So let’s say I have this setup
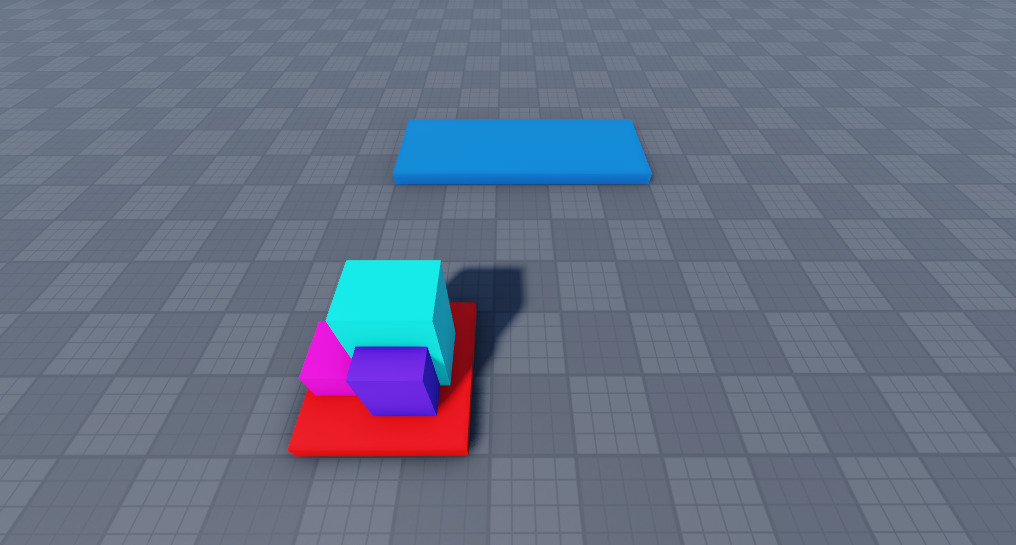
The multiple parts a model. I want each individual to be positioned on top of the blue part, but maintain their relative positions.
local Blue = workspace.Blue
local Model = workspace.Model
for i = 1, 4 do
local Part = Model[i]
Part:PivotTo(Blue.CFrame)
task.wait(2)
end
So when the model has been moved over, the model should look the exact same
local Blue = workspace.Blue
local Model = workspace.Model
local LastPartCFrame, LastPartPos
for i = 1, 4 do
local Part = Model[i]
If LastPartSize == nil then
Part:PivotTo(Blue.CFrame) + Vector3.new(0,Blue.Position.Y,0)
Else
Part:PivotTo(LastPartCFrame) + Vector3.new(0,LastPartPos/2,0)
end
LastPartSize = Model[i].CFrame
LastPartPos = Model[i].Position.Y
task.wait(2)
end
No clue if this will work so let me know. Just edited to make it work.
Without trying, but just by looking, this needs to work if each colored part were say a seperate model with multiple parts in it. So it can’t just work on a single part basis
sorry should have mentioned that in original post
Just make it the primary part. And weld.
It also doesn’t work anyway 
Not sure if this is what your looking for but i would say save cframe offsets of that red part to each and every part by using .CFrame:Inverse()
-- for each part in that model
-- part is the current part
-- red part is, well, the red part
-- best to save in a table for access
local offset = part.CFrame:Inverse() * redPart.CFrame
Then you can cframe the red part to where you want, and loop through all cframe offsets and set the cframe based off of the cframe of the red parts and the offsets.
-- for each part
part.CFrame = redPart.CFrame * the_cframe_offset
Or maybe this is just too complicated and your looking for something related to :PivotTo
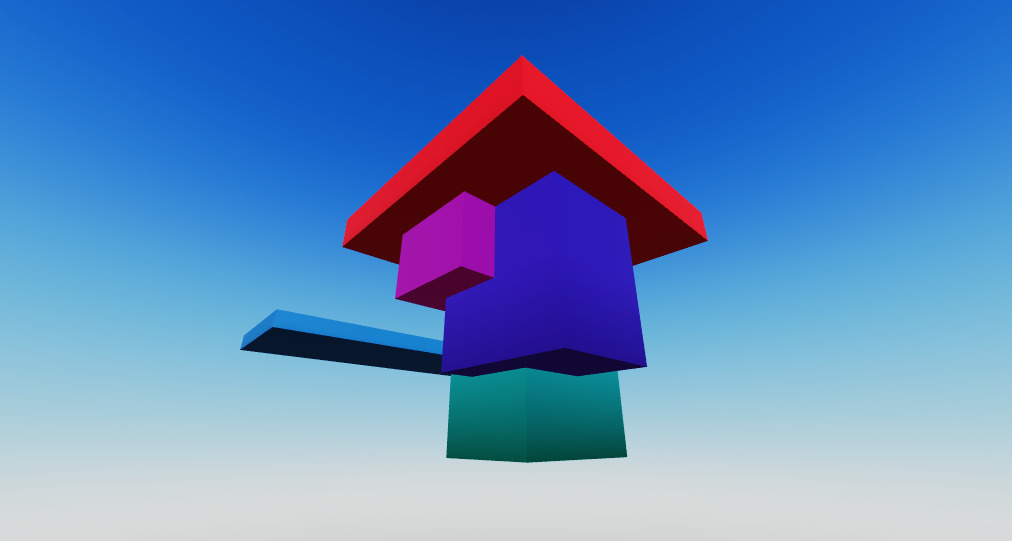
Seemed to just position them under the red part. There’s no reference to the blue part there either
Did you cframe the red part first? Also I think for the cframe offset code it was supposed to be
local offset = redPart.CFrame:Inverse() * part.CFrame
That still didn’t work unfortunately 
local Blue = workspace.Blue
local Model = workspace.Model
local LastPartCFrame, LastPartSize = nil
for _, v in Model:GetChildren() do -- Iterate over the model, you can change this to :GetDescendants() if you want.
if v:IsA("BasePart") then -- Make sure its a part and not a script or anyother object
local Part = v -- Set the part
if LastPartSize ~= nil and LastPartCFrame ~= nil then -- Check if there was a last part
Part.CFrame = LastPartCFrame + Vector3.new(0,LastPartSize ,0)
else
Part.CFrame = Blue.CFrame + Vector3.new(0,Blue.Size.Y ,0)
end
LastPartCFrame = Part.CFrame -- Set the last part CFrame to the parts CFrame
LastPartSize = Part.Size.Y -- Set LastPartSize to parts size
task.wait(2)
end
end
I have tried this and it worked. If it doesn’t let me know.