisionGroup. That is what makes me think it should be possible with a built-in function instead of having to create our own.