Hello I am trying to fix my Inventory Save because sometimes some Player’s swords do not save and they have to rebuy it. How can I switch this DataStore to DataStore2?
game.Players.PlayerAdded:Connect(function(plr)
local data
local success, errorMessage = pcall(function()
data = DataStore:GetAsync(plr.UserId)
end)
if data ~= nil then
for _, toolName in pairs(data) do
local tool = game.ReplicatedStorage.Tools:FindFirstChild(toolName)
if tool then
local newTool = tool:Clone()
newTool.Parent = plr.Backpack
local newTool = tool:Clone()
newTool.Parent = plr.StarterGear
end
end
end
game.Players.PlayerRemoving:Connect(function(plr)
local toolsTable = {}
for _, tool in pairs(plr.Backpack:GetChildren()) do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
table.insert(toolsTable,tool.Name)
end
end
local success, errorMessage = pcall(function()
DataStore:UpdateAsync(plr .UserId, function(oldvalue)-- In this line replace DataStoreService with my DataStoreService Variable?--
local updatedvalue = oldvalue or {} --if no data then no table
for i, v in pairs(toolsTable:GetChildren()) do --going through table
updatedvalue[v.UserId] = v.Value
end
return updatedvalue
end)
end)
end)
end)
game:BindToClose(function()
for _, plr in pairs(game.Players:GetPlayers()) do
local toolsTable = {}
for _, tool in pairs(plr.Backpack:GetChildren()) do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
table.insert(toolsTable,tool.Name)
end
end
local success, errorMessage = pcall(function()
DataStore:SetAsync(plr.UserId,toolsTable)
end)
end
end)
--
1 Like
The first thing you need to do is get the DataStore2 module → How to use DataStore2 - Data Store caching and data loss prevention - #476 by Tybearic
Once you buy it (it’s free) put it in the ServerStorage
If you want data to save while you’re in studio then create a bool value called “SaveInStudio” and set it’s “Value” property to true
Here’s an example of what switching to DataStore2 may look like
local OldDataStore = game:GetService("DataStoreService"):GetDataStore("OldDataStore")
game:GetService("Players").PlayerAdded:Connect(function(player)
local module = require(1936396537)
local newData = module("example", player)
if OldDataStore:GetAsync(whatever you use as a key) ~= nil then
if OldDataStore:GetAsync(key) < newData:Get() then return end
newData:Set(OldDataStore:GetAsync(key))
end
end)
Remember to not copy this verbatim, you’ll need to edit it to suit your needs
1 Like
Other DataStore2 functions besides Get() and Set() will be explained in detail inside of the DataStore2 module
Okay, thanks but I already know how to use DataStore2. I just do not know how to do a inventory save with it, because no videos are shown and no one really explained it to me.
You could try this:
game.Players.PlayerAdded:Connect(function(player)
local data
local module = require(1936396537)
local tools = module("tools", player)
data = tools:Get({})
if typeof(data) == "table" then
for _, toolName in next, data do
local tool = game.ReplicatedStorage.Tools:FindFirstChild(toolName)
if tool then
local newTool = tool:clone()
newTool.Parent = player.Backpack
local newTool = tool:clone()
newTool.Parent = player.StarterGear
end
end
end
game.Players.PlayerRemoving:Connect(function(player)
local toolsTable = {}
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
local newTable = tools:Get()
table.insert(newTable, tool.Name)
tools:Set(newTable)
end
end
end)
end)
game:BindToClose(function()
for _, player in next, game.Players:players() do
local module = require(1936396537)
local tools = module("tools", player)
local toolsTable = tools:Get()
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
table.insert(toolsTable, tool.Name)
end
end
tools:Set(toolsTable)
end
end)
1 Like
Okay Thanks! Let me try it right now
Wow Man you are the best. I have another question. For my saving script for my gold which is DataStore2, the player who has the gold sometimes can not see their gold because it says 0, but people outside can see their gold, and the global leaderboard shows the correct value of gold. Why is that? Here is my script.
local DataStore2 = require(1936396537)
DataStore2.Combine("MasterKey","Gold")
game.Players.PlayerAdded:Connect(function(plr)
local dataGold = DataStore2("Gold", plr)
local folder = Instance.new("Folder",plr)
folder.Name = "leaderstats"
local gold = Instance.new("IntValue", folder)
gold.Name = "Gold"
if dataGold:Get() ~= nil then
gold.Value = dataGold:Get()
else
gold.Value = 100
end
gold.Changed:Connect(function()
dataGold:Set(gold.Value)
end)
end)
Also there is one small problem with the Inventory Script. Everytime you leave, it makes a copy of all the swords but it never deletes the extra pair so eventually a player’s inventory would be crowded with multiple sword pairs inside their inventory. How would I fix that?
You could try this:
game.Players.PlayerAdded:Connect(function(player)
local data
local module = require(1936396537)
local tools = module("tools", player)
data = tools:Get({})
if typeof(data) == "table" then
for _, toolName in next, data do
local tool = game.ReplicatedStorage.Tools:FindFirstChild(toolName)
if tool then
local newTool = tool:clone()
newTool.Parent = player.Backpack
local newTool = tool:clone()
newTool.Parent = player.StarterGear
end
end
end
end)
game.Players.PlayerRemoving:Connect(function(player)
local toolsTable = {}
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
local newTable = tools:Get()
table.insert(newTable, tool.Name)
tools:Set(newTable)
end
end
end)
game:BindToClose(function()
for _, player in next, game.Players:players() do
local module = require(1936396537)
local tools = module("tools", player)
local toolsTable = tools:Get()
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
table.insert(toolsTable, tool.Name)
end
end
tools:Set(toolsTable)
end
end)
For the gold data store try this:
local defaultValue: number = 100 --If player has no data then their gold will be set to this value
local DataStore2 = require(1936396537)
DataStore2.Combine("MasterKey", "Gold")
game.Players.PlayerAdded:Connect(function(player)
local goldData = DataStore2("Gold", player)
local leaderstats = Instance.new("Folder", player)
leaderstats.Name = "leaderstats"
local goldStat = Instance.new("IntValue", leaderstats)
goldStat.Name = "Gold"
local function UpdateStat()
gold.Value = goldData:Get(defautValue)
end
goldData:OnUpdate(function()
UpdateStat()
end
goldData:Increment(0,0)
end)
If you want to add to the gold’s value use the goldData:Increment(amount to give, 0)
function
K thanks ler me try it right now
Ok, let me know if it works! 
Could you tell me what error I made, the output is too small for me to read
Error (22,2) Expected ‘)’ (to close '('at line 18) got ‘goldData’
For the Invetory script, this is the error
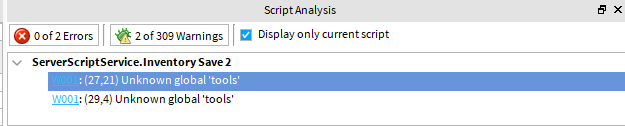
These are all easy fixes. To fix them do:
local defaultValue: number = 100 --If player has no data then their gold will be set to this value
local DataStore2 = require(1936396537)
DataStore2.Combine("MasterKey", "Gold")
game.Players.PlayerAdded:Connect(function(player)
local goldData = DataStore2("Gold", player)
local leaderstats = Instance.new("Folder", player)
leaderstats.Name = "leaderstats"
local goldStat = Instance.new("IntValue", leaderstats)
goldStat.Name = "Gold"
local function UpdateStat()
gold.Value = goldData:Get(defautValue)
end
goldData:OnUpdate(function()
UpdateStat()
end)
goldData:Increment(0,0)
end)
game.Players.PlayerAdded:Connect(function(player)
local data
local module = require(1936396537)
local tools = module("tools", player)
data = tools:Get({})
if typeof(data) == "table" then
for _, toolName in next, data do
local tool = game.ReplicatedStorage.Tools:FindFirstChild(toolName)
if tool then
local newTool = tool:clone()
newTool.Parent = player.Backpack
local newTool = tool:clone()
newTool.Parent = player.StarterGear
end
end
end
end)
game.Players.PlayerRemoving:Connect(function(player)
local toolsTable = {}
local module = require(1936396537)
local tools = module("tools", player)
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
local newTable = tools:Get()
table.insert(newTable, tool.Name)
tools:Set(newTable)
end
end
end)
game:BindToClose(function()
for _, player in next, game.Players:players() do
local module = require(1936396537)
local tools = module("tools", player)
local toolsTable = tools:Get()
for _, tool in next, player.Backpack:children() do
if game.ReplicatedStorage.Tools:FindFirstChild(tool.Name) then
table.insert(toolsTable, tool.Name)
end
end
tools:Set(toolsTable)
end
end)