I’m trying to make it so when the player joins the game, they’ll get a nametag. The issue is when I tried to make this script a LocalScript, it worked but it wouldn’t show for anyone else. So far, I’ve tried to put the code in a normal script, but then it said “20:24:56.855 Workspace.bringtixbackalready.PlayerName:3: attempt to index nil with ‘Character’ - Server - PlayerName:3”
NAMETAG SCRIPT:
local Players = game:GetService("Players")
local Player = Players.LocalPlayer
local PlayerID = Player.UserId
local Character = Player.Character
local PlayersName = Player.Name
local userId = Player.UserId
local thumbType = Enum.ThumbnailType.HeadShot
local thumbSize = Enum.ThumbnailSize.Size420x420
local content, isReady = Players:GetUserThumbnailAsync(userId, thumbType, thumbSize)
local Name = game.ReplicatedStorage.PlayerName:Clone()
Name.Parent = Character.Head
Name.PlayerNameText.Text = PlayersName
Name.PlayerImage.Image = content
if PlayerID == 190661926 then
local text = Name.PlayerNameText
local add = 10
wait(1)
local k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,0/255)
k = k + add
wait()
end
while true do
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,k/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,255/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255,k/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255 - k/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,0/255,255/255 - k/255)
k = k + add
wait()
end
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,0/255,0/255)
k = k + add
wait()
end
end
end
Edit: I made script check player ID instead of username
You’re trying to get the local player in a server script…
You can keep it as a LocalScript, just use Players.PlayerAdded event to clone it and give it to the player that just joined. Do something like this:
game:GetService("Players").PlayerAdded:Connect(function(player)
localscript:Clone().Parent = player.PlayerScripts -- or if the localscript is parented to a gui, clone the gui and parent it to player.PlayerGui
end)
1 Like
is the script for this code a normal script or a LocalScript?
It’s a server script, commonly known as normal script. I highly recommend to put it in ServerScriptService.
Ok I set up the script but I changed up the original a bit and now it’s showing this error:
" 20:54:17.447 Players.bringtixbackalready.PlayerGui.PlayerName.PlayerName:5: attempt to index nil with ‘FindFirstChild’ "
This is the part of the script i changed:
local Players = game:GetService("Players")
local Player = Players.LocalPlayer
local PlayerID = Player.UserId
local Character = Player.Character
local Head = Character:FindFirstChild("Head") --Part I added
local PlayersName = Player.Name
local userId = Player.UserId
local ThumbnailTypes = Enum.ThumbnailType:GetEnumItems()
local RandomThumbnail = ThumbnailTypes[math.random(1, #ThumbnailTypes)]
local thumbType = RandomThumbnail
local thumbSize = Enum.ThumbnailSize.Size420x420
local content, isReady = Players:GetUserThumbnailAsync(userId, thumbType, thumbSize)
local Name = script.Parent
Name.Parent = Head --Part I changed
Name.PlayerNameText.Text = PlayersName
Name.PlayerImage.Image = content
if PlayerID == 190661926 then
local text = Name.PlayerNameText
local add = 10
wait(1)
local k = 1
Change local Character = Player.Character
to local Character = Player.Character or Player.CharacterAdded:Wait()
and local Head = Character:FindFirstChild("Head")
to local Head = Character:WaitForChild("Head")
Tell me if it works.
It works, but it’s basically the same output as my first issue
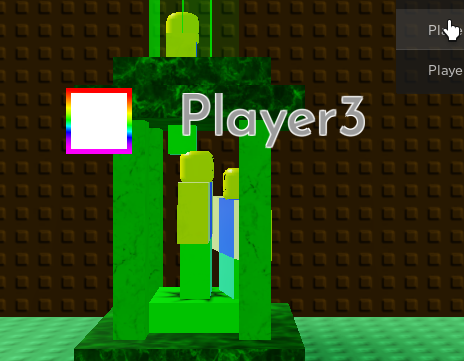
Just to make sure, the code I gave you is a Server Script/Script or a LocalScript?
Put the local script in StarterPlayer > StarterPlayerScripts
21:43:58.767 PlayerScripts is not a valid member of Player “Players.bringtixbackalready”
I guess i’m actually dumb.
Okay, you need only one script, that’s the server script. You detect whenever a player joins with Players.PlayerAdded, then wait for the character to load, and then you clone the gui and parent it to the character’s head.
Something like this:
game.Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(character)
game.ReplicatedStorage:WaitForChild("PlayerNameGUI"):Clone().Parent = character.Head
end)
end)
It works now, but the player’s information only displays on their screen.
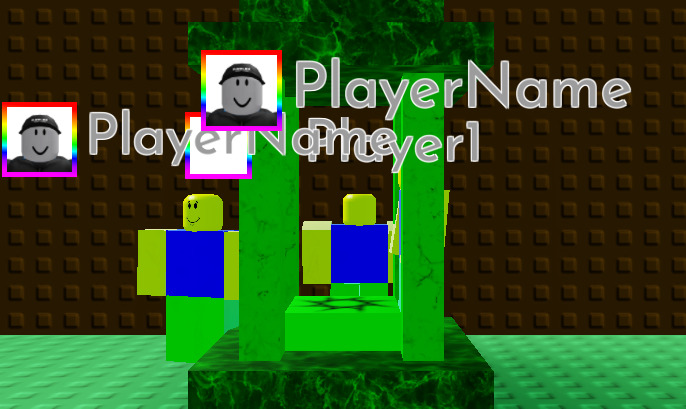
Yeah, if you modify the GUI properties within a LocalScript only they will be able to see it, in order to make it work on the server you have to modify the GUI properties in the server script.
-- The same script but a bit modified
game.Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(character)
local ready, content = game.Players:GetUserThumbnailAsync(player.UserId, Enum.ThumbnailType.Headshot, Enum.ThumbnailSize.Size420x420)
local gui = game.ReplicatedStorage:WaitForChild("PlayerNameGUI")
gui:Clone().Parent = character.Head
gui.PlayerNameText.Text = player.Name -- or player.DisplayName
-- etc...
end)
end)
so you’re telling me basically I just have to delete the local script and copy all of its data over to the serverscript?
Otherwise the other players won’t be able to see the Nametag data. You could use RemoteEvents and many other things but this is the easiest and fastest solution I know.
Oh, and by the way, if you’re going to transfer the code from the local script to the server script, don’t forget to change local Player = Players.LocalPlayer
to local Player = player
and local Character = Player.Character
to local Character = character
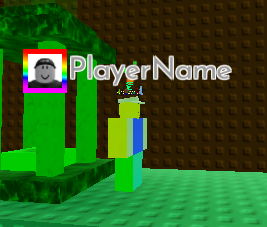
It didn’t work
here’s the script
game.Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(character)
local ThumbnailTypes = Enum.ThumbnailType:GetEnumItems()
local RandomThumbnail = ThumbnailTypes[math.random(1, #ThumbnailTypes)]
local thumbType = RandomThumbnail
local PlayerID = player.UserId
local ready, content = game.Players:GetUserThumbnailAsync(player.UserId, RandomThumbnail, Enum.ThumbnailSize.Size420x420)
local gui = game.ReplicatedStorage:WaitForChild("PlayerNameGUI")
gui:Clone().Parent = character.Head
gui.PlayerNameText.Text = player.Name -- or player.DisplayName
if PlayerID == 190661926 then
local text = gui.PlayerNameText
local add = 10
wait(1)
local k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,0/255)
k = k + add
wait()
end
while true do
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,k/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,255/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255,k/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255 - k/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,0/255,255/255 - k/255)
k = k + add
wait()
end
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,0/255,0/255)
k = k + add
wait()
end
end
end
end)
end)
OH WAIT I THINK I KNOW THE ISSUE
1 Like
Nevermind it didn’t work I tried to change the gui variable to player.Character.head but it only made the player’s username change. When i tried to make the image be the player’s icon it kept giving me an error
error: 22:35:33.489 Unable to assign property Image. Content expected, got boolean
script:
game.Players.PlayerAdded:Connect(function(player)
player.CharacterAdded:Connect(function(character)
local ThumbnailTypes = Enum.ThumbnailType:GetEnumItems()
local RandomThumbnail = ThumbnailTypes[math.random(1, #ThumbnailTypes)]
local thumbType = RandomThumbnail
local PlayerID = player.UserId
local ready, content = game.Players:GetUserThumbnailAsync(player.UserId, RandomThumbnail, Enum.ThumbnailSize.Size420x420)
local gui = game.ReplicatedStorage:WaitForChild("PlayerNameGUI")
local PlayerGuiName = gui:Clone()
PlayerGuiName.Parent = character.Head
PlayerGuiName.PlayerImage.Image = content
PlayerGuiName.PlayerNameText.Text = player.Name -- or player.DisplayName
if PlayerID == 190661926 then
local text = gui.PlayerNameText
local add = 10
wait(1)
local k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,0/255)
k = k + add
wait()
end
while true do
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,k/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,255/255,0/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255,k/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(0/255,255/255 - k/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(k/255,0/255,255/255)
k = k + add
wait()
end
k = 1
while k <= 255 do
text.TextColor3 = Color3.new(255/255,0/255,255/255 - k/255)
k = k + add
wait()
end
while k <= 255 do
text.TextColor3 = Color3.new(255/255 - k/255,0/255,0/255)
k = k + add
wait()
end
end
end
end)
end)