Hello there! I’m making a spleef game and I need to make a really good hitbox for them. I am not going to use Touched for it, since I have more than 100 spleefs. I am currently using raycasting to detect whenever a character intersects with it. It doesn’t seem to work properly, here are some images of people using a glitch to win every round. Here:
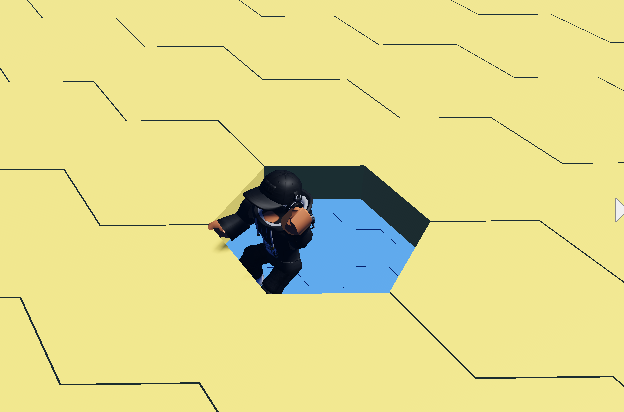
As you can see, they are standing still and nothing is happening to the spleefs.
Here’s the raycasting code I am using:
function Round:Raycast(character)
if (not character) then return end;
local params = RaycastParams.new()
params.FilterType = Enum.RaycastFilterType.Whitelist
local whiteList = {}
for _, spleef in pairs(workspace.Spleef:GetDescendants()) do
if spleef:IsA("BasePart") and spleef.Name == "Hexagon" then
if spleef.Sinking.Value then
continue;
end
table.insert(whiteList,spleef)
end
end
local leftFoot = character:FindFirstChild("LeftFoot")
local rightFoot = character:FindFirstChild("RightFoot")
local human = character:FindFirstChildOfClass("Humanoid")
if (not human) then return end
if (not leftFoot) then return end
if (not rightFoot) then return end
if (not human.RootPart) then return end
if (human.RootPart) then return end;
params.FilterDescendantsInstances = whiteList
local rayCast = workspace:Raycast(leftFoot.Position,Vector3.new(0,-5,0),params);
if (not rayCast) then
rayCast = workspace:Raycast(rightFoot.Position,Vector3.new(0,-5,0),params);
end
if (not rayCast) then
rayCast = workspace:Raycast(human.RootPart.Position,human.RootPart.CFrame.LookVector*3,params);
end
if (not rayCast) then return end
local instance = rayCast.Instance
if (not instance:IsDescendantOf(workspace.Spleef)) then return end
self:DisappearSpleef(instance)
end
Help is appreciated 
None of those look like glitches to me. The position of body parts is based off of the center point of them. On the first one, the center point of the player’s legs are over the hole, in the second one, their legs aren’t on the platform at all and they aren’t looking at the platform directly either.
In my opinion, your best bet would be that if all of your raycasts return nil, loop over the player’s body parts with GetTouchingParts()
and see if any platform is being touched, and if so, destroy it. Because this is a last resort, it isn’t run that often and isn’t too much of a performance hog.
3 Likes
Thanks for replying to me, it’s really appreciated 
I will do that right now. Thank you so much
Doesn’t seem to work at all. Sometimes it does work, but sometimes it doesn’t detect the spleef: Code:
function Round:Raycast(character)
if (not character) then return end;
local params = RaycastParams.new()
params.FilterType = Enum.RaycastFilterType.Whitelist
local whiteList = {}
for _, spleef in pairs(workspace.Spleef:GetDescendants()) do
if spleef:IsA("BasePart") and spleef.Name == "Hexagon" then
if spleef.Sinking.Value then
continue;
end
table.insert(whiteList,spleef)
end
end
local leftFoot = character:FindFirstChild("LeftFoot")
local rightFoot = character:FindFirstChild("RightFoot")
local human = character:FindFirstChildOfClass("Humanoid")
if (not human) then return end
if (not leftFoot) then return end
if (not rightFoot) then return end
if (leftFoot and not (leftFoot.Parent)) then return end
if (rightFoot and not (rightFoot.Parent)) then return end
if (not human.RootPart) then return end
if (human.RootPart) and (not (human.RootPart.Parent)) then return end
params.FilterDescendantsInstances = whiteList
local rayCast = workspace:Raycast(leftFoot.Position,Vector3.new(0,-5,0),params);
if (not rayCast) then
rayCast = workspace:Raycast(rightFoot.Position,Vector3.new(0,-5,0),params);
end
if (not rayCast) then
rayCast = workspace:Raycast(human.RootPart.Position,human.RootPart.CFrame.LookVector*3,params);
end
if (not rayCast) then
for _, bodyPart in pairs(character:GetDescendants()) do
if bodyPart:IsA("BasePart") then
for _, touchingPart in pairs(bodyPart:GetTouchingParts()) do
if touchingPart:IsDescendantOf(workspace.Spleef) then
warn("removing")
self:DisappearSpleef(touchingPart)
break
end
end
end
end
return
end
local instance = rayCast.Instance
if (not instance:IsDescendantOf(workspace.Spleef)) then return end
self:DisappearSpleef(instance)
end
Can anyone help me, it’s been an hour, thank you