Hey, I’m currently trying to make a scrollingframe which should hold the player’s accessories, and I was wondering why it was not putting the accessory template for the accessories in the scrollingframe, so i added prints, and i realised that those did not even print anything. theres nothing related in the output aswell, this is my script:
local player = game.Players.LocalPlayer
local template = script.Parent.ItemTemplate
local scrollingFrame = script.Parent.ScrollingFrame
-- String split function
local function splitString(inputstr, sep)
sep = sep or "%s"
local t = {}
for str in string.gmatch(inputstr, "([^"..sep.."]+)") do
table.insert(t, str)
end
return t
end
-- Function to clone and populate the accessories
local function PopulateAccessories()
-- Get Humanoid
local humanoid = player.Character:FindFirstChildOfClass("Humanoid")
if not humanoid then
return -- Return if Humanoid is not found
end
-- Get HumanoidDescription
local humanoidDescription = humanoid:GetAppliedDescription()
print("HatAccessory:", humanoidDescription.HatAccessory)
-- Get hat accessory IDs (assuming they are comma-separated)
local hatAccessoryIds = {}
if humanoidDescription.HatAccessory ~= "" then
hatAccessoryIds = splitString(humanoidDescription.HatAccessory, ",")
end
-- Clone template for each hat accessory
for _, accessoryId in ipairs(hatAccessoryIds) do
print("Hat ID:", accessoryId)
local clone = template:Clone()
clone.Parent = scrollingFrame
end
end
-- Call PopulateAccessories initially
PopulateAccessories()
-- Connect a function to update accessories when they change
player.CharacterAdded:Connect(PopulateAccessories)
btw im open for suggestions to improve the script, I’ve been trying for a while to make the system with nothing working, and I’m now trying by using humanoidDescription and getting the hat IDs there. I’m not sure if the script works yet though
. thanks
2 Likes
If this is a descendant of a SurfaceGui with ‘ResetOnSpawn’ enabled, it’s likely that the humanoid check is running before the character’s humanoid is actually created. Given this is a local script running each time the character is created, it’s fine to WaitForChild.
1 Like
It’s not, its a descendent from a screenGui in StarterGui (sorry for the late reply) :
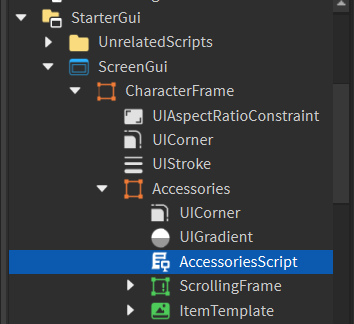
2 Likes
Sorry, brains a little frazzled right now lol. I did mean ScreenGui, I promise! I would recommend moving away from ResetOnSpawn, or at least using WaitForChild in your humanoid check.
1 Like
No problem
That did fix the problem! Thanks! 
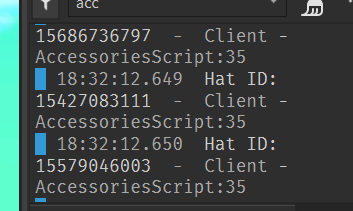
just wondering, is there a better way to get a player’s accessory ID’s?
(btw, I’m going eating, I’ll reply as quick as possible if someone has replied)
Try using HumanoidDescription:GetAccessories(). The docs provide a good example on how to use it.
1 Like
Verify that the anticipated outcome is being returned by the GetAppliedDescription() function. Additionally, confirm that the expected values that are separated by are included in the HatAccessory attribute. I also believe that adding a few debug yields would assist you to identify any potential problems.
1 Like
hey, i tried making something, does this look decent? I’m not the greatest at scripting, but i tried:
(btw that doesnt work)
2 Likes
actually i ended up going with the other sollution. It works fine and all, but it doesnt update. when my humanoidDescription changes, it doesnt add or remove the accessories in the scrollingframe, this is my script:
local player = game.Players.LocalPlayer
local template = script.Parent.ItemTemplate
local scrollingFrame = script.Parent.ScrollingFrame
local MarketplaceService = game:GetService("MarketplaceService")
-- String split function
local function splitString(inputstr, sep)
sep = sep or "%s"
local t = {}
for str in string.gmatch(inputstr, "([^"..sep.."]+)") do
table.insert(t, str)
end
return t
end
-- Function to clone and populate the accessories
local function PopulateAccessories()
-- Get Humanoid
local humanoid = player.Character:WaitForChild("Humanoid")
if not humanoid then
return -- Return if Humanoid is not found
end
-- Get HumanoidDescription
local humanoidDescription = humanoid:GetAppliedDescription()
print("HatAccessory:", humanoidDescription.HatAccessory)
-- Get hat accessory IDs (assuming they are comma-separated)
local hatAccessoryIds = {}
if humanoidDescription.HatAccessory ~= "" then
hatAccessoryIds = splitString(humanoidDescription.HatAccessory, ",")
end
print("Starting PopulateAccessories")
for _, accessoryId in ipairs(hatAccessoryIds) do
print("Hat ID:", accessoryId)
local clone = template:Clone()
clone.Parent = scrollingFrame
clone.Name = accessoryId
clone.Image = "http://www.roblox.com/Thumbs/Asset.ashx?Height=256&Width=256&AssetID=" .. accessoryId
local buyButton = clone.Frame.BuyButton
buyButton.MouseButton1Click:Connect(function()
MarketplaceService:PromptPurchase(player, accessoryId)
end)
end
end
-- Call PopulateAccessories initially
PopulateAccessories()
-- Connect a function to update accessories when they change
player.CharacterAdded:Connect(PopulateAccessories)
1 Like
The accessories in the scrolling frame don’t appear to be updating when the humanoidDescription adaptations, which appears to be the quandary you are discerning. You can resolve this by changing your PopulateAccessories function such that before adding new accessories, the ones that are currently in the scrolling frame are cleared out.
i figured my script by myself surprisingly, and i was wondering if there’d be a better way to update the items. I tried using “.changed”, but that did not work (maybe i was using it wrong). this is my complete script:
local player = game.Players.LocalPlayer
local template = script.Parent.ItemTemplate
local scrollingFrame = script.Parent.ScrollingFrame
local MarketplaceService = game:GetService("MarketplaceService")
local RunService = game:GetService("RunService")
-- String split function
local function splitString(inputstr, sep)
sep = sep or "%s"
local t = {}
for str in string.gmatch(inputstr, "([^"..sep.."]+)") do
table.insert(t, str)
end
return t
end
local selectedAccessoryId = nil
-- Function to clone and populate the accessories
local function PopulateAccessories()
for _, child in ipairs(scrollingFrame:GetChildren()) do
if child:IsA("ImageButton") then
child:Destroy()
end
end
local humanoid = player.Character:FindFirstChildOfClass("Humanoid")
if not humanoid then
return -- Return if Humanoid is not found
end
local humanoidDescription = humanoid:GetAppliedDescription()
local hatAccessoryIds = {}
if humanoidDescription.HatAccessory ~= "" then
hatAccessoryIds = splitString(humanoidDescription.HatAccessory, ",")
end
local hairAccessoryIds = {}
if humanoidDescription.HairAccessory ~= "" then
hairAccessoryIds = splitString(humanoidDescription.HairAccessory, ",")
end
local faceAccessoryIds = {}
if humanoidDescription.FaceAccessory ~= "" then
faceAccessoryIds = splitString(humanoidDescription.FaceAccessory, ",")
end
local backAccessoryIds = {}
if humanoidDescription.BackAccessory ~= "" then
backAccessoryIds = splitString(humanoidDescription.BackAccessory, ",")
end
-- Combine all accessory IDs into one table
local allAccessoryIds = {}
for _, id in ipairs(hatAccessoryIds) do
table.insert(allAccessoryIds, id)
end
for _, id in ipairs(hairAccessoryIds) do
table.insert(allAccessoryIds, id)
end
for _, id in ipairs(faceAccessoryIds) do
table.insert(allAccessoryIds, id)
end
for _, id in ipairs(backAccessoryIds) do
table.insert(allAccessoryIds, id)
end
print("Starting PopulateAccessories")
for _, accessoryId in ipairs(allAccessoryIds) do
print("Accessory ID:", accessoryId)
local clone = template:Clone()
clone.Parent = scrollingFrame
clone.Name = accessoryId
clone.Image = "http://www.roblox.com/Thumbs/Asset.ashx?Height=256&Width=256&AssetID=" .. accessoryId
local openFrame = clone.Frame
clone.MouseButton1Click:Connect(function()
if selectedAccessoryId then
local previousSelectedAccessory = scrollingFrame:FindFirstChild(selectedAccessoryId)
if previousSelectedAccessory then
previousSelectedAccessory.Frame.Visible = false
end
end
-- Show selected accessory's frame
openFrame.Visible = true
selectedAccessoryId = accessoryId
end)
local buyButton = clone.Frame.BuyButton
buyButton.MouseButton1Click:Connect(function()
MarketplaceService:PromptPurchase(player, accessoryId)
end)
local removeButton = clone.Frame.RemoveButton
removeButton.MouseButton1Click:Connect(function()
game.ReplicatedStorage.removeSingle:FireServer(accessoryId)
end)
end
end
PopulateAccessories()
local updateInterval = 1 -- Update every second
local elapsedTime = 0
RunService.Heartbeat:Connect(function(deltaTime)
elapsedTime = elapsedTime + deltaTime
if elapsedTime >= updateInterval then
elapsedTime = 0
PopulateAccessories()
end
end)
still available if someone is down to help 
I made a few changes to the script, if someone is down to help thatd be great