Functions can utilize parameters for data that will be passed in. When you declare a function, you may include one or more parameter names inside the parentheses; for example this function uses the parameter “hit”. When calling a function with parameters, specify the values that should be passed to the function. For instance, the following function takes the two numbers passed in during each call, adds them together, and outputs the result:
> local function addNumbers(num1, num2)
> local result = num1 + num2
> print(num1 .. " + " .. num2 .. " = " .. result)
> end
>
> addNumbers(2, 3)
> addNumbers(4, 0.5)
> addNumbers(1000, 500)
This particular function in question is an event triggered function, which does what you expect, it’s run when triggered by an event. The above function was just an example of parameters. In this case, script.Parent.Touched:connect
refers to the parent of the script, and when the parent part is touched it triggers the function when it gets a connection. Each object in roblox has 3 (for the most part, sometimes more) catagories of properties, in this case, “Part” has Properties, Functions, and Events. Properties are physical appearance and position, functions are the functions that can be used in conjunction with code referring to or calling the part, and events are things that are triggered when certain things happen with the part. Part has 2 Events: Touched, and onTouched. So, when the part comes in contact with another part, the touched event is fired. The function that precedes this is now run, and the function has the parameter of “hit”.
if hit and hit.Parent and hit.Parent:FindFirstChild("Humanoid")
The function contents start with an if
statement. First it checks if hit (if the event was fired) then it checks if the object that touched touched the parent of the script, which is Part
. If both of those are true, then it checks if the parented part was touched by an instance with the given name “Humanoid”. If so, it returns the first child of the instance of the given name “Humanoid”. If all 3 of those are true, it then sets the health of the humanoid whose parent collided with the script’s parent to 0.
Now, onto your next question of why they didn’t use
script.Parent.OnTouch:connect(touch)
if touch then
Humanoid.health = 0
There are a few issues with this code.
- There is no such event called OnTouch inherited from BasePart.
- There is no end/semicolon to justify the end of the statement.
- “touch” is in parentheses, even though you didn’t call a function
Now, because you are new to Lua and don’t fully understand all the syntax and stuff, I’ve fixed those few rookie mistakes for you. It can be hard to remember at first but you’ll get it.
script.Parent.OnTouch:connect(function(touch)
if touch then Humanoid.Health = 0 end
end)
Now that we’ve fixed those minor mistakes, you are left with 2 warnings.
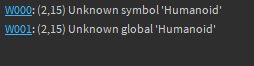
Humanoid is unknown because it is undefined, and simply defining it as a variable won’t work either. If you just defined it as a variable, it cannot have health properties. The “.” in between Humanoid.Health signifies an distinguishment in inheritance, so anything that comes after the period is a child of the instance that precedes the period. Since Humanoid is not defined and has no parent, a warning occurs and the code is for the most part useless. If you were to clarify that the humanoid was linked up with a player, anything that the part collided with would kill the player by doing touch.Parent:FindFirstChild("Humanoid")
after the then
, nothing would happen still because the computer doesn’t know if it hit the part.
One major characteristic you need to remember about coding is that computers are only as smart as you want them to be, and need to be told certain instructions step by step is a certain way. It’s kinda hard to comprehend for people new to programming, because you never think logically that you have to spoon feed instructions a certain way to a machine that has processing speeds and reaction times faster than 10 people.
I hope this helped you out some, I tried to explain as least as possible without having to go into the technical details all while making sense at the same time. So if there are any logical holes in my explanation please feel free to ask any questions