I made a function that fills in parts just by saying where point A and point B is. Downside is that it has to be able to divide by its own Z, but here you go.
WARNING:
DO NOT USE THIS FOR GAMES, THIS IS JUST A THING I MADE FOR OTHERS TO LEARN FROM
Example
Before
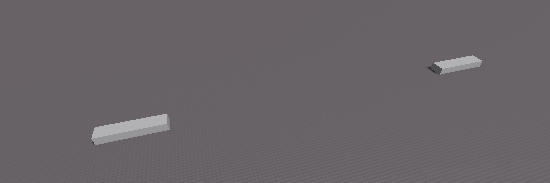
After
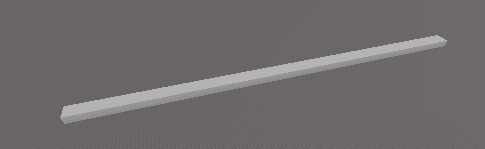
Code
function calculate(a,b)
local lastPosition = a.Position
for i=1,(b.Position.Z-b.Size.Z)/b.Size.Z do
print(i)
local part = Instance.new("Part",workspace)
part.Position = lastPosition + Vector3.new(0,0,a.Size.Z)
part.Size = a.Size
part.Anchored = true
lastPosition = part.Position
end
end
calculate(workspace.A,workspace.B)
3 Likes
what do you mean by that? i don’t understand
What if you have two different sized parts, at different angles from each other?
part.Size = a.Size
This won’t correctly fill in the gap, as gapfill would. I suggest checking out the plugin source code, to find a way to resolve this issue.
It was made in a few minutes and hasn’t been improved, there’s a problem where it’ll only work on even numbers.
1 Like
I made an improved version, instead of doing multiple parts, it does one part that creates a part in between pointA and pointB.
Code
function fillGap(pointA,pointB)
local part = Instance.new("Part")
part.Size = Vector3.new(pointA.Size.X,pointA.Size.Y,(pointB.Position.Z-pointB.Size.Z))
part.Position = Vector3.new(pointA.Position.X,pointA.Position.Y,(pointB.Position.Z/pointB.Size.Z))
part.Anchored = true
part.Parent = workspace
end
fillGap(workspace.A,workspace.B)