Answer (TL;DR)
No, not at all.
I don’t think this is micro-optimization; I don’t know how many RBX Signals are needed to connect to crash a game (let alone lag a game.) but as long as it’s in ServerScriptService; you should be okay, however don’t abuse the system. The most i’ve ever had is 58 in different scripts, it was a poor choice as that was when I first started scripting and it was unorganized and unnecessary.
What To Do
Option #1
For starts, you could just run multiple functions in a script with a spawn(function())
or a coroutine.wrap(function()end)()
. On-top of that, you could call each function and if you want optimal organization, use one module and call to it through the script and the module can do what you need it to do.
First Example
game.Players.PlayerAdded:Connect(function(plr)
--[[I alternate between the two depending on what i'm programming, use this as an example.]]
spawn(function()
--Call a function here or program here in this.
end)
coroutine.wrap(function()
--Call a function here or program here in this.
end)()
end)
Option #2 (Not Recommended)
You could put multiple connections into a script, like so;
Second Example
game.Players.PlayerAdded:Connect(function(plr)
--[[I alternate between the two depending on what i'm programming, use this as an example.]]
spawn(function()
--Call a function here or program here in this.
end)
coroutine.wrap(function()
--Call a function here or program here in this.
end)()
end)
game.Players.PlayerAdded:Connect(function(plr)
--[[I alternate between the two depending on what i'm programming, use this as an example.]]
spawn(function()
--Call a function here or program here in this.
end)
coroutine.wrap(function()
--Call a function here or program here in this.
end)()
end)
game.Players.PlayerAdded:Connect(function(plr)
--[[I alternate between the two depending on what i'm programming, use this as an example.]]
spawn(function()
--Call a function here or program here in this.
end)
coroutine.wrap(function()
--Call a function here or program here in this.
end)()
end)
Option #3
The last thing you could do is have it tied to a module and call the functions in the module;
I won’t give a full example of this because it would be tedious to, there are plenty of examples of modules in use you can look at and Roblox has a great example of Modules and the functions inside of them; click here to view it.
What I Do
Here’s a picture example of what I do now of days;
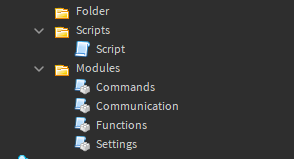
I only have multiple modules because I had to have multiple modules. You can’t have modules communicate with each other on their own so I had to make this system. It’s a Module Communication system, Functions are to do things inside of the other things such as Grab the Player or tool throughout a table… etc, Commands are for admin commands, Communication is so I can communicate in-between each module and Settings is the most self explanatory, it has the settings for the other modules to refer to.
I hope this answers your question, if it did; mark it as the solution… have a great day and reply with any questions.