So I am making a boat and when the player pushes W, the player’s boat will move.
BUT, I’m stuck at the part where I make it move and then stop moving.
Here is my script so far:
local thing = script.Parent
local root = thing.PrimaryPart
local event = game.ReplicatedStorage.Moving
event.OnServerEvent:Connect(function(plr, moving)
for i, boat in pairs(workspace.Boats:GetChildren()) do
if boat.Owner.Value == plr.Name then
if moving then
elseif not moving then
end
end
end
end)
And the local script located in StarterPlayerScripts:
local uis = game:GetService("UserInputService")
local event = game.ReplicatedStorage.Moving
uis.InputBegan:Connect(function(input, gpe)
if input.KeyCode == Enum.KeyCode.W then
event:FireServer(true)
end
end)
uis.InputEnded:Connect(function(input, gpe)
if input.KeyCode == Enum.KeyCode.W then
event:FireServer(false)
end
end)
4 Likes
Have you looked into using a BodyMover
?
You would use one of these to make the boat move
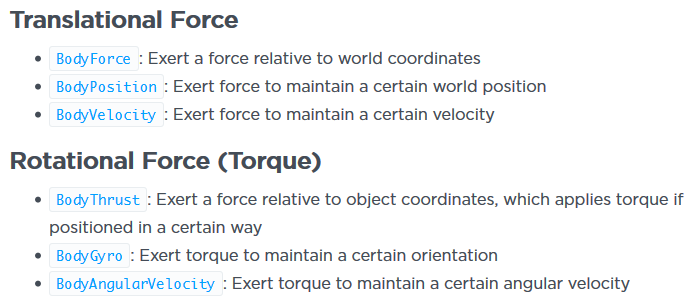
BodyMovers: BodyMover
I was thinking of using it, but I’ve never used it before.
They have some good examples in the docs for the idnividual BodyMover
s.
For example, the `BodyForce` has an example of how to reduce gravity
Source: BodyForce
Ok great!
So I tried applying it to my script and nothing happened:
local event = game.ReplicatedStorage.Moving
event.OnServerEvent:Connect(function(plr, moving)
for i, boat in pairs(workspace.Boats:GetChildren()) do
if boat.Owner.Value == plr.Name then
if moving then
local bodyPos = Instance.new("BodyPosition",boat.PrimaryPart)
bodyPos.Name = "Mover"
bodyPos.Position = boat.PrimaryPart.Position
while bodyPos ~= nil do
wait(.1)
bodyPos.Position = bodyPos.Position + Vector3.new(1,0,0)
end
elseif not moving then
local bodyPos = boat.PrimaryPart:WaitForChild("Mover")
bodyPos:Destroy()
end
end
end
end)
The primary part has weld constraints for each part in the boat:
The primary part is also anchored and cancollide is false and the other parts are anchored and cancollide is true.
I think you need to unanchor all the parts. If it still doesn’t work, try taking a look at this list?
Still not moving after unanchoring every part, and making the MaxForce higher.
local event = game.ReplicatedStorage.Moving
event.OnServerEvent:Connect(function(plr, moving)
for i, boat in pairs(workspace.Boats:GetChildren()) do
if boat.Owner.Value == plr.Name then
if moving then
local bodyPos = Instance.new("BodyPosition",boat.PrimaryPart)
bodyPos.Name = "Mover"
bodyPos.Position = boat.PrimaryPart.Position
bodyPos.MaxForce = Vector3.new(10000,10000,10000)
while bodyPos ~= nil do
wait(.1)
bodyPos.Position = bodyPos.Position + Vector3.new(1,0,0)
end
elseif not moving then
local bodyPos = boat.PrimaryPart:WaitForChild("Mover")
bodyPos:Destroy()
end
end
end
end)
The BodyPosition is appearing inside of the part though…
I think I know the problem. The boat I’m using might be too heavy, so that’s why it isn’t moving.
Turning on the massless
problem might help
1 Like
I already tried, and it didn’t work.
I’m experimenting with CustomPhysicalProperties
to see if that helps.
That didn’t work either. Tried looking at the Developer Hub, but that didn’t really help either.
I decided I’d just stick with TweenService so like:
- Player presses E and boat starts moving
- Player presses E again and boat stops moving