local RunService = game:GetService'RunService'
local Players = game:GetService'Players'
local LocalPlayer = Players.LocalPlayer
local Character = LocalPlayer.Character or LocalPlayer.CharacterAdded:Wait()
local Humanoid = Character:FindFirstChildOfClass'Humanoid'
local RootPart = Character:WaitForChild'HumanoidRootPart'
local RootJoint = RootPart:WaitForChild'RootJoint'
local RootC0 = RootJoint.C0
local MaxTiltAngle = 10
local Tilt = CFrame.new()
RunService.RenderStepped:Connect(function(Delta)
local MoveDirection = RootPart.CFrame:VectorToObjectSpace(Humanoid.MoveDirection)
Tilt = Tilt:Lerp(CFrame.Angles(math.rad(-MoveDirection.Z) * MaxTiltAngle, math.rad(-MoveDirection.X) * MaxTiltAngle, 0), 0.2 ^ (1 / (Delta * 60)))
RootJoint.C0 = RootC0 * Tilt
end)
So I saw this in another topic and I was just mainly confused as to why math.rad(-MoveDirection.X) * MaxTiltAngle
is what causes the leaning to the left or right of the character when moving in the negative or positive x direction. Shouldn’t the character sort of turn left or right because it’s the y value? help 
1 Like
Hi there!
I understand your confusion so let’s break it down:
-
Understanding MoveDirection
:
-
Humanoid.MoveDirection
is a normalized vector that indicates the direction the character is trying to move. Its components (X
, Y
, Z
) represent movement in the local space of the character:
-
X: Forward/backward (positive forward, negative backward).
-
Y: Upward/downward (typically not used for leaning).
-
Z: Left/right (positive to the right, negative to the left).
-
Why math.rad(-MoveDirection.X) * MaxTiltAngle
?
- In the context of the character’s local movement:
-
Negative X (moving backward) causes a tilt backward, which makes sense.
-
Positive X (moving forward) keeps the character upright.
- The
MoveDirection.Z
value influences the tilt left or right. However, in this case, math.rad(-MoveDirection.X)
is used to control the tilt based on forward/backward movement, which might be an intentional design choice.
-
Tilt Adjustments:
- The script uses
Tilt:Lerp(...)
for smooth transitions, helping to avoid abrupt changes in the character’s orientation.
- The leaning effect occurs mainly through the X direction because it’s representing the forward motion.
-
Suggested Changes for Clarity:
- If you want the character to lean left or right based on the Z component of
MoveDirection
, you can modify the line like this:Tilt = Tilt:Lerp(CFrame.Angles(math.rad(-MoveDirection.Z) * MaxTiltAngle, math.rad(-MoveDirection.X) * MaxTiltAngle, 0), 0.2 ^ (1 / (Delta * 60)))
- This way, positive Z values would tilt the character to the right, while negative values tilt to the left.
Heres a quick diagram which shows you the x, y and z axis.
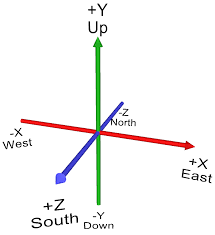
I hope this helps clarify.
1 Like