I’m trying to make it so that the material of the parts in an object turn to Bright red and have a ForceField material during the transparency tween, and then turn back to the original material and color the part had once the transparency tween is finished.
Without the wait(2.25), the transparency tween works, but the material and color do not change, they stay the original color and material.
When the wait(2.25) is introduced right before changing “v” back to the original material and transparency, all of the parts are loaded in with a transparency of 0, and each individual part is tweened at a time.
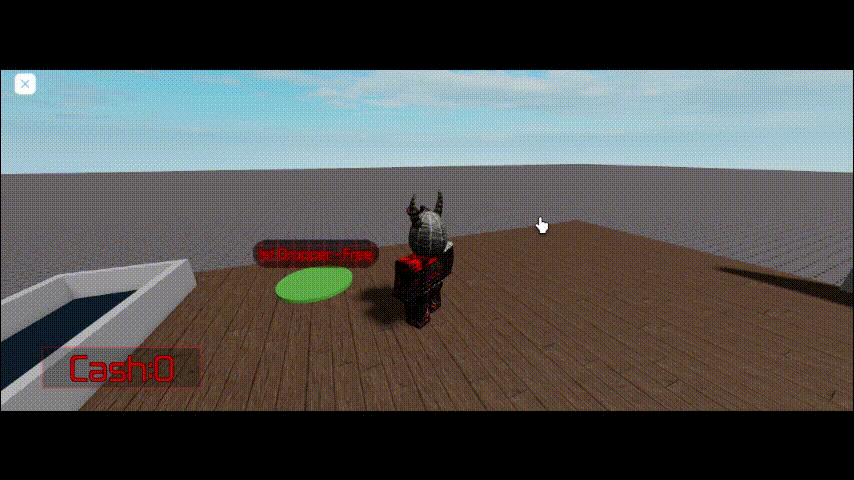
local function TweenVisible(Model, Time)
local VisibleInfo = TweenInfo.new(Time, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
local Tweens = {}
for _, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
local OriginalColor = v.BrickColor
local OriginalMaterial = v.Material
v.Material = Enum.Material.ForceField
v.BrickColor = BrickColor.new("Bright red")
v.Transparency = 1
local Tween = TweenService:Create(v, VisibleInfo, {Transparency = 0})
Tweens[v] = Tween
Tween:Play()
wait (2.25)
v.Material = OriginalMaterial
v.BrickColor = OriginalColor
end
end
return(Tweens)
end
This is the portion of the script in which the function is called later on.
if v:FindFirstChild("Price") and Player:WaitForChild("leaderstats").Cash.Value >= v.Price.Value then
if not Debounce then
Debounce = true
Player.leaderstats.Cash.Value -= v.Price.Value
Items[v.Item.Value].Parent = BoughtItems
v.ButtonPart.Building:Play()
local time = 2.25 --Time for tween to take
local ButtonTween = TweenService:Create(v.ButtonPart, TweenInfo.new(time), {Transparency = 1})
local Tweens = TweenVisible(BoughtItems[v.Item.Value], time)
ButtonTween:Play()
wait(2.25)
v:Destroy()
Debounce = false
end
end
1 Like
Your issue is that your waiting inside the for loop, meaning that each tween will be waited on individually.
local function TweenVisible(Model, Time)
local VisibleInfo = TweenInfo.new(Time, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
local Tweens = {}
for _, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
v:SetAttribute("OriginalColor", v.BrickColor)
v:SetAttribute("OriginalMaterial ", v.Material)
v.Material = Enum.Material.ForceField
v.BrickColor = BrickColor.new("Bright red")
v.Transparency = 1
local Tween = TweenService:Create(v, VisibleInfo, {Transparency = 0})
Tweens[v] = Tween
Tween:Play()
end
end
wait(2.25)
for _, v in pairs(Model:GetDescendants()) do
v.Material = v.GetAttribute("OriginalMaterial")
v.BrickColor = v.GetAttribute("OriginalColor")
end
return(Tweens)
end
1 Like
I get an error stating that Material is not a supported attribute type.
I edited the function to the following, and it allowed for the color part to work. Perhaps there is another way I have to tell the script what material is?
local function TweenVisible(Model, Time)
local VisibleInfo = TweenInfo.new(Time, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
local Tweens = {}
for _, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
v:SetAttribute("OriginalColor", v.BrickColor)
--v:SetAttribute("OriginalMaterial", v.Material)
--v.Material = Enum.Material.ForceField
v.BrickColor = BrickColor.new("Bright red")
v.Transparency = 1
local Tween = TweenService:Create(v, VisibleInfo, {Transparency = 0})
Tweens[v] = Tween
Tween:Play()
end
end
wait(2.25)
for _, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
--v.Material = v:GetAttribute("OriginalMaterial")
v.BrickColor = v:GetAttribute("OriginalColor")
end
end
return(Tweens)
end
My bad, I forgot attributes don’t support things like enums.
local function TweenVisible(Model, Time)
local VisibleInfo = TweenInfo.new(Time, Enum.EasingStyle.Linear, Enum.EasingDirection.InOut, 0, false, 0)
local Tweens = {}
local Materials = {}
for i, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
v:SetAttribute("OriginalColor", v.BrickColor)
Materials[i] = v.Material
v.Material = Enum.Material.ForceField
v.BrickColor = BrickColor.new("Bright red")
v.Transparency = 1
local Tween = TweenService:Create(v, VisibleInfo, {Transparency = 0})
Tweens[v] = Tween
Tween:Play()
end
end
wait(2.25)
for i, v in pairs(Model:GetDescendants()) do
if v:IsA("BasePart") then
v.Material = Materials[i]
v.BrickColor = v:GetAttribute("OriginalColor")
end
end
return(Tweens)
end
1 Like
Thank you for your help, I’ll have to look up info on attributes, this is my first time seeing one in a script, somehow.
1 Like
Glad to help! Here’s the developer article post about attributes:
1 Like