Hi there!
I am not sure how this could be done with the kind of shape you have in your image but I recently made a procedural room generation system with props and here’s how I handled the spawning of props:
(I highly recommend reading the comments)
CanPlaceProp function: (should be above the main function)
-- Function to check if a prop can be placed at a given position
function CanPlaceProp(position, room, prop)
local ray = Ray.new(position, Vector3.new(0, 1, 0))
local ignoreList = {prop}
local hitPart, hitPosition, hitNormal = workspace:FindPartOnRayWithIgnoreList(ray, ignoreList)
-- Check if the ray hit something and it's not the PropBlock
-- will explain this ^^^^^ at the end
return hitPart == nil or (hitPart.Name ~= "PropBlock" and hitPart ~= prop)
end
Main part spawning function:
local propInstance = prop:Clone() -- change "prop" to what part you want to clone, should be in ReplicatedStorage
-- Attempt to place the prop in a suitable position
local attempts = 0
local maxAttempts = 15 -- you should probably make this number higher for your situation
local propZones = room.PropZones:GetChildren() -- This is a folder and should contain parts that are the shape of the thing you want to place your parts on, and it should be 0.1 studs thick (see image nr. 1)
repeat
local NewRandom = Random.new()
local propZone = propZones[NewRandom:NextInteger(1, #propZones)]
local randomPosition = Vector3.new(
NewRandom:NextNumber(-propZone.Size.X/2, propZone.Size.X/2) + propZone.Position.X,
NewRandom:NextNumber(-propZone.Size.Y/2, propZone.Size.Y/2) + propZone.Position.Y,
NewRandom:NextNumber(-propZone.Size.Z/2, propZone.Size.Z/2) + propZone.Position.Z
)
propInstance:SetPrimaryPartCFrame(CFrame.new(randomPosition)) -- your part should be inside a model, as well as have a small part (0.1 stud cube) at the bottom for its origin (see image nr. 2)
attempts = attempts + 1
until CanPlaceProp(propInstance.PrimaryPart.Position, room, propInstance) or attempts >= maxAttempts
-- Check if a suitable position was found
if attempts < maxAttempts then
propInstance.Parent = room.Propsplacement -- Change this to the folder you want the part to be in
else
warn("Unable to find a suitable position for prop: ", PropToGenerate)
propInstance:Destroy()
end
Images and a bit of explaining:
image nr. 1
With the shape of your platform, I recommend making a propZone the size of those platforms (or a propZone for each platform because you can use multiple, just put them in a folder) and then using PropBlock parts to block out the air and not let the parts spawn outside of the platforms (also put them in a folder, you can use as many as you want)
image nr. 2 (had to use imgur because it wasn’t letting me post it here for some reason, so I hope that the image loads correctly)
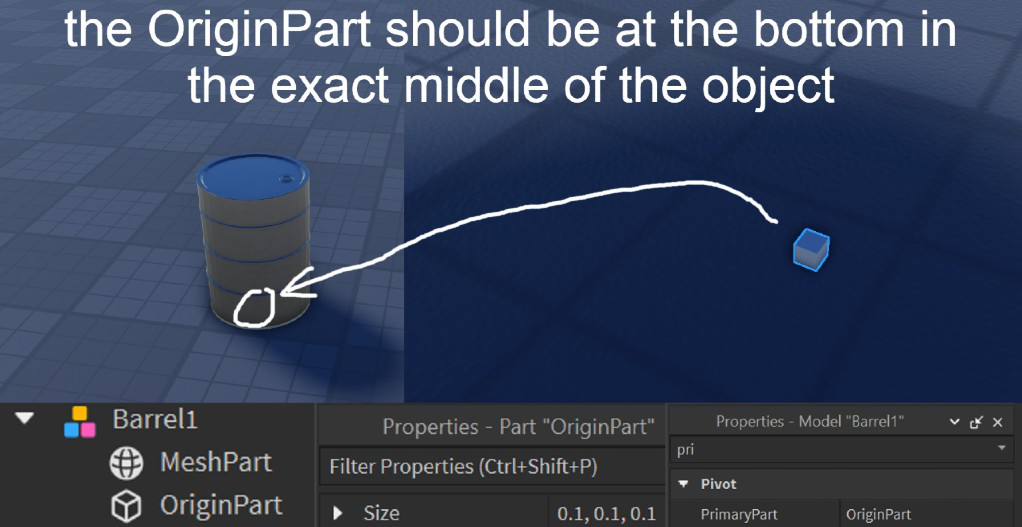
Let’s say you want random barrels to be placed, this is how you would set that up ^
I hope that all of this makes sense and that you can implement this into your script!