Hello!
I’ve currently got 6 posters in game. They’ve got decals on them and they’re on the wall. I’m trying to get every single player in the servers’ photo / headshot on the posters. Currently what I’ve attempted was looping through the player list and posters and putting the images like that. The only problem with this is that it only grabs one player and puts their photo on every single poster.
Image of files:
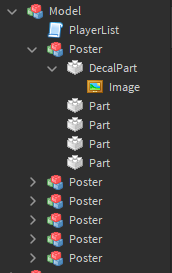
How would I go about making sure each player has a photo, and removing and adding a new one each time a player leaves or joins? If it’s of any interest, max player count is 6 and there’s 6 posters.
Since you have 6 posters and a 6 player max server then the code should be easy:
for i, v in pairs(game.Players:GetChildren()) do
local Poster = nil
for e, r in pairs(Model:GetChildren()) do
if r.Name == "Poster" and r.DecalPart.Image.ImageId == "" then
Poster = r
end
end
-- Put the character's image in Poster.DecalPart.Image
end
2 Likes
Thank you! Works perfectly paired with player.added and player.removing; I figured it was an easy method I was just overlooking it.
1 Like
This is a script I have quickly made to achieve your goal.
I suggest you name the poster models from 1-6 (eg: “Poster1”, “Poster2”, etc) for my solution to work
--Services
local Players = game:GetService("Players")
--Configs (Change to your liking)
local ThumbnailSize = Enum.ThumbnailSize.Size420x420
local ThumbnailType = Enum.ThumbnailType.HeadShot
--Storage variables
local PlayerList = {}
--Object refs
local PostersContainer = script.Parent --Change to the path of the model containing the poster models
local PosterAmount = 6 --The amount of poster models you have
local PosterPrefix = "Poster" --The prefix for each poster
local DecalPartName = "DecalPart" --The name of the decal part
local DecalName = "Image" --The name of the decal
--Event/functions
local function UpdatePosters() --Handles the update of all the poster
for i = 1,PosterAmount do
local Player = PlayerList[i]
if not Player then return end
local Poster = PostersContainer:FindFirstChild("Poster"..i)
if not Poster then continue end
local Decal = Poster[DecalPartName][DecalName]
Decal.Texture = Player.Image --If this is a image label/button, you can change "Texture" to "Image"
end
end
local function OnPlayerAdded(plr:Player) --Handles a player being added to the game
local Image = Players:GetUserThumbnailAsync(plr.UserId, ThumbnailType, ThumbnailSize)
local PlayerTable = {
Name = plr.Name,
Image = Image
}
table.insert(PlayerList, PlayerTable)
UpdatePosters()
end
local function OnPlayerRemoving(plr:Player) --Handles a player leaving the game
local Index = nil
for i,v in ipairs(PlayerList) do --Loops through the table that stores the Images to removing the player that left
if v.Name == plr.Name then
Index = i
break
end
end
if Index then
table.remove(PlayerList, Index)
UpdatePosters()
end
end
--Connecting the events
Players.PlayerAdded:Connect(OnPlayerAdded)
Players.PlayerRemoving:Connect(OnPlayerRemoving)
I know there’s already a solution but I started putting this together before they did so I thought I’d at least finish it so you have more than one option.
1 Like