no, its when an aura is skipped, as i told you before, then it stops working.
As for the if statement, i think its the first one, the one that has the “Equip:” result shows twice in the output
ill show you:
- first i rolled a common
- i skipped this common
- then i rolled an uncommon
- i equipped this uncommon
- the auras that got inside of my inventory where both uncommon and common in that order:
(when you skip an aura, that aura doesnt get printed into the output)
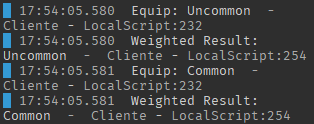
this is the full script
local rp = game:GetService("ReplicatedStorage")
local ss = game:GetService("SoundService")
local equipEvent = rp.EquipAura
local rollEvent = rp.Roll
local mainEvent = rp.MainEvent
local button = script.Parent
local Auras = require(rp:WaitForChild("Auras"))
local invFrame = script.Parent.Parent.Parent:WaitForChild("Tabs").Inventory:WaitForChild("ScrollingFrame")
local collectionFrame = script.Parent.Parent.Parent:WaitForChild("Tabs").Collection:WaitForChild("ScrollingFrame")
local exampleBtn = invFrame:WaitForChild("Example")
local db = false
local specialChance = 1000
local cutsceneChance = 10000
local function auraTick(nameTxt : TextLabel, chanceTxt : TextLabel)
local selectedAura = math.random(1, #Auras)
local aura = Auras[selectedAura]
nameTxt.Text = tostring(aura.name)
nameTxt.Position = UDim2.fromScale(.5, .46)
nameTxt.TextColor3 = aura.color
nameTxt.FontFace = aura.font
game.TweenService:Create(nameTxt, TweenInfo.new(.5, Enum.EasingStyle.Sine, Enum.EasingDirection.Out), { Position = UDim2.fromScale(.5, .48)}):Play()
chanceTxt.Text = "1 in " .. tostring(aura.chance)
chanceTxt.Position = UDim2.fromScale(.5, .515)
chanceTxt.TextColor3 = aura.color
chanceTxt.FontFace = aura.font
game.TweenService:Create(chanceTxt, TweenInfo.new(.5, Enum.EasingStyle.Sine, Enum.EasingDirection.Out), { Position = UDim2.fromScale(.5, .535)}):Play()
ss:WaitForChild("Tick"):Play()
end
button.MouseButton1Click:Connect(function()
if db == false and game.Players.LocalPlayer:GetAttribute("AutoRoll") == false then
db = true
rollEvent:FireServer()
end
end)
rollEvent.OnClientEvent:Connect(function(result, chance, color, font)
local blackFrame = script.Parent.Parent.Frame
local star = blackFrame.Star
local vignette = blackFrame.Vignette
local nameTxt = blackFrame.AuraName
local chanceTxt = blackFrame.AuraChance
local skipButton = blackFrame.SkipAura
local equipButton = blackFrame.EquipAura
local skipClicked = false
local equipClicked = false
local cooldown = game.Players.LocalPlayer:GetAttribute("Cooldown")
blackFrame.Visible = true
auraTick(blackFrame.AuraName, blackFrame.AuraChance)
task.wait(cooldown - 0.25)
auraTick(blackFrame.AuraName, blackFrame.AuraChance)
task.wait(cooldown - 0.25)
auraTick(blackFrame.AuraName, blackFrame.AuraChance)
task.wait(cooldown - 0.25)
auraTick(blackFrame.AuraName, blackFrame.AuraChance)
task.wait(cooldown - 0.25)
nameTxt.Text = tostring(result)
nameTxt.Position = UDim2.fromScale(.5, .46)
nameTxt.TextColor3 = color
nameTxt.FontFace = font
game.TweenService:Create(nameTxt, TweenInfo.new(.5, Enum.EasingStyle.Sine, Enum.EasingDirection.Out), { Position = UDim2.fromScale(.5, .48)}):Play()
chanceTxt.Text = "1 in " .. tostring(chance)
chanceTxt.Position = UDim2.fromScale(.5, .515)
chanceTxt.TextColor3 = color
chanceTxt.FontFace = font
game.TweenService:Create(chanceTxt, TweenInfo.new(.5, Enum.EasingStyle.Sine, Enum.EasingDirection.Out), { Position = UDim2.fromScale(.5, .535)}):Play()
ss:WaitForChild("Tick"):Play()
task.wait(cooldown - 0.25)
if chance >= specialChance and chance < cutsceneChance then
blackFrame.Transparency = 0
vignette.Visible = true
vignette.ImageTransparency = 1
vignette.ImageColor3 = color
game:GetService("TweenService"):Create(vignette, TweenInfo.new(4, Enum.EasingStyle.Sine, Enum.EasingDirection.In), { ImageTransparency = 0 }):Play()
task.wait(4)
ss:WaitForChild("NormalBoom"):Play()
blackFrame.Transparency = 0.45
vignette.ImageColor3 = Color3.fromRGB(255, 255, 255)
vignette.ImageTransparency = 1
elseif chance >= cutsceneChance then
ss:WaitForChild("Shine"):Play()
blackFrame.Transparency = 0
star.Visible = true
vignette.Visible = true
star.ImageColor3 = color
vignette.ImageColor3 = color
vignette.ImageTransparency = 0
star:TweenSize(UDim2.fromScale(0.5, 0.5), Enum.EasingDirection.Out, Enum.EasingStyle.Sine, 4)
game:GetService("TweenService"):Create(star, TweenInfo.new(8, Enum.EasingStyle.Sine, Enum.EasingDirection.InOut), { Rotation = math.random(360, 360*1.5) }):Play()
task.wait(4)
star:TweenSize(UDim2.fromScale(1, 1), Enum.EasingDirection.In, Enum.EasingStyle.Sine, 4)
task.wait(4)
ss:WaitForChild("SpecialBoom"):Play()
blackFrame.Transparency = 0.45
vignette.Visible = false
vignette.ImageColor3 = Color3.fromRGB(255, 255, 255)
vignette.ImageTransparency = 1
star.Visible = false
star.Size = UDim2.fromScale(1, 1)
star.Rotation = 0
star.ImageColor3 = Color3.fromRGB(255, 255, 255)
end
if tonumber(game.Players.LocalPlayer:GetAttribute("AutoSkip")) < chance then
if (tonumber(game.Players.LocalPlayer:GetAttribute("AutoEquip")) > chance) or (game.Players.LocalPlayer.Auras.Value == game.Players.LocalPlayer.Max.Value) then
skipButton.Visible = true
equipButton.Visible = true
elseif tonumber(game.Players.LocalPlayer:GetAttribute("AutoEquip")) < chance and game.Players.LocalPlayer.Auras.Value ~= game.Players.LocalPlayer.Max.Value then
print("Equip: " .. tostring(result))
equipEvent:FireServer(result)
mainEvent:FireServer(1, "aurasValue")
-- create a button
local btn = exampleBtn:Clone()
btn.Parent = invFrame
btn.Visible = true
btn.Text = tostring(result)
btn.TextColor3 = color
btn.UIStroke.Color = color
btn.FontFace = font
btn.Chance.Value = chance
local auraBtn : TextButton = collectionFrame[tostring(result)]
auraBtn.Locked.Value = false
auraBtn.Text = tostring(result)
auraBtn.TextColor3 = color
auraBtn.FontFace = font
auraBtn.Interactable = true
print("Weighted Result: " .. result)
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
end
else
print("Weighted Result: " .. result)
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
end
local equippedAnAura = false
local equipWarned = false
local showedEquipWarning = false
skipButton.MouseButton1Click:Connect(function()
local warned = false
if chance > specialChance then
blackFrame.Warning.Visible = true
warned = true
end
if warned == true then
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
else
print("Skipped: " .. tostring(result))
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
end
end)
equipButton.MouseButton1Click:Connect(function()
if game.Players.LocalPlayer.Auras.Value ~= game.Players.LocalPlayer.Max.Value and equippedAnAura == false then
equippedAnAura = true
print("Equip: " .. tostring(result))
equipEvent:FireServer(result)
mainEvent:FireServer(1, "aurasValue")
-- create a button
local btn = exampleBtn:Clone()
btn.Parent = invFrame
btn.Visible = true
btn.Text = tostring(result)
btn.TextColor3 = color
btn.UIStroke.Color = color
btn.FontFace = font
btn.Chance.Value = chance
local auraBtn : TextButton = collectionFrame[tostring(result)]
auraBtn.Locked.Value = false
auraBtn.Text = tostring(result)
auraBtn.TextColor3 = color
auraBtn.FontFace = font
auraBtn.Interactable = true
print("Weighted Result: " .. result)
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
blackFrame.Warning.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
elseif game.Players.LocalPlayer.Auras.Value == game.Players.LocalPlayer.Max.Value and equipWarned == false then
equipWarned = true
elseif equipWarned == true then
blackFrame.Warning.Visible = true
blackFrame.Warning.Text = "Not enough storage! (if you click equip, a random aura from your inventory will get deleted)"
showedEquipWarning = true
elseif game.Players.LocalPlayer.Auras.Value == game.Players.LocalPlayer.Max.Value and showedEquipWarning == true then
for _, child in pairs(invFrame:GetChildren()) do
if child:IsA("TextButton") then
child:Destroy()
mainEvent:FireServer(-1, "aurasValue")
break
end
end
print("Equip: " .. tostring(result))
equipEvent:FireServer(result)
-- create a button
local btn = exampleBtn:Clone()
btn.Parent = invFrame
btn.Visible = true
btn.Text = tostring(result)
btn.TextColor3 = color
btn.UIStroke.Color = color
btn.FontFace = font
btn.Chance.Value = chance
local auraBtn : TextButton = collectionFrame[tostring(result)]
auraBtn.Locked.Value = false
auraBtn.Text = tostring(result)
auraBtn.TextColor3 = color
auraBtn.FontFace = font
auraBtn.Interactable = true
print("Weighted Result: " .. result)
mainEvent:FireServer(1, "roll")
blackFrame.Visible = false
skipButton.Visible = false
equipButton.Visible = false
script.Parent.Frame.Transparency = 0.45
script.Parent.Frame.Size = UDim2.fromScale(1, 1)
game.TweenService:Create(script.Parent.Frame, TweenInfo.new(cooldown), { Transparency = 1, Size = UDim2.fromScale(0, 1) }):Play()
task.wait(cooldown)
db = false
end
end)
end)