Hello,
I am making a tower defense game and I want the towers to have attack and idle animations. They are already animated but when I try to implement them I believe they override each other. But it only seems to be affecting the arms.
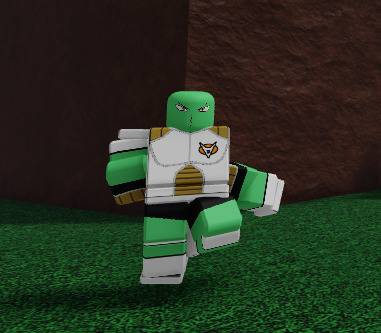
This is the script that tells the tower to play the idle animation
local function setAnimation(object, animName)
local humanoid = object:WaitForChild("Humanoid")
local animationsFolder = object:WaitForChild("Animations")
if humanoid and animationsFolder then
local animationObject = animationsFolder:WaitForChild(animName)
if animationObject then
local animator = humanoid:FindFirstChild("Animator") or Instance.new("Animator", humanoid)
local playingTracks = animator:GetPlayingAnimationTracks()
for i, track in pairs(playingTracks) do
if track.Name == animName then
return track
end
end
local animationTrack = animator:LoadAnimation(animationObject)
return animationTrack
end
end
end
local function playAnimation(object, animName)
local animationTrack = setAnimation(object, animName)
if animationTrack then
animationTrack:Play()
else
warn("Animation track does not exist")
return
end
end
workspace.Towers.ChildAdded:Connect(function(object) -- HERE
playAnimation(object, "Idle")
end)
animateTowerEvent.OnClientEvent:Connect(function(tower, animName, target)
playAnimation(tower, animName)
if target then
if tower.Config:FindFirstChild("Trail") then
fireProjectile(tower, target)
end
tower.HumanoidRootPart.Attack:Play()
end
end)
This is the script that tells the tower to play the attack animation.
function tower.Attack(newTower, player)
local config = newTower.Config
local target = tower.FindTarget(newTower, config.Range.Value, config.TargetMode.Value)
if target and target:FindFirstChild("Humanoid") and target.Humanoid.Health > 0 and target:FindFirstChild("IsHidden") then
if config:FindFirstChild("SeeHidden") then
local targetCFrame = CFrame.lookAt(newTower.HumanoidRootPart.Position, target.HumanoidRootPart.Position)
newTower.HumanoidRootPart.BodyGyro.CFrame = targetCFrame
animateTowerEvent:FireAllClients(newTower, "Attack", target) -- HERE
player.Gold.Value += math.min(config.Damage.Value, target.Humanoid.Health)
target.Humanoid:TakeDamage(config.Damage.Value)
player.Kills.Value += 1
task.wait(config.Cooldown.Value)
end
elseif target and target:FindFirstChild("Humanoid") and target.Humanoid.Health > 0 and not target:FindFirstChild("IsHidden") then
local targetCFrame = CFrame.lookAt(newTower.HumanoidRootPart.Position, target.HumanoidRootPart.Position)
newTower.HumanoidRootPart.BodyGyro.CFrame = targetCFrame
animateTowerEvent:FireAllClients(newTower, "Attack", target) -- HERE
player.Gold.Value += math.min(config.Damage.Value, target.Humanoid.Health)
target.Humanoid:TakeDamage(config.Damage.Value)
player.Kills.Value += 1
task.wait(config.Cooldown.Value)
end
task.wait(0.05)
if newTower and newTower.Parent then
tower.Attack(newTower, player)
end
end
I am unfamiliar with how animations work. Is there perhaps a way to pause the idle animation whilst the tower is attacking and vice versa?
Any help is appreciated