Sometimes we need to assign a unique ID to certain entities and we use this way as the simplest way:
local Table = {}
local Part1 = Instance.new("Part")
Part1.Parent = workspace
Part1.Name = "Part1"
table.insert(Table,Part1)
local Part1Id = table.find(Table,Part1)
A unique ID has been assigned for this entity.
because tables cannot have more than one entity with the same key
If we try to pull that entity from the table using this unique id, we can reach that object.
However, if an entity in the table is deleted, all entities after that entity are shifted by 1 line and the keys become meaningless.
as in this example:
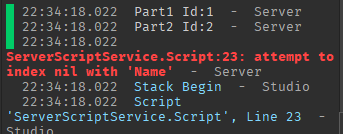
local Table = {}
local Part1 = Instance.new("Part")
Part1.Parent = workspace
Part1.Name = "Part1"
table.insert(Table,Part1)
local Part1Id = table.find(Table,Part1)
local Part2 = Instance.new("Part")
Part2.Parent = workspace
Part2.Name = "Part2"
table.insert(Table,Part2)
local Part2Id = table.find(Table,Part2)
print(string.format("Part1 Id:%d",Part1Id))
print(string.format("Part2 Id:%d",Part2Id))
Part1:Destroy()
table.remove(Table,Part1Id)
print(string.format("Part2:",Table[Part2Id].Name))
And I have developed a library as a solution to this.
local KeyGenerator = require(script.Parent.KeyGenerator)
local Ukey1 = KeyGenerator:GenerateGlobalKey()
-- Returns a unique random key
task.wait(1)
KeyGenerator:RemoveKey(Ukey1)
-- frees up space in the system by deleting dead or unnecessary outlines.
One more example:

local KeyGenerator = require(script.Parent.KeyGenerator)
for i = 1,500 do
task.spawn(function()
print(KeyGenerator:GenerateGlobalKey())
end)
end
KeyGenerator Module (1.2 KB)
What are you talking about? No you didn’t. The key now is 1 and the value is Part1. That’s what table.insert()
does. And then you’re using table.find()
to iterate through the list and check every single element if it is Part1.
find - Variant
Within the given array-like table haystack, find the first occurrence of value needle, starting from index init or the beginning if not provided. If the value is not found, nil is returned.
A linear search algorithm is performed.
How to actually set a part as a key and make a UUID for it
local UUIDS = {}
local Part1 = Instance.new("Part")
Part1.Parent = workspace
Part1.Name = "Part1"
-- add part
UUIDS[Part1] = game.HttpService:GenerateGUID()
-- remove it from the table
UUIDS[Part1] = nil
But in general, you do not need this. After all, now you’d still need to check if the UUID is the same. You may as well just check if the part is the same in the first place. Roblox lets you do that even across the Client-Server boundary out of the box.
1 Like
I’m not sure how this proves useful. It seems obsolete compared to HttpService:GenerateGUID()
.
Sure, there’s less characters but the sheer expense of searching and creating each byte in the ID outweighs the cost.
1 Like
It makes sense to create UUIDs, but they are unnecessarily long and not very good for speed.
Usually that doesn’t really matter. The speed difference is negligible.
If it really does to you, just do it like this.
local UUID = 0
local function generateUUID() UUID+=1; return UUID end
local UUIDS = {}
local Part1 = Instance.new("Part")
Part1.Parent = workspace
Part1.Name = "Part1"
-- add part
UUIDS[Part1] = generateUUID()
-- remove it from the table
UUIDS[Part1] = nil
Although I still don’t understand your use-case for this.