Update 1.3
- Improved CPU performance
-
Any
type now supports tables
-
Any
type now supports EnumItems
- Added
BooleanNumber
type
- Added rate limit to how many events the client can send per server heartbeat [this only effects exploiters]
- Client is no longer framerate dependant and will fire a maximum of 60 events every second
- Bug fixes
here we can see the CPU time it takes to Fire()
an array of 50 booleans and an array of 50 dictionaries with numbers in them
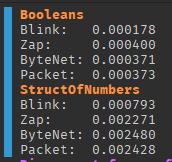
and if you want to run the test for yourself here is the benchmark i used
Benchmark.rbxl (93.3 KB)
its now possible to send tables using the Any
type
-- this will send 22 bytes [1 byte for the packet id, 3 bytes for the table, 11 bytes for "MyCoolKey", 7 bytes for "Hello"]
local packet = Packet("Name", Packet.Any)
packet:Fire({MyCoolKey = "Hello"})
-- this will send 7 bytes [1 byte for the packet id, 6 bytes for "Hello"]
local packet = Packet("Name", {MyCoolKey = Packet.String})
packet:Fire({MyCoolKey = "Hello"})
as you can see sending tables with the Any
type using a lot more data compared to predefining your tables so its always recommended to predefine your types to get the best performance
one benefit to using the Any
type for tables is you can use Any
type for the key for example
local packet = Packet("Name", Packet.Any)
packet:Fire({[Vector3.new(1, 2, 3)] = Enum.Material.Grass})
packet:Fire({[{Key1 = {1, 4, 7}, Key2 = "Test"}] = false})
you can now send EnumItems
with the any type
local packet1 = Packet("Name1", Packet.Any)
packet:Fire(Enum.Material.Grass)
this will send 4 bytes for the EnumItem
vs 3 if we had defined it
the BooleanNumber
type lets you send 1 boolean and 1 number for just 1 byte
local packet1 = Packet("Name1", Packet.BooleanNumber)
packet:Fire({Boolean = true, Number = 54})
the number only has 7 bits so has a range between 0 - 127
the client is expected to fire at most 60 events every second 1 per server heartbeat
if exploiters sends more then 10 events per heartbeat the server will now ignore them
this limit can be modified by editing this line in the main module
local bytes = (playerBytes[player] or 0) + math.max(buffer.len(receivedBuffer), 800)
if bytes > 8_000 then
if RunService:IsStudio() then
warn(player.Name, "is exceeding the data/rate limit; some events may be dropped")
end
return
end
here we can see there is a limit of 8000 bytes [8KB] per heartbeat
and we can see that a event costs a minimum of 800 bytes even if the size is less
8000 / 800 = 10 events per heartbeat
the reason we must except more then 1 event per heartbeat is because ping can fluctuate and some events might arrive on the same frame normally this will be around 2-3 events per frame but to give some extra headroom I have set it to a max of 10 if you feel that this is not enough you can modify the 800 to something like 400 to allow 20 events per heartbeat
also remember that this limit is only from Client > Server
there are no limits going from Server > Client
if you find any bugs please report them and ill do my best to fix them