I want to achieve a Npc that pathfinds around walls and chase after players when seen moving around. When the player stops moving around the npc stops chasing after the player and goes back to pathfinding. The pathfinding parts is in my workspace in a folder named PathfindingParts. The parts are labeled 1-9. The issue here is that the code does not work but no errors in the output either. I searched about this but haven’t found a solution for this yet.
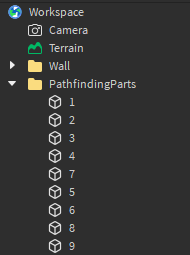
Here’s the code:
local PathfindingService = game:GetService("PathfindingService")
local Players = game:GetService("Players")
local RunService = game:GetService("RunService")
local PathfindingFolder = workspace.PathfindingParts
local NPC = workspace.PathfindingNPC
local Player = nil
local Path = nil
local CurrentWaypoint = nil
local function onPlayerAdded(player)
Player = player
end
Players.PlayerAdded:Connect(onPlayerAdded)
function getNextWaypoint()
if CurrentWaypoint == nil or CurrentWaypoint.Parent == nil then
CurrentWaypoint = PathfindingFolder:GetChildren()[1]
else
local index = table.find(PathfindingFolder:GetChildren(), CurrentWaypoint)
if index ~= nil and index < #PathfindingFolder:GetChildren() then
CurrentWaypoint = PathfindingFolder:GetChildren()[index+1]
else
CurrentWaypoint = PathfindingFolder:GetChildren()[1]
end
end
return CurrentWaypoint.Position
end
function followPlayer()
if Player == nil or Player.Parent == nil then
return
end
local playerPosition = Player.Character and Player.Character.HumanoidRootPart.Position or nil
if playerPosition == nil then
return
end
local distance = (NPC.HumanoidRootPart.Position - playerPosition).Magnitude
if distance <= 10 then
NPC.Humanoid:TakeDamage(15)
end
if distance > 15 then
Path = nil
return
end
if Path == nil then
Path = PathfindingService:CreatePath()
Path:ComputeAsync(NPC.HumanoidRootPart.Position, playerPosition)
CurrentWaypoint = nil
end
local waypoints = Path:GetWaypoints()
if #waypoints == 0 then
return
end
local nextWaypoint = waypoints[1]
if nextWaypoint.Action == Enum.PathWaypointAction.Jump then
NPC.Humanoid.Jump = true
end
if nextWaypoint.Action == Enum.PathWaypointAction.MoveTo then
NPC.Humanoid:MoveTo(Vector3.new(nextWaypoint.Position.X, NPC.HumanoidRootPart.Position.Y, nextWaypoint.Position.Z))
end
if nextWaypoint.Action == Enum.PathWaypointAction.Finish then
Path = nil
end
end
RunService.Heartbeat:Connect(function()
if Player == nil or Player.Parent == nil then
followPlayer = nil
getNextWaypoint()
elseif Player.Character ~= nil and Player.Character.HumanoidRootPart ~= nil then
if followPlayer ~= nil then -- add a check to make sure followPlayer is not nil before calling it
followPlayer()
end
else
getNextWaypoint()
end
end)