Sorry for the really late reply, got busy!
So I was in the place file that you sent me (thank you so much) and I was trying to implement your code into mine and this is what I have so far. I tried to make it known what was new and old and or original.
local UserInputService = game:GetService("UserInputService")
local Players = game:GetService("Players")
local CollectionService = game:GetService("CollectionService")
local MarketplaceService = game:GetService("MarketplaceService")
local RunService = game:GetService("RunService")
local TweenService = game:GetService("TweenService")
local LocalPlayer = Players.LocalPlayer
local Character = LocalPlayer.Character or LocalPlayer.CharacterAdded:Wait()
local Head = Character:WaitForChild("Head")
local GameDataModule = require(game.ReplicatedStorage.Modules.GameDataModule)
local RankDataModule = require(game.ReplicatedStorage.Modules.RankDataModule)
local HighlightItemsModule = require(game.ReplicatedStorage.Modules.HighlightItemsModule)
local ItemTooltip = script.Parent.ItemTooltip
local PlayerInformation = script.Parent.PlayerInformation
local PlayerInformationSelectText = script.Parent.PlayerInformation.SelectText
local UserIDBox = script.Parent.UserID
local UserIDBoxSelectText = script.Parent.UserID.SelectText
local ItemMagnitude = GameDataModule.ItemMagnitude
local CameraMagnitude = GameDataModule.CameraMagnitude
local Camera = game.Workspace.CurrentCamera
local Mouse = LocalPlayer:GetMouse()
local DestroyScript = LocalPlayer:GetRankInGroup(GameDataModule.GroupID) <= RankDataModule.Trainee
local Employee = LocalPlayer:GetRankInGroup(GameDataModule.GroupID) >= RankDataModule.JuniorBarista
local HasPremium = MarketplaceService:UserOwnsGamePassAsync(LocalPlayer.UserId, GameDataModule.Gamepass_Premium)
local PlayerIsInGroup = LocalPlayer:IsInGroup(GameDataModule.GroupID)
local RealPlayersName = ""
local DetectedNamesUserID = 0
local RaycastParameters --[[New]]
local GetMouseData = require(script:WaitForChild("MouseData")) --[[New]]
local Thread --[[New]]
local FormatText = "<b>@%s (%d)</b>\nCmd/Ctrl + Right Click to Select" --[[New]]
local function GetUserIDFromName(Name)
local UserID
local Success = pcall(function()
UserID = Players:GetUserIdFromNameAsync(Name)
end)
return Success and UserID or nil
end
local function UpdateTooltipPosition()
ItemTooltip.Position = UDim2.new(0, Mouse.X + 16, 0, Mouse.Y + 55)
PlayerInformation.Position = UDim2.new(0, Mouse.X + 16, 0, Mouse.Y + 55)
UserIDBox.Position = UDim2.new(0, Mouse.X + 16, 0, Mouse.Y + 55)
end
local function CreateHighlight(Target)
if not Target:FindFirstChildWhichIsA("Highlight") then
local NewHighlight = Instance.new("Highlight")
NewHighlight.DepthMode = Enum.HighlightDepthMode.Occluded
NewHighlight.FillTransparency = 1
NewHighlight.OutlineTransparency = 1
NewHighlight.Parent = Target
NewHighlight.Name = Target.Name.."Highlight"
return NewHighlight
end
end
--[[Removed]]
local function FadeInHighlight(Highlight, FadeIn)
if Highlight == nil then return end
local Goal = FadeIn and {OutlineTransparency = 0} or {OutlineTransparency = 1}
local Tween = TweenService:Create(Highlight, TweenInfo.new(0.5), Goal)
Tween:Play()
warn("Applied Highlight")
end
--[[New]]
--[[local function FadeInHighlight(Highlight, FadeIn)
repeat
Highlight.OutlineTransparency = math.max(Highlight.OutlineTransparency - (task.wait() / FadeIn), 0)
until Highlight.OutlineTransparency == 0
end]]
local function FadeOutAndDestroy(Highlight)
if Highlight and Highlight.Parent then
Highlight:Destroy()
warn("Deleted Highlight")
end
end
local function CheckClothes(PlayerName)
local Shirt = game.Workspace[PlayerName]:FindFirstChild("Shirt")
local Pants = game.Workspace[PlayerName]:FindFirstChild("Pants")
if Shirt.ShirtTemplate == "http://www.roblox.com/asset/?id=1" or Shirt.ShirtTemplate == "http://www.roblox.com/asset/?id=1" or Shirt.ShirtTemplate == "http://www.roblox.com/asset/?id=1" or Shirt.ShirtTemplate == "http://www.roblox.com/asset/?id=1" and Pants.PantsTemplate == "http://www.roblox.com/asset/?id=1" or Pants.PantsTemplate == "http://www.roblox.com/asset/?id=1" or Pants.PantsTemplate == "http://www.roblox.com/asset/?id=1" or Pants.PantsTemplate == "http://www.roblox.com/asset/?id=1" then
return true
else
return false
end
end
--[[New]]
local function SetRaycastParams(Character)
local RaycastParams = RaycastParams.new()
RaycastParams.FilterDescendantsInstances = {Character}
RaycastParams.FilterType = Enum.RaycastFilterType.Exclude
RaycastParameters = RaycastParams
end
--[[New]]
SetRaycastParams(LocalPlayer.Character or LocalPlayer.CharacterAdded:Wait())
LocalPlayer.CharacterAdded:Connect(SetRaycastParams)
local function UpdateTooltip()
--[[New]] local Player, Character, MouseLocation = GetMouseData(RaycastParameters)
local Target = Mouse.Target
UpdateTooltipPosition()
local IsWithinCameraMagnitude = (Head.CFrame.Position - Camera.CFrame.Position).Magnitude < CameraMagnitude
if Target and Target.Parent then
local IsHighlightedItem = false
for _, Tags in pairs(HighlightItemsModule) do
for _, TaggedItem in pairs(CollectionService:GetTagged(Tags)) do
if TaggedItem == Target.Parent then
IsHighlightedItem = true
break
end
end
end
if IsHighlightedItem or Target.Parent:IsA("Model") and Target.Parent.Parent == game.Workspace or Target.Parent.Parent:IsA("Model") and Target.Parent.Parent.Parent == game.Workspace then
local KitchenTrashcanAttribute = Target.Parent:GetAttribute("KitchenTrashcan")
local PremiumTrashcanAttribute = Target.Parent:GetAttribute("PremiumTrashcan")
local IsTrashcan = string.sub(Target.Parent.Name, 1, #"Trashcan") == "Trashcan"
local HasUniform = CheckClothes(LocalPlayer.Name)
local IsWithinItemMagnitude = nil
if IsHighlightedItem and Target.Parent.Hitbox then
IsWithinItemMagnitude = (Head.Position - Target.Parent.Hitbox.Position).Magnitude < ItemMagnitude
end
if IsTrashcan and not KitchenTrashcanAttribute and not PremiumTrashcanAttribute and IsWithinItemMagnitude and IsWithinCameraMagnitude then
ItemTooltip.Text = Target.Parent:GetAttribute("ItemName") or ""
if not Target.Parent:FindFirstChild(Target.Parent.Name.."Highlight") then
local NewHighlight = CreateHighlight(Target.Parent)
FadeInHighlight(NewHighlight, 0.5)
end
elseif Employee and IsWithinItemMagnitude and IsWithinCameraMagnitude and (Target.Parent.Parent.Parent.Name == "Kitchen" or Target.Parent.Parent.Parent.Parent.Name == "Kitchen") and HasUniform then
ItemTooltip.Text = Target.Parent:GetAttribute("ItemName") or ""
if not Target.Parent:FindFirstChild(Target.Parent.Name.."Highlight") then
local NewHighlight = CreateHighlight(Target.Parent)
FadeInHighlight(NewHighlight, 0.5)
end
elseif Employee and IsWithinItemMagnitude and IsWithinCameraMagnitude and (Target.Parent.Parent.Parent.Name == "BaristaLounge" or Target.Parent.Parent.Parent.Parent.Name == "BaristaLounge") then
ItemTooltip.Text = Target.Parent:GetAttribute("ItemName") or ""
if not Target.Parent:FindFirstChild(Target.Parent.Name.."Highlight") then
local NewHighlight = CreateHighlight(Target.Parent)
FadeInHighlight(NewHighlight, 0.5)
end
elseif HasPremium and Target.Parent.Name == "Trashcan7" and IsWithinItemMagnitude and IsWithinCameraMagnitude then
ItemTooltip.Text = Target.Parent:GetAttribute("ItemName") or ""
if not Target.Parent:FindFirstChild(Target.Parent.Name.."Highlight") then
local NewHighlight = CreateHighlight(Target.Parent)
FadeInHighlight(NewHighlight, 0.5)
end
end
end
--[[Old]]
--[[if IsWithinCameraMagnitude and PlayerIsInGroup and not DestroyScript and ((Target.Parent:IsA("Model") and Target.Parent.Parent == game.Workspace) or (Target.Parent.Parent:IsA("Model") and Target.Parent.Parent.Parent == game.Workspace)) and not (Target.Parent:FindFirstChildWhichIsA("Highlight") or Target.Parent.Parent:FindFirstChildWhichIsA("Highlight")) then
print("Check - A")
DetectedNamesUserID = GetUserIDFromName(Target.Parent.Name)
if DetectedNamesUserID then
RealPlayersName = Players:GetNameFromUserIdAsync(DetectedNamesUserID)
local NewHighlight = CreateHighlight(Target.Parent)
PlayerInformation.Text = "@"..RealPlayersName.." ("..DetectedNamesUserID..")"
.Text = "Cmd/Ctrl + Right Click to Select"
FadeInHighlight(NewHighlight, 0.5)
print("Player")
end
end
for _, Model in pairs(game.Workspace:GetDescendants()) do
if Model:IsA("Model") and Model.Name ~= Target.Parent.Name or (Head.CFrame.Position - Camera.CFrame.Position).Magnitude > CameraMagnitude then
for _, ModelHighlight in pairs(Model:GetChildren()) do
if ModelHighlight:IsA("Highlight") then
print("Check - B [Deleted]")
ItemTooltip.Text = ""
PlayerInformation.Text = ""
PlayerInformationSelectText.Text = ""
RealPlayersName = ""
UserIDBox.Text = ""
UserIDBoxSelectText.Text = ""
DetectedNamesUserID = 0
FadeOutAndDestroy(ModelHighlight)
end
end
end
end]]
--[[New]]
if Player and Character ~= nil then
local NewHighlight = CreateHighlight(Character)
FadeInHighlight(NewHighlight, 0.5)
local NewHighlight = Character:FindFirstChildOfClass("Highlight")
PlayerInformationSelectText.Visible = true
NewHighlight.Adornee = Character
PlayerInformationSelectText.Position = UDim2.fromOffset(MouseLocation.X, MouseLocation.Y)
PlayerInformationSelectText.Text = string.format(FormatText, Player.DisplayName, Player.UserId)
if Thread == nil then
Thread = task.spawn(FadeInHighlight)
end
--[[elseif Character ~= nil then
for _, ModelHighlight in pairs(Character:GetChildren()) do
if ModelHighlight:IsA("Highlight") then
PlayerInformationSelectText.Visible = false
ModelHighlight.Adornee = nil
if Thread then
task.cancel(Thread)
Thread = nil
ModelHighlight.OutlineTransparency = 1
end
end
end]]
end
for _, Model in pairs(game.Workspace:GetDescendants()) do
if Model:IsA("Model") and Model.Name ~= Target.Parent.Name or (Head.CFrame.Position - Camera.CFrame.Position).Magnitude > CameraMagnitude then
for _, ModelHighlight in pairs(Model:GetChildren()) do
if ModelHighlight:IsA("Highlight") then
print("Check - B [Deleted]")
ItemTooltip.Text = ""
PlayerInformation.Text = ""
PlayerInformationSelectText.Text = ""
RealPlayersName = ""
UserIDBox.Text = ""
UserIDBoxSelectText.Text = ""
DetectedNamesUserID = 0
FadeOutAndDestroy(ModelHighlight)
end
end
end
end
end
end
UserInputService.InputBegan:Connect(function(Input)
if Input.KeyCode == Enum.KeyCode.LeftControl then
UserInputService.InputBegan:Connect(function(InputedObject)
if InputedObject.UserInputType == Enum.UserInputType.MouseButton2 then
if Mouse.Target and Mouse.Target.Parent:IsA("Model") and Mouse.Target.Parent.Parent == game.Workspace then
PlayerInformation.Text = ""
PlayerInformationSelectText.Text = ""
UserIDBox.Text = "@"..RealPlayersName.." ("..DetectedNamesUserID..")"
UserIDBoxSelectText.Text = "Cmd/Ctrl + C to Copy User ID"
UserIDBox:CaptureFocus()
UserIDBox.SelectionStart = 0
UserIDBox.CursorPosition = #UserIDBox.Text + 1
UserIDBox.Visible = true
end
end
end)
end
end)
UserInputService.InputBegan:Connect(function(Input)
if Input.KeyCode == Enum.KeyCode.LeftControl then
UserInputService.InputBegan:Connect(function(InputedObject)
if InputedObject.KeyCode == Enum.KeyCode.C and UserIDBoxSelectText.Text == "Cmd/Ctrl + C to Copy User ID" then
UserIDBoxSelectText.Text = "Copied player information"
wait(2)
if UserIDBoxSelectText.Text ~= "Cmd/Ctrl + C to Copy User ID" then
UserIDBox.Visible = false
UserIDBox.Text = ""
UserIDBoxSelectText.Text = ""
PlayerInformation.Text = "@"..RealPlayersName.." ("..DetectedNamesUserID..")"
PlayerInformationSelectText.Text = "Cmd/Ctrl + Right Click to Select"
end
end
end)
end
end)
RunService.RenderStepped:Connect(UpdateTooltip)
So I ended up just using my old FadeInHighlight function because I would run into issues using yours and so I just changed it back.
Then added the SetRaycastParams function and etc.
Added 1 new line in the UpdateTooltip function and the reset is at the bottom.
I found out that when I brought back my old code for the unhovering part and removing the highlight/text stuff, that when I move to the Characters Hair, it just thinks I’m not on the character anymore and unselects it. Here’s a gif of what I mean.
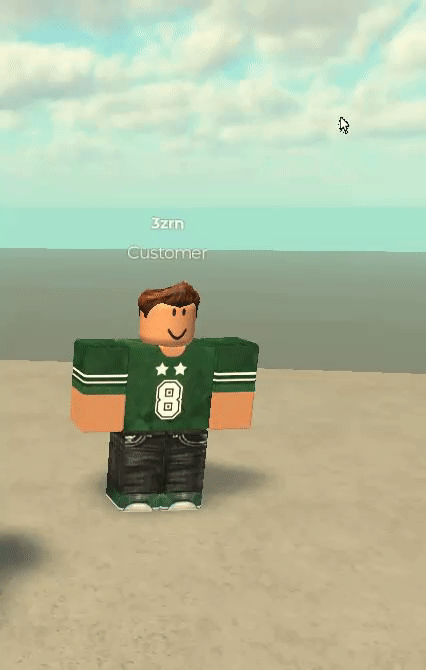
If anyone has any ideas on how to help that would be greatly appreciated, and thank you so much JohhnyLegoKing and others for all you’ve done on this thread.